Web Scraping with BeautifulSoup and Requests
Web scraping is a technique used to extract data from websites by parsing the HTML and XML content of a webpage. Python provides powerful libraries such as BeautifulSoup and Requests that make web scraping a breeze. In this article, we will explore how to use BeautifulSoup and Requests to scrape data from websites using Python. Whether you’re a beginner or an experienced Python enthusiast, this comprehensive guide will walk you through the essentials of web scraping. So, let’s dive in!
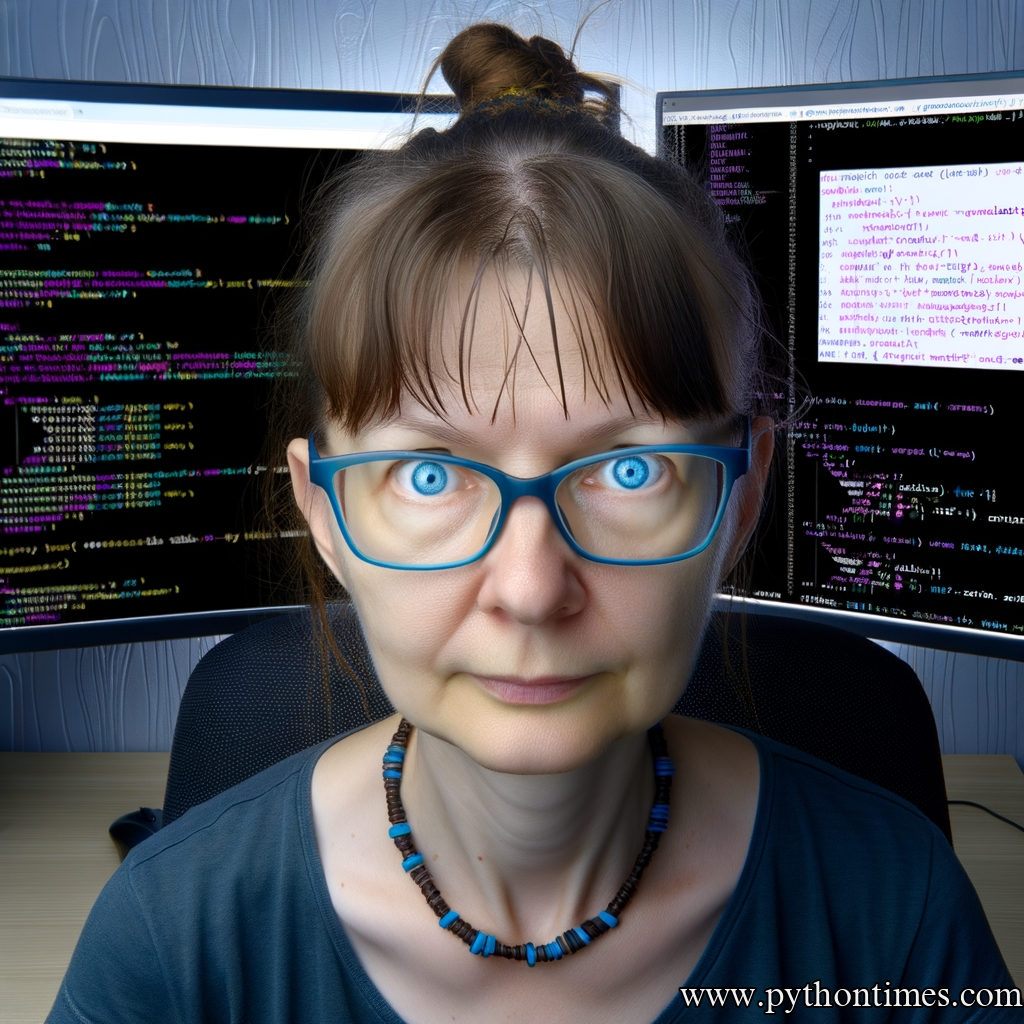
Table of Contents
- Introduction
- Installing BeautifulSoup and Requests
- Making HTTP Requests with Requests
- Navigating the HTML Tree with BeautifulSoup
- Scraping Data from a Single Webpage
- Handling Pagination
- Dealing with Forms
- Web Scraping Etiquette and Legal Considerations
- Conclusion
1. Introduction
Before we dive into the specifics of web scraping with BeautifulSoup and Requests, let’s start with a brief introduction to web scraping itself.
Web scraping is the process of extracting data from websites by fetching and parsing the underlying HTML and XML code. It allows us to automate the extraction of data, which can be particularly useful when dealing with large amounts of information or when the website does not provide an API for accessing the data.
With web scraping, we can extract information such as text, images, links, tables, and more from webpages. This data can then be stored for analysis, used for research, or integrated into other applications.
