Understanding Django Templates and Template Tags
Django, a high-level web framework for Python, is designed to meet the fast-paced and tight deadlines of newsroom-like environments. It was meant to provide components to help handle common Web development tasks. Django focuses on automating as much as possible and adhering to the DRY principle – Don’t Repeat Yourself.
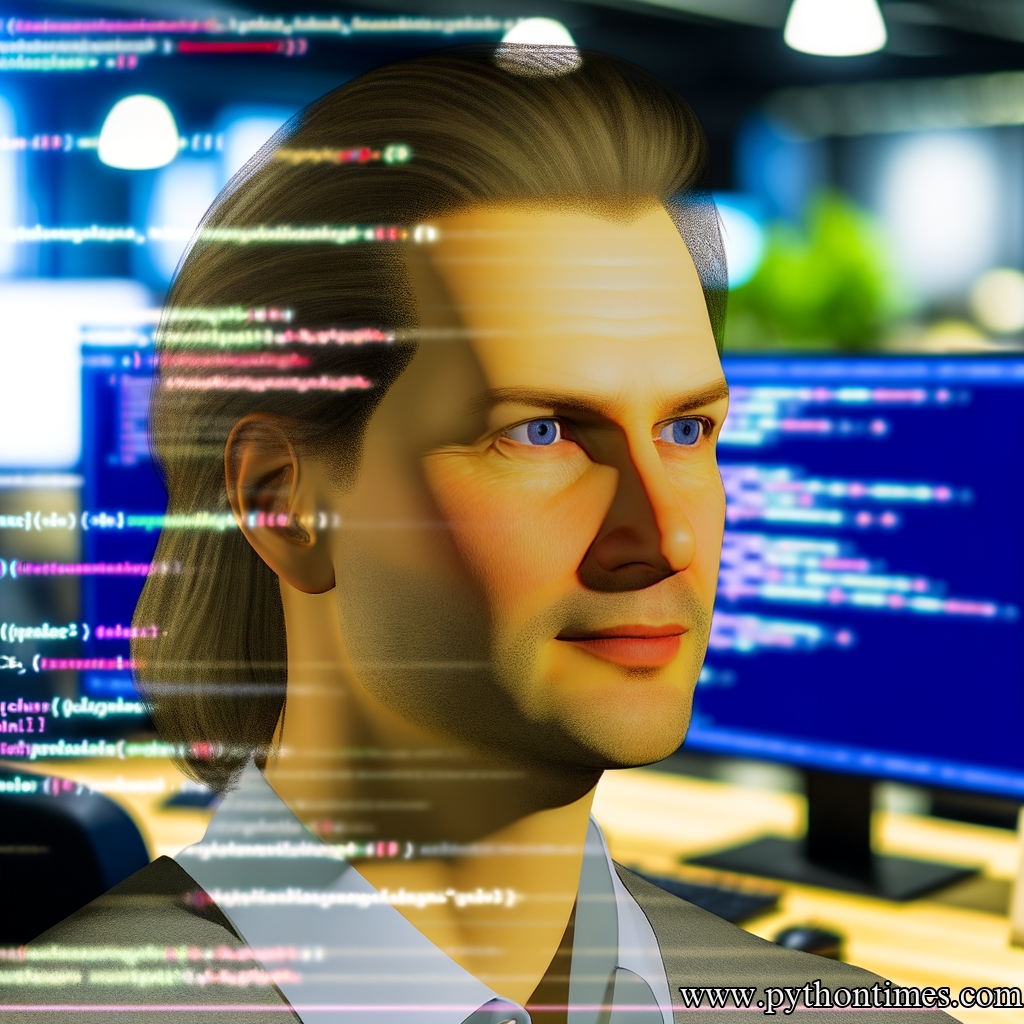
In this guide, we’ll explore one significant feature of Django – Templates and Template Tags. We’ll break down the concepts into digestible explanations and provide practical examples for both beginner and experienced developers. Let’s get started!
Django Templates: An Overview
In Django, a template is a simple text file that defines the structure or layout of a file (HTML, XML, CSV, etc.). It can contain variables, which get replaced with values when the template is evaluated, and tags, which control the logic of the template. The engine introduces a domain-specific language (DSL) for this purpose.
