The Power of Python Generators: Leveraging Lazy Evaluation
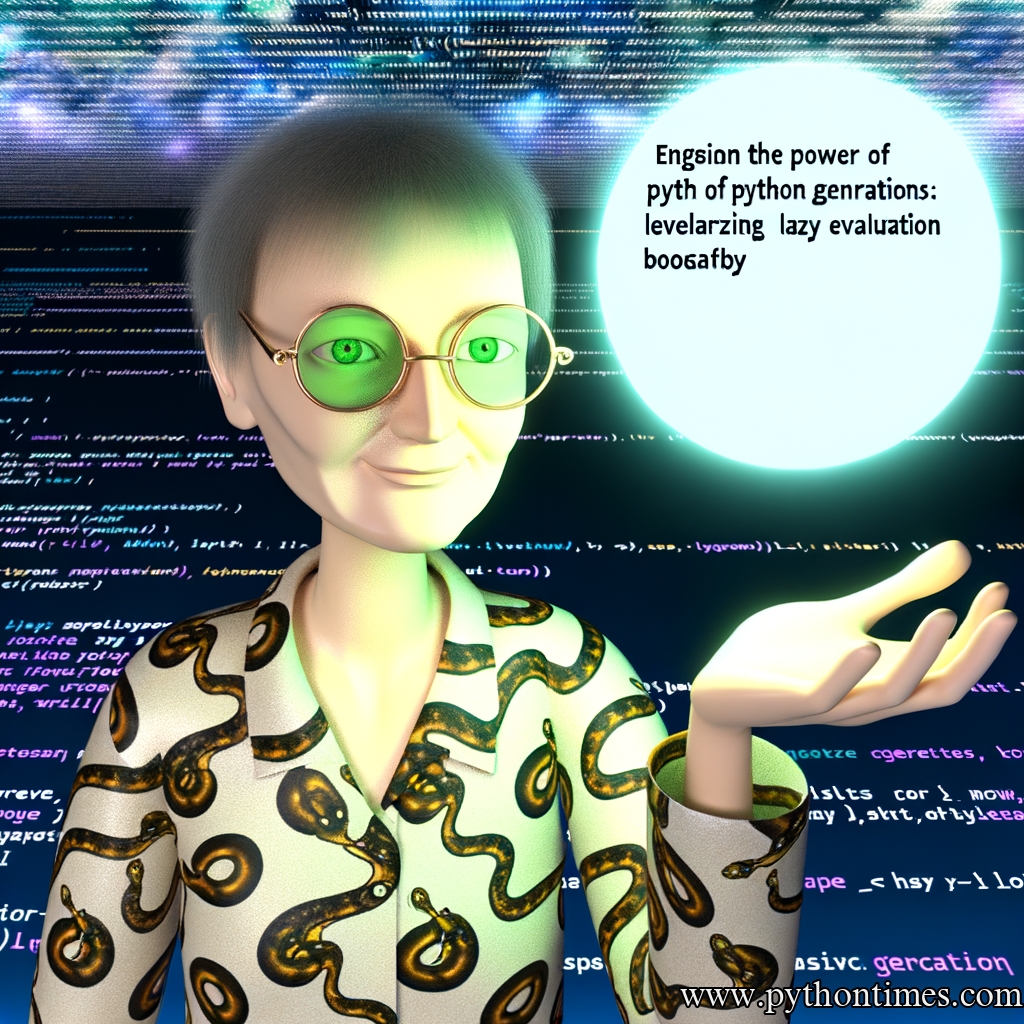
Python is renowned for its flexibility, ease of use, and extensive libraries. One of its most powerful features is its support for generators, which enable lazy evaluation. Lazy evaluation allows programmers to optimize memory usage and improve overall efficiency by only generating values as needed. In this article, we will explore the power of Python generators and how to leverage lazy evaluation to enhance your code.
Understanding Generators
At its core, a generator is a specialized type of iterator. It generates values one by one, on the fly, using the yield
statement. Unlike traditional iterators, which store all values in memory, generators compute and yield values as requested. This approach saves memory and allows for efficient computation of large or infinite sequences.
Consider the following example:
def fibonacci_generator():
a, b = 0, 1
while True:
yield a
a, b = b, a + b
The fibonacci_generator
function creates an infinite sequence of Fibonacci numbers. By utilizing the yield
statement, we can generate each Fibonacci number on demand. This is possible because generators maintain their state between successive calls, allowing them to remember their position within the sequence.
The Benefits of Lazy Evaluation
With generators, you only generate values as needed, which offers several benefits:
1. Reduced Memory Usage
Generating values on the fly allows you to work with large or infinite sequences without storing them entirely in memory. By avoiding the need to pre-calculate or load an entire sequence, you can significantly reduce memory consumption. This is especially valuable when dealing with algorithms that generate or manipulate large datasets.
2. Improved Efficiency
Lazy evaluation enables more efficient computation by only generating values when necessary. This can result in faster code execution, as unnecessary calculations are avoided. By generating values incrementally, you can reduce the overhead associated with upfront calculations.
3. Enhanced Readability
Generators enable a more intuitive and readable coding style. By expressing computations as a sequence of generator calls, you can make complex algorithms more concise and easier to understand. This can improve maintainability and make your code more accessible to other developers.
