Error Handling Best Practices in Python
Writing error-free code is near to impossibility. Even experienced developers create bugs and face unexpected scenarios while coding. That’s where Error Handling comes into play. In Python, error handling is done through the use of exceptions that are caught in try
blocks and handled in except
blocks. If an error is encountered, a ‘try’ block code execution is stopped and transferred down to the ‘except’ block. In addition to using an except block after the ‘try’ block, ‘else’ and ‘finally’ keywords can be useful.
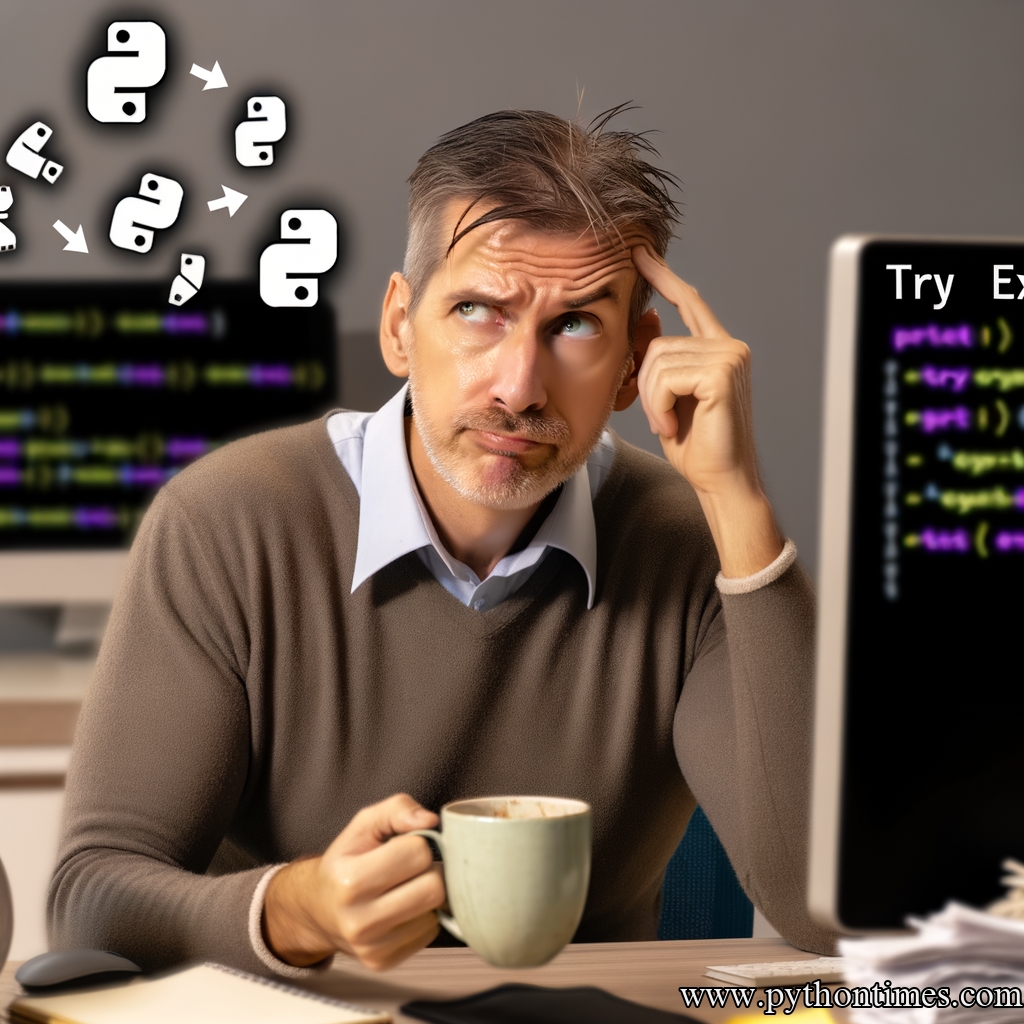
This article will present some best practices in Python Error Handling. We’ll go over the following points:
- Basic Exception Handling
- Catching Multiple Exceptions
- Using Else and Finally Clauses
- Custom Exceptions
- Assertions in Python
- Using Logging for Error Diagnostic
- Do Not Suppress All Exceptions
- Clean Up Resources Properly
- Difference Between Syntax Errors and Exceptions
1. Basic Exception Handling:
The basic syntax for catching exceptions in Python is:
try:
# some code here
except SomeException:
# some code here
In the above example, the code within the try
block is executed. If Python encounters an exception of the type SomeException
, the control will immediately pass to the except
block, bypassing the rest of the try
block.
Consider an example:
try:
result = 5 / 0
except ZeroDivisionError:
print("Sorry, you can't divide by zero")
If you run the above code, Python will find that it cannot perform division by zero and raise a ZeroDivisionError
which we handle in the except
block.
2. Catching Multiple Exceptions:
A try
block can have multiple except
blocks to catch and handle multiple exceptions. The syntax looks something like this:
try:
# some code here
except FirstException:
# handle first exception
except SecondException:
# handle second exception
Here’s an example:
try:
text = input("Enter something: ")
if text == '1':
raise ValueError("ValueError occurred")
elif text == '2':
raise TypeError("TypeError occurred")
except ValueError as e:
print(e)
except TypeError as e:
print(e)
3. Using Else and Finally Clauses:
In Python, else
clause in the try/except
block can contain the code that must be executed if no error was raised. The finally
clause lets you execute code, regardless of the result of the try
and except
blocks.
try:
# some code here
except SomeException:
# handle exception
else:
# No exception, execute this code
finally:
# Always executed
4. Custom Exceptions:
In python, you can define your own exceptions by deriving a class from the built-in Exception class or any of its subclasses.
class MyException(Exception):
pass
Then use this custom exception in your code.
try:
raise MyException("This is an exception")
except MyException as e:
print(e)
5. Assertions in Python:
Assertions are used as debugging tools as they help in checking the validity of the code. They force the programmer to think about the logic of the code and at the same time, they signal Python to test particular logic.
assert condition, "Error message"
6. Using Logging for Error Diagnostic:
logger.exception comes handy when you are catching exceptions. It logs an ERROR message and stacks trace of the exception.import logging
try:
1 / 0
except ZeroDivisionError as e:
logging.exception("Exception occurred")
7. Do Not Suppress All Exceptions:
In Python, never use a blank except
clause in your Python programs.
try:
# some code here
except: # Don't do this!
pass
This will catch all exceptions and handle them by doing nothing. This is a bad programming practice as it will catch system exit interrupts and keyboard interrupts too.
8. Clean Up Resources Properly
One of the crucial best practices in relation to error handling revolves around resource cleanup. Whether or not exceptions occur, closing a file, releasing a lock, or other similar resource cleanup tasks must always be fulfilled.
try:
f = open('file.txt')
# perform file operations
finally:
f.close()
This ensures that f.close()
is always executed, irrespective of whether an exception occurred or not.
9. Difference Between Syntax Errors and Exceptions:
Syntax errors occur when the parser encounters a syntax error, these are perhaps the easiest to fix. While exceptions are raised when some internal event (like an operation that is not possible) occurs, which changes the normal flow of the program.
Through experience and practice, you can improve your skills in error handling which play a pivotal role to write robust, professional Python code. Remember, “Errors should never pass silently. Unless explicitly silenced” as Zen of Python suggests!
Happy Coding!