Reinforcement Learning Fundamentals: A Comprehensive Guide for Python Enthusiasts
Reinforcement learning (RL) is one of the most exciting areas of Machine Learning. It enables an agent to formulate intelligent behaviors based on interacting with an environment, also known as learning from experience. Python, with its ease of use and wide range of libraries suitable for scientific computing and artificial intelligence tasks, is the perfect language to start learning and implementing reinforcement learning methods.
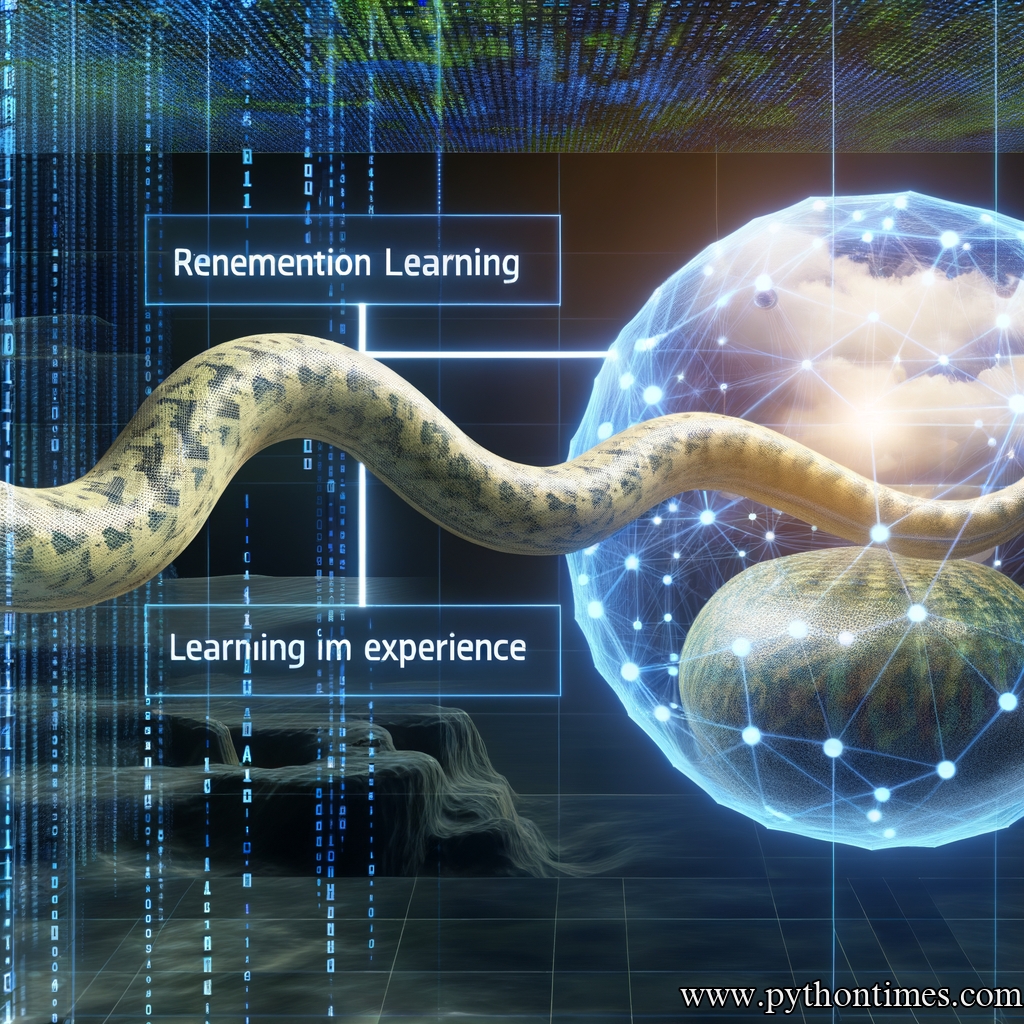
This article is purposed to enlighten both Python beginners and experienced enthusiasts on the principles behind reinforcement learning, why it’s vital, and how to implement it in Python. Buckle up!
Table of Contents 1. What is Reinforcement Learning? 2. Key Concepts in Reinforcement Learning 3. Markov Decision Process 4. Types of RL Methods 5. RL in Python: A Practical Approach 6. Summary and Conclusion
1. What is Reinforcement Learning?
Reinforcement Learning (RL) is an aspect of Machine Learning (ML) where an agent learns to behave in an environment, by performing certain actions and observing the results or feedback. It’s about making decisions sequentially. In contrast to supervised learning where a tutor corrects the learner’s mistakes, RL is about trial-and-error. With every wrong choice, the agent refines its policy which in turn helps to make future decisions.
