Text Mining and Sentiment Analysis with Python
Python is a versatile programming language widely used for data analysis in fields such as natural language processing, artificial intelligence, and machine learning. Among its many applications, Python is extremely efficient for text mining and sentiment analysis. In this article, we will take a comprehensive look at how to perform text mining and sentiment analysis using Python.
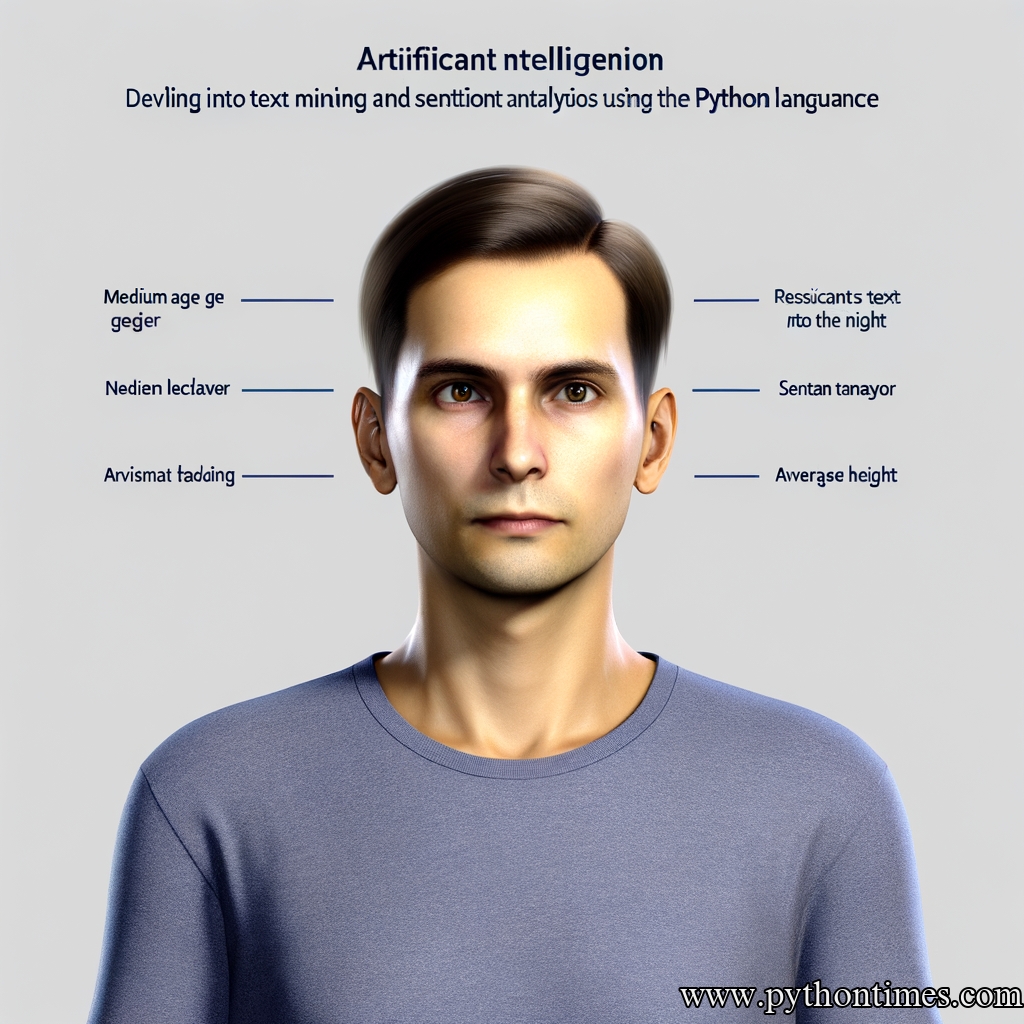
Table of Contents
- An Introduction to Text Mining
- An Introduction to Sentiment Analysis
- Python Libraries for Text Mining and Sentiment Analysis
- Text Mining with Python
- Sentiment Analysis with Python
- Practical Examples
- Conclusion
1. An Introduction to Text Mining
Text mining, also known as text data mining, essentially means unearthing valuable information from text. It is a method of extracting hidden insights from unstructured text data, which are then used for various purposes such as further processing, investigation, or analysis.