Why Use Django Templates?
The main goal of using templates is to separate the presentation of a document from its data. Some reasons why Django templates are used include:
-
The template system is designer-friendly. Designers can edit templates without having to know Python.
-
The templates are decoupled from the rest of the application. This allows you to change your interfaces without revising the underlying code.
-
The template system is extensible. New template tags and filters can be introduced as needed.
Understanding Template Tags
Django templates are populated with data using variables and tags. Variables are surrounded by curly braces {{ }}
, while tags are surrounded by {% %}
.
-
Variables: These are simple placeholders for data. When the template engine encounters a variable, it evaluates it and replaces it with the result.
-
Tags: Tags are more complex. They can output content, serve as control structures (e.g., an “if” statement or a “for” loop), grab content from a database, or enable or disable auto-escaping.
Basic Template Tags
Django’s template system comes with a wide array of built-in tags and filters. Here are some basic tags:
-
{% for %} and
{% endfor %}
: Creates a loop over a list. -
{% if %},
{% else %}
, and{% endif %}
: Encloses a conditional expression. -
{% block %} and
{% endblock %}
: Defines an area that is replaceable and can be filled with content when the template is used.
Let’s illustrate these concepts with some examples:
- Using Variables inside Templates:
<html>
<body>
<h1>Welcome, {{ user.name }}!</h1>
</body>
</html>
Here, {{ user.name }}
is a variable. When Django renders this template, it will replace the variable with the user’s actual name.
- Using Tags inside Templates:
<html>
<body>
{% if user.is_authenticated %}
<h1>Welcome, {{ user.name }}!</h1>
{% else %}
<h1>Welcome, Guest!</h1>
{% endif %}
</body>
</html>
In this code, {% if user.is_authenticated %}
and {% endif %}
are template tags that form an if...else
conditional structure.
Advanced Template Tags
To make use of advanced template tags, Django has to load them first using the {% load %}
tag. These tags offer extra functionalities such as looping over a list in reverse order, creating forms from context data, and more.
Here’s an example how to use the {% load %}
tag:
{% load i18n %}
<html>
<body>
<h1>{% trans "Welcome, " %}{{ user.name }}!</h1>
</body>
</html>
In this example, {% load i18n %}
loads the internationalization tags library. It uses the {% trans %}
tag to mark a string for translation.
Built-in template tags and filters are just the beginning. You can create your own tags and filters, and Django can use them just like the built-in ones. This provides unlimited potential for text processing and manipulation in your templates.
Template Inheritance
Django’s template architecture provides an inheritance system, enabling an easy way to manage repetitive layout elements such as headers, footers, and navigation menus. With template inheritance, you define these elements in a base template and then extend the base template in child templates.
A child template can also override blocks of content from its parent template using the {% block %}
tag:
{% extends "base_generic.html" %}
{% block content %}
<h1>Our Amazing Products</h1>
<p>Here are a few of our most popular products:</p>
{% for product in product_list %}
<p>{{ product.name }} - ${{ product.price }}</p>
{% endfor %}
{% endblock %}
In this example, the {% extends %}
tag tells Django that this template “extends” the base_generic.html
template. The {% block content %}
tag defines a block that child templates can fill.
FAQs
Q: Where are Django templates stored?
A: Django templates are typically stored in a templates
directory inside your Django application.
Q: How do I load a Django template?
A: To load a Django template, you use the render()
function in your view.
Q: Can I use JavaScript inside Django templates?
A: Yes, you can include JavaScript code inside Django templates like a standard HTML file.
Q: Can I include one template inside another?
A: Yes, with the {% include %}
tag, you can include the contents of one template inside another.
Q: What happens if a variable inside a Django template doesn’t exist?
A: Django templates fail silently when they encounter a variable that does not exist. Django will insert the value of settings.TEMPLATE_STRING_IF_INVALID
(Default: ”) when it encounters an invalid variable.
Conclusion
Understanding Django templates and template tags is key to building powerful, interactive websites with Django. We’ve covered the basic and advanced use of Django templates, template tags and variables, and even how to use the powerful concept of template inheritance. Remember, this is just a foundation – Django’s template system provides a host of more advanced capabilities, which you can explore as you become more comfortable with these basics.