There are three core components of any RL system – agent, environment, and actions. An agent
is the program or intelligence taking action based on feedback; the environment
is where the agent interacts and makes decisions. Actions are the steps that agents take while interacting with the environment.
RL draws from various disciplines including psychology, neuroscience, control theory, operations research, and computer science. It has a variety of practical applications in real-world scenarios such as game playing, robotics, supply chain management, and resource allocation.
2. Key Concepts in Reinforcement Learning
In the realm of RL, a few concepts appear frequently. Understanding them is essential for a firm grasp on how RL works.
- State: A state is the current condition, the snapshot of what the environment is at a time.
- Action: Actions are the steps that the agent can take while interacting with the environment.
- Reward: A reward is the feedback that the agent receives after making an action. It can be positive (for good actions) or negative (for bad actions).
- Policy: The policy is the strategy that defines the learning or decision-making process of an agent. It determines what action the agent will take in a given state.
- Value Function: A value function helps predict future rewards. There are two types: state-value function (V) which measures how good it is to be in a particular state, and action-value function (Q) which measures how good it is to perform a particular action in a given state.
- Discount Factor: The discount factor is a measure of how much importance is given to future rewards.
3. Markov Decision Process
A Markov Decision Process (MDP) is a mathematical model used often in RL. It provides a formal framework for decision-making where outcomes are partly random and partly under the control of a decision-maker. MDPs consist of:
- A set of states (S)
- A set of actions (A)
- A transition function T(s, a, s’) – the probability of transitioning to state s’ if action a is taken at state s
- A reward function R(s, a) – immediate reward received after transitioning to state s’ from s due to action a
- A discount factor gamma (γ)
MDPs satisfy the Markov property – the probability of transitioning to any particular state depends solely on the current state and action, not on the sequence of preceding states.
4. Types of RL Methods
Reinforcement learning methods are categorized into two main types:
- Value-based: The goal here is to find the optimal value function. Q-learning and DQN (Deep Q-Network) are examples of value-based methods.
- Policy-based: These methods aim to find the optimal policy. Monte Carlo (MC) methods, and Temporal Difference (TD) methods are examples.
5. RL in Python: A Practical Approach
Python, being a high-level, syntax-friendly language, offloads the cognitive burden and allows us to shift our focus on understanding, experimenting, and learning reinforcement learning.
Let’s look at a simple example of implementing Q-learning, a value-based RL method in Python using the gym
library. The task here is the classic balance the cart pole game.
# Importing necessary libraries
import gym
import numpy as np
# Setting up the environment
env = gym.make('CartPole-v0')
# Initialize q-table with zeros
Q = np.zeros([env.observation_space.n, env.action_space.n])
# Define the learning parameters
lr = .85
y = .99
num_episodes = 2000
for i in range(num_episodes):
# Reset the environment and get the first new state
s = env.reset()
d = False
j = 0
# The Q-Table learning algorithm
while j < 99:
j += 1
# Choose an action by greedily picking from Q table
a = np.argmax(Q[s,:] + np.random.randn(1, env.action_space.n)*(1./(i+1)))
# Get new state and reward from environment
s1, r, d, _ = env.step(a)
# Update Q-Table with new knowledge
Q[s,a] = Q[s,a] + lr*(r + y*np.max(Q[s1,:]) - Q[s,a])
s = s1
if d == True:
break
print("Q-Table Values")
print(Q)
In this Python example, we’re using an environment provided by gym
, setting up a Q-table to store our state-action values, and then using a simple loop to carry out the learning process.
6. Summary and Conclusion
In this article, we’ve taken a comprehensive look at Reinforcement Learning, key concepts, types, and how to implement a simple RL method in Python. RL is deep, exciting, and offers broad applicability. Python indeed brings these capabilities closer to both beginners and experienced practitioners alike, making it easier to prototype, test, and develop robust RL-based systems.
As the saying goes, practice makes perfect. So I encourage you to explore further, try out more complex applications, and immerse yourself in the fascinating world of Reinforcement Learning. The knowledge of Python and Reinforcement Learning together certainly holds the potential to take you places. Enjoy the journey!
Resources: 1. Sutton, R. S., & Barto, A. G. (2018). Reinforcement Learning: An Introduction 2. Gym: Gym is a toolkit for developing and comparing reinforcement learning algorithms
Happy Python Programming!