Text mining involves several sub-processes: information retrieval, data mining, information extraction, and knowledge discovery. These processes convert raw text data into a structured format.
2. An Introduction to Sentiment Analysis
Sentiment analysis, or opinion mining, refers to using natural language processing, text analysis, and computational linguistics to identify and extract subjective information from source materials. It measures the inclination of people’s opinions in relation to the events, topics, and individuals within the text.
Through sentiment analysis, you can identify sentiments such as positive, negative, or neutral present in the data.
3. Python Libraries for Text Mining and Sentiment Analysis
Python, with its rich ecosystem of libraries, makes text mining and sentiment analysis easy and efficient. Here are the key libraries:
-
NLTK (Natural Language Toolkit): A popular open-source library in Python used for natural language processing, which includes text mining and sentiment analysis.
-
Spacy: It is widely used for tasks associated with natural language understanding, including tokenization, part-of-speech tagging, named entity recognition, and more.
-
TextBlob: TextBlob provides a simple API for NLP tasks such as part-of-speech tagging, noun phrase extraction, and sentiment analysis.
-
Gensim: An open-source library for unsupervised topic modeling and natural language processing.
-
Scikit-learn: A machine learning library in Python built on scipy (Scientific Python), numpy, and matplotlib, it provides simple and efficient tools for data mining and data analysis.
4. Text Mining with Python
We’ll now explore how to perform text mining with Python. But first, you need to install the necessary libraries. If you haven’t already done so, you can use the following commands:
pip install nltk
pip install spacy
pip install gensim
pip install scikit-learn
4.1 Data Preprocessing
In text mining, data preprocessing is a crucial step that involves cleaning the raw text data to make it suitable for analysis. Here’s how you can perform data preprocessing with Python:
import nltk
import re
from nltk.corpus import stopwords
from nltk.stem.porter import PorterStemmer
nltk.download('stopwords')
def preprocess_text(text):
review = re.sub('[^a-zA-Z]', ' ', text)
review = review.lower()
review = review.split()
ps = PorterStemmer()
review = [ps.stem(word) for word in review if not word in set(stopwords.words('english'))]
review = ' '.join(review)
return review
5. Sentiment Analysis with Python
While TextBlob is capable of performing many NLP tasks, for this guide, we will focus on using it for sentiment analysis.
from textblob import TextBlob
def analyze_sentiment(text):
analysis = TextBlob(text)
if analysis.sentiment.polarity > 0:
return 'Positive'
elif analysis.sentiment.polarity < 0:
return 'Negative'
else:
return 'Neutral'
6. Practical Examples
Having gained an understanding of the theories and Python libraries needed for text mining and sentiment analysis, let’s put these concepts into practice.
6.1 Practical Example of Text Mining
Suppose you have a dataset of customer reviews, and you need to mine this data to understand the common themes in these reviews.
import pandas as pd
from sklearn.feature_extraction.text import CountVectorizer
dataset = pd.read_csv('customer_reviews.csv')
processed_reviews = dataset['review'].apply(preprocess_text)
cv = CountVectorizer(max_features = 1500)
X = cv.fit_transform(processed_reviews).toarray()
6.2 Practical Example of Sentiment Analysis
Suppose you have tweets from Twitter users regarding a new product launch, and you want to determine public sentiment regarding this event.
tweets = pd.read_csv('new_product_tweets.csv')
tweets['sentiment'] = tweets['tweet'].apply(analyze_sentiment)
tweets.head()
7. Conclusion
Text mining and sentiment analysis are powerful tools in the current data-driven landscape. Python provides some of the robust libraries like NLTK, Spacy, TextBlob, Gensim, and Scikit-learn to conduct an in-depth analysis of text data.
Understanding the right library usage, data preprocessing techniques, the methodology of text mining and sentiment analysis will provide a strong foundation in the area of Natural Language Processing with Python.
Remember, the key to becoming proficient in text mining and sentiment analysis is continuous practice. So don’t stop at just reading this article; practice these techniques on diverse datasets!
Happy learning!