Authentication and Authorization in Django
Django, a mighty Python web framework, has built-in provisions for creating robust and secure web applications, including the fundamental security concepts of Authentication and Authorization. This article is intended for both beginners new to Django and seasoned Python enthusiasts who wish to refresh or strengthen their knowledge of Django’s sponge-like Auth system.
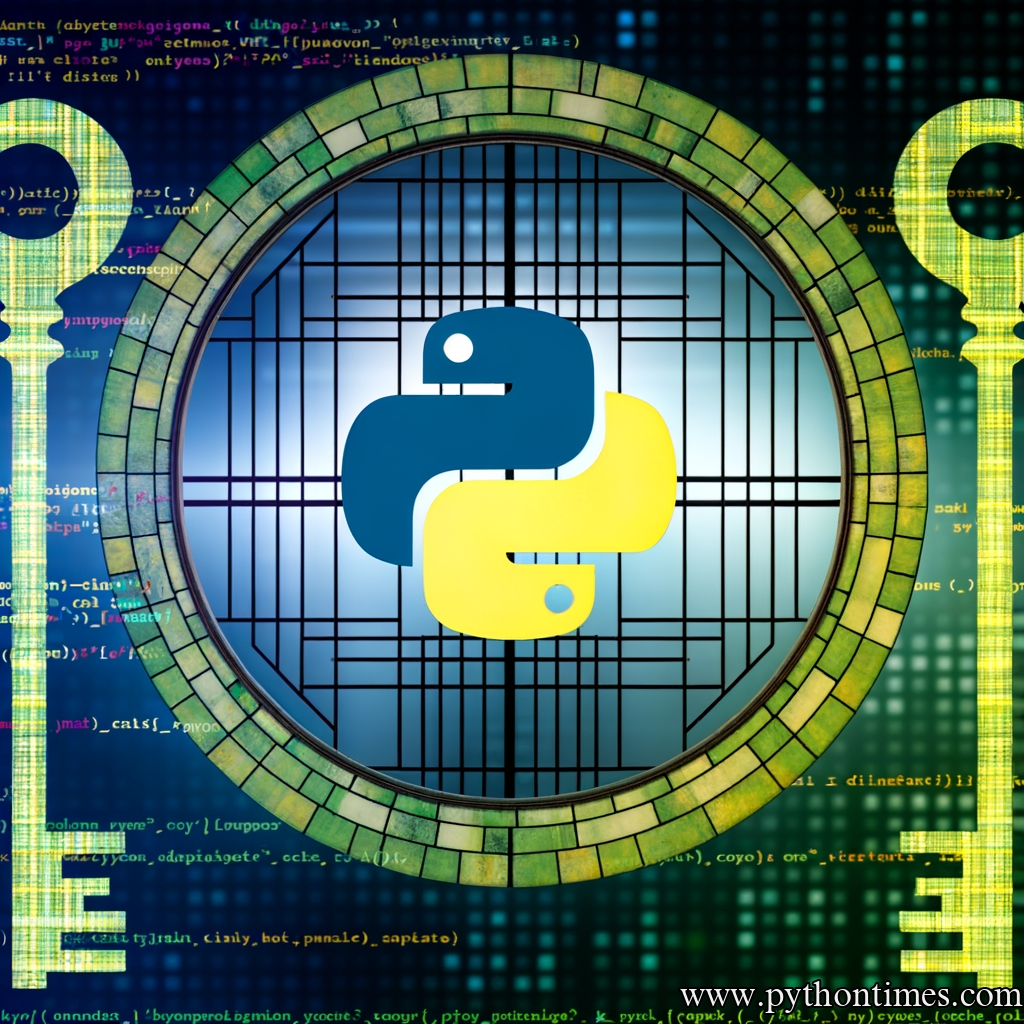
What is Authentication and Authorization in Django?
Authentication refers to the process of verifying the identity of a user, while authorization pertains to granting or denying permissions to a specific user to access certain features or pages in a website. Django provides a robust system for both authentication and authorization, collectively referred to as Django’s ‘Authentication’ system.
Authentication in Django
Django authentication provides capabilities for password hashing. It ships with a built-in User model for authentication, however, the flexibility of Django allows us to create our custom user model.
from django.contrib.auth.models import User
User = User.objects.create_user('john', 'lennon@thebeatles.com', 'johnpassword')
User.save()
This code creates a new user with a username, email, and password.
To verify a user’s credentials, Django provides an authenticate
function.
from django.contrib.auth import authenticate
user = authenticate(username='john', password='johnpassword')
if user is not None:
# A backend authenticated the credentials
print("User authenticated")
else:
# No backend authenticated the credentials
print("Authentication failed")
Authorization in Django
After authenticating users, Django utilizes Authorization to handle permissions and group management. Django’s authorization includes permission and group handling. ‘Permission’ in Django is the ability to perform a specific task, i.e., changing a model. ‘Group’ is a collection of users to apply the same permissions to multiple users.
from django.contrib.auth.models import Permission, User
from django.contrib.contenttypes.models import ContentType
content_type = ContentType.objects.get_for_model(User)
permission = Permission.objects.create(codename='can_view_users', name='Can view users', content_type=content_type)
user = User.objects.get(username='john')
user.user_permissions.add(permission)
The above code provides a user permission codenamed ‘can_view_users’.
Django’s Authentication Views
For developers prioritizing agility, Django also provides several built-in views in django.contrib.auth.views to handle common authentication tasks. These include login, logout, password reset, password reset done, password reset confirm, password reset complete, and password change.
Conclusion
Django’s built-in authentication and authorization system is a great start for any web application. It is secure, robust, scalable, and most importantly, customizable. It makes managing users, groups, and permissions exceedingly straightforward and elegant. Authentication and Authorization indeed form the backbone of any secure Django application.
FAQ
-
What does Authentication mean in Django? The process of confirming a user’s identity by verifying their provided credentials.
-
What does Authorization mean in Django? It refers to the process of granting a user certain permissions to perform tasks or access resources.
-
Can I create a custom user model in Django? Yes, Django allows creating a custom user model for more flexible authentication.
-
What is a Permission in Django? In Django, Permission is the ability of a user to perform a certain task, such as changing a model.
-
What is a Group in Django? A Group in Django is a collection of users having the same permissions.