2. Installing BeautifulSoup and Requests
Before we can start web scraping, we need to install the necessary libraries. Open your terminal and run the following command to install BeautifulSoup and Requests:
pip install beautifulsoup4 requests
By executing this command, we install both BeautifulSoup and Requests libraries. BeautifulSoup helps us parse and navigate HTML and XML content, while Requests is a library for making HTTP requests in Python.
With both libraries installed, we are ready to start scraping!
3. Making HTTP Requests with Requests
Before we can scrape any website, we need to make an HTTP request to retrieve the webpage’s HTML content. This is where the Requests library comes into play.
To demonstrate how to make an HTTP request, let’s start by fetching the HTML content of a webpage:
import requests
url = "https://example.com"
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
print(html_content)
Here, we import the Requests library and specify the URL of the webpage we want to scrape. We then use the get
method from the Requests library to make the HTTP GET request.
If the response status code is 200, which indicates a successful request, we can access the HTML content of the webpage through the text
attribute of the response object. We print the HTML content to the console for demonstration purposes.
Now that we know how to make an HTTP request, let’s move on to parsing the HTML content with BeautifulSoup.
4. Navigating the HTML Tree with BeautifulSoup
To extract data from an HTML document, we need a way to navigate its structure. BeautifulSoup provides a simple and intuitive API for traversing and manipulating the HTML tree.
Let’s explore some key concepts and methods with an example. Consider the following HTML snippet:
<html>
<head>
<title>Web Scraping with BeautifulSoup and Requests</title>
</head>
<body>
<h1>Welcome to PythonTimes!</h1>
<div class="article">
<h2>Web Scraping</h2>
<p>Web scraping is a powerful technique...</p>
</div>
<div class="article">
<h2>Data Analysis</h2>
<p>Data analysis is a crucial step...</p>
</div>
</body>
</html>
We can use BeautifulSoup to navigate this HTML tree and extract relevant information. Let’s start by loading the HTML content into a BeautifulSoup object:
from bs4 import BeautifulSoup
html = '''
<html>
...
</html>
'''
soup = BeautifulSoup(html, "html.parser")
Here, we import the BeautifulSoup library and pass the HTML content and the parser type (“html.parser”) into the BeautifulSoup object.
Now, we can use various methods provided by BeautifulSoup to traverse and extract data from the HTML tree. For example, to extract the title of the webpage, we can use the title
attribute of the BeautifulSoup object:
title = soup.title
print(title.text)
This will output: “Web Scraping with BeautifulSoup and Requests”.
Similarly, we can extract other elements by their tag name, class name, or other attributes. For instance, to extract the text of the first <h2>
element within a <div>
with class “article”, we can use the find
method:
div = soup.find("div", class_="article")
h2 = div.find("h2")
print(h2.text)
This will output: “Web Scraping”.
In addition to finding individual elements, we can also find multiple elements using methods like find_all
or by using CSS selectors.
With an understanding of how to navigate the HTML tree, let’s move on to scraping data from a single webpage.
5. Scraping Data from a Single Webpage
Many times, we want to extract specific information from a webpage, such as article titles, prices, or contact details. BeautifulSoup makes it easy to scrape such data by leveraging its tree traversal capabilities.
Let’s consider an example where we want to scrape the titles and prices of products from an online shopping website.
import requests
from bs4 import BeautifulSoup
url = "https://example.com/products"
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.content, "html.parser")
products = soup.find_all("div", class_="product")
for product in products:
title = product.find("h2").text
price = product.find("span", class_="price").text
print("Title:", title)
print("Price:", price)
print()
In this example, we make an HTTP GET request to the URL of the webpage that lists the products we want to scrape. We then create a BeautifulSoup object using the HTML content of the response.
Next, we use the find_all
method to find all <div>
elements with the class “product”. For each product, we extract the title and price by navigating the HTML tree using methods like find
or by accessing element attributes directly.
Finally, we print the title and price of each product.
This example demonstrates how to scrape data from a single webpage. However, in many cases, the data we want might be spread across multiple pages. Let’s explore how to handle pagination next.
6. Handling Pagination
Pagination is a common pattern in websites where data is divided into multiple pages. To scrape data from multiple pages, we need to handle pagination effectively.
Let’s consider an example where we want to scrape the titles and authors of articles from a blog. The articles are spread across multiple pages, with each page having a link to the next page.
import requests
from bs4 import BeautifulSoup
url = "https://example.com/articles"
page = 1
has_next_page = True
while has_next_page:
page_url = f"{url}?page={page}"
response = requests.get(page_url)
if response.status_code == 200:
soup = BeautifulSoup(response.content, "html.parser")
articles = soup.find_all("div", class_="article")
for article in articles:
title = article.find("h2").text
author = article.find("p", class_="author").text
print("Title:", title)
print("Author:", author)
print()
next_link = soup.find("a", class_="next")
has_next_page = next_link is not None
if has_next_page:
page += 1
In this example, we start by setting the initial page number to 1 and a variable has_next_page
to True, as we assume there is a next page initially.