4. Infinite Sequences
With generators, you can easily work with infinite sequences. Since values are generated on demand, you can handle sequences that would otherwise be impractical or impossible to compute in their entirety. This is particularly useful in scenarios such as stream processing or simulations, where you might not know the size of the desired sequence in advance.
Practical Examples
To truly grasp the power of generators, let’s explore some practical examples that demonstrate their versatility and efficiency.
Example 1: Prime Number Generator
Generating prime numbers can be a computationally expensive task, especially as the numbers get larger. By utilizing a generator, we can lazily compute prime numbers one at a time, avoiding unnecessary calculations.
def prime_generator():
primes = []
current = 2
while True:
if all(current % prime != 0 for prime in primes):
yield current
primes.append(current)
current += 1
In this example, the prime_generator
function generates prime numbers by iteratively checking if a number is divisible by any previously generated prime numbers. By utilizing lazy evaluation, we only perform the necessary calculations, resulting in more efficient code execution.
Example 2: Parsing Large Files
Reading and parsing large files can quickly become memory-intensive. By utilizing generators, we can process files line by line, avoiding the need to hold the entire file in memory.
def parse_large_file(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line.strip()
The parse_large_file
function reads a file line by line and yields each line as it is processed. This approach allows you to handle files of any size without worrying about memory limitations. Additionally, you can incorporate additional processing steps within the generator function to transform the data as needed.
Tips for Leveraging Generators
Now that we’ve explored the power of generators, let’s dive into some tips and best practices for leveraging them effectively:
1. Understanding Generator Expressions
In addition to defining generators as functions, Python also supports generator expressions. Similar to list comprehensions, generator expressions provide a concise syntax for defining generators.
# Example 1: Generator Expression for Squares
squares = (x ** 2 for x in range(10))
# Example 2: Filtering Using a Generator Expression
even_numbers = (x for x in range(10) if x % 2 == 0)
Generator expressions are especially useful when you need to generate values on the fly without the need for a separate generator function.
2. Chaining Generators
Generators can be chained together to perform complex computations while preserving memory efficiency. By utilizing generator functions or expressions in conjunction with the yield from
statement, you can create powerful generator pipelines.
def transform_values(generator):
for value in generator:
yield perform_transformation(value)
def filter_values(generator):
for value in generator:
if meets_condition(value):
yield value
def process_data(data):
filtered_data = filter_values(data)
transformed_data = transform_values(filtered_data)
yield from transformed_data
In this example, the process_data
generator pipeline takes in a data source (data
) and applies successive transformations and filtering steps. By chaining generators together, you can achieve complex data processing pipelines while maintaining the benefits of lazy evaluation.
3. Utilizing Generator Caching
Generators can be cached to improve efficiency in certain scenarios. Python provides the itertools
module, which offers useful functions for combining and manipulating iterators and generators. The itertools
module includes the tee
function, which creates multiple independent copies of a generator.
from itertools import tee
def cached_generator(generator):
cached_copy, uncached_copy = tee(generator)
return list(cached_copy), uncached_copy
By caching a generator, you can iterate over its values multiple times without re-computing them. This can be particularly useful when working with complex computations or when the generator’s values are expensive to generate.
4. Managing Resource Cleanup
When working with generators that involve external resources, such as file or network operations, it’s essential to manage resource cleanup appropriately. Python provides the contextlib
module, which offers a convenient way to handle resource cleanup using the contextmanager
decorator.
from contextlib import contextmanager
@contextmanager
def open_file(file_path):
file = open(file_path, 'r')
try:
yield file
finally:
file.close()
In this example, the open_file
context manager ensures that the file is closed once the generator has completed its execution. This allows you to handle file I/O using generators while ensuring proper resource cleanup.
Real-World Applications
Generators have a wide range of applications across various domains. Let’s explore a few real-world scenarios where generators can provide significant benefits:
1. Data Streaming and Processing
Generators are invaluable when it comes to processing large amounts of data. They enable stream processing by reading data on-the-fly and transforming or filtering it as needed. This is particularly useful in scenarios such as log analysis or real-time data processing, where data streams need to be processed continuously.
2. Web Scraping and API Requests
When scraping websites or making API requests, it’s common to retrieve data in chunks or paginated form. Generators can simplify this process by lazily fetching and processing data. This approach enables more efficient memory usage and allows you to work with large datasets without overwhelming your computer’s resources.
3. Machine Learning and Data Science
Generators can be advantageous in machine learning and data science workflows. They allow you to lazily load and preprocess large datasets, reducing memory usage and improving training speeds. By adapting your data pipelines to utilize generators, you can efficiently train machine learning models on massive datasets.
Conclusion
Python generators offer a powerful approach to lazy evaluation, enabling more efficient code execution and reduced memory usage. By generating values on-demand, you can handle large or infinite sequences, process data efficiently, and optimize resource management. Whether you’re working on complex computations, processing large datasets, or building real-time applications, leveraging generators can help you unlock the full potential of your Python code.
In this article, we’ve explored the benefits of lazy evaluation, provided practical examples, and shared tips for utilizing generators effectively. By incorporating generators into your programming toolkit, you can write more efficient, readable, and scalable code.
So, what are you waiting for? Go forth and harness the power of Python generators to take your code to new heights!