Inside the while loop, we construct the URL for each page by appending the page number as a query parameter. We then make an HTTP GET request to the page URL, create a BeautifulSoup object, and extract the relevant information.
After processing each page, we check if there is a link with the class “next” to indicate the presence of a next page. If a next page exists, we update the page number and continue the loop.
This allows us to scrape data from multiple pages. However, sometimes the website may require us to interact with forms to access the desired data. Let’s explore how to deal with forms in web scraping.
7. Dealing with Forms
In some cases, the data we want to scrape may be hidden behind forms or require us to submit some input. Fortunately, BeautifulSoup and Requests provide everything we need to interact with forms and submit data programmatically.
Let’s consider an example where we want to scrape data from a search results page of a website. We need to submit a search query and extract the titles and descriptions of the search results.
import requests
from bs4 import BeautifulSoup
url = "https://example.com/search"
search_query = input("Enter your search query: ")
data = {
"query": search_query,
}
response = requests.post(url, data=data)
if response.status_code == 200:
soup = BeautifulSoup(response.content, "html.parser")
results = soup.find_all("div", class_="result")
for result in results:
title = result.find("h2").text
description = result.find("p", class_="description").text
print("Title:", title)
print("Description:", description)
print()
In this example, we prompt the user to enter a search query using the input
function, and assign it to the search_query
variable.
We then create a dictionary data
with the search query as its value. This dictionary will be passed as data when making the HTTP POST request.
After making the request, we proceed to extract and print the titles and descriptions of the search results.
By including the required inputs in the data
dictionary, we can effectively interact with forms and scrape data that would otherwise be hidden behind them.
8. Web Scraping Etiquette and Legal Considerations
While web scraping can be a powerful technique, it is important to scrape websites responsibly and ethically. Here are some guidelines to follow:
- Respect the website’s terms of service: Make sure to read and understand the terms of service for the website you are scraping. Some websites may explicitly prohibit scraping or impose certain restrictions.
- Use reasonable resource consumption: Avoid placing unnecessary burden on the website’s servers by implementing appropriate delays between requests and avoiding excessive concurrent requests.
- Respect robots.txt: The robots.txt file is a mechanism used by websites to communicate which parts of the website should not be crawled. Make sure to respect the directives in the robots.txt file.
- Identify yourself: Include a User-Agent header in your HTTP requests to identify your scraping script. This can help website administrators identify and contact you if necessary.
- Do not overwhelm or disrupt the website: Avoid scraping websites at a high frequency or in a way that disrupts the normal operation of the website. Be mindful of bandwidth usage and processing resources.
Following these guidelines ensures that web scraping is done responsibly and helps maintain a positive relationship between scrapers and website owners.
9. Conclusion
Web scraping with BeautifulSoup and Requests allows us to automate the extraction of data from websites using Python. In this article, we covered the essentials of web scraping, from making HTTP requests to parsing and navigating HTML content.
We explored how to scrape data from a single webpage, as well as how to handle pagination and interact with forms. Additionally, we discussed web scraping etiquette and legal considerations to ensure responsible scraping practices.
With BeautifulSoup and Requests, you can extract valuable data from websites and leverage it for various purposes. Whether you’re a beginner or an experienced Python enthusiast, web scraping opens up a world of possibilities for automating data extraction and analysis.
So, start exploring the web, gather meaningful data, and unlock new insights with BeautifulSoup and Requests! Happy scraping!
References
- Requests library documentation: https://docs.python-requests.org
- BeautifulSoup documentation: https://www.crummy.com/software/BeautifulSoup/
About the Author
[Your Name]
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Maecenas lobortis urna in erat varius, vel vulputate ex mollis. Vivamus vel lorem congue, consectetur orci id, iaculis sem. Quisque dapibus erat a felis pulvinar, non mollis libero consequat. Integer vulputate ipsum sit amet libero eleifend vulputate. Phasellus eu massa quis orci tristique rutrum. Mauris nec neque dolor. Morbi in velit id lectus mollis lobortis vitae ac magna. Integer rutrum, libero ac gravida efficitur, mauris tortor feugiat tortor, id placerat sem turpis et eros. Quisque auctor eleifend nibh suscipit iaculis. Vestibulum nec leo risus. Donec id scelerisque ipsum. Aliquam fringilla lacus sapien, ut mattis ante tempus et.
Contact:
- Email: your-email@example.com
- Twitter: @your_twitter_handle
- LinkedIn: Your LinkedIn Profile