Pythonic Patterns: Best Practices for Writing Elegant Code
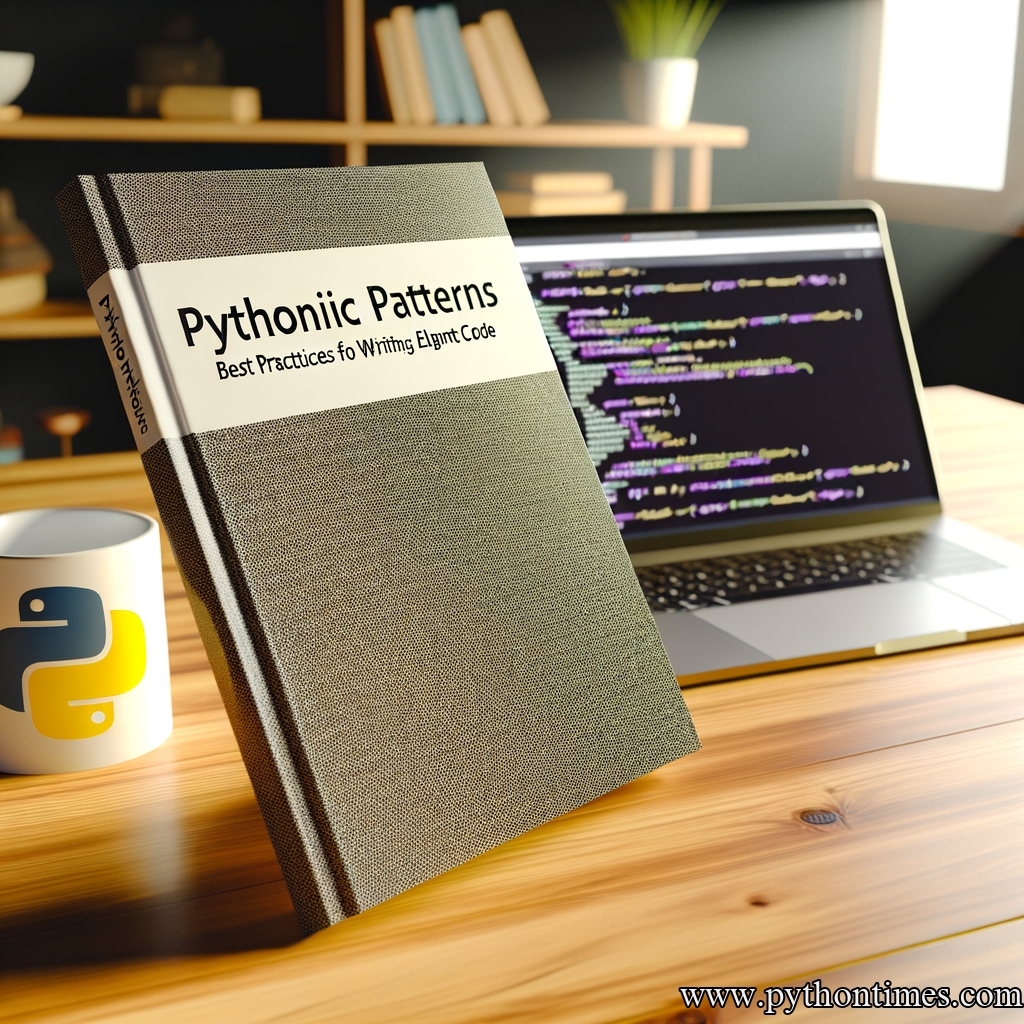
Introduction
Welcome to PythonTimes.com, your ultimate resource for all things Python! In this article, we will explore Pythonic Patterns: Best Practices for Writing Elegant Code. Whether you are a beginner getting started with Python or a seasoned professional looking to enhance your coding style, this guide will provide valuable insights and practical examples to help you write elegant code.
Why Pythonic Patterns Matter
Python, known for its readability and simplicity, offers developers a wide range of coding approaches. However, not all code is created equal. Pythonic Patterns are a set of guidelines and best practices that promote clean, concise, and efficient code. Adhering to these patterns helps improve code quality, readability, and maintainability, ultimately saving time and effort in the long run.
Simplicity is Key
At the heart of Pythonic Patterns lies the principle of simplicity. Python’s creator, Guido van Rossum, famously stated, “Readability counts.” By following Pythonic Patterns, we can write code that is not only functional but also easy to understand. This is crucial when collaborating with teammates or maintaining code in the future.
Now, let’s dive into some of the most essential Pythonic Patterns that will elevate your code to a higher standard.
Pattern 1: Writing Idiomatic Loops with List Comprehension
Consider the following task: given a list of numbers, we want to create a new list that contains only the even numbers. Using traditional loops, we could write something like this:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = []
for num in numbers:
if num % 2 == 0:
even_numbers.append(num)
While this code achieves the desired result, it can be simplified using list comprehension:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = [num for num in numbers if num % 2 == 0]
List comprehension allows us to express the same logic in a single line. This compact and Pythonic approach not only reduces code clutter but also improves readability by eliminating unnecessary variable declarations.
In addition to filtering elements, list comprehension can be used to perform calculations on the filtered elements. Let’s say we want to create a new list that contains the square of each even number:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_squares = [num ** 2 for num in numbers if num % 2 == 0]
By leveraging list comprehension, we achieve the desired result with elegance and brevity.
Pattern 2: Leveraging Context Managers with the ‘with’ Statement
Resource management is a common concern in programming. Python provides a powerful mechanism called context managers to handle resources such as files, network connections, or locks. Using context managers ensures that resources are properly managed and released, even in the presence of exceptions.
Traditionally, resource management involves manually opening and closing the resource:
file = open('data.txt', 'r')
try:
# Do something with the file
finally:
file.close()
With the introduction of the with
statement, managing resources becomes more concise and Pythonic:
with open('data.txt', 'r') as file:
# Do something with the file
The with
statement automatically takes care of closing the resource, even if an exception occurs within the block. This pattern improves code readability and reduces the chance of resource leaks.
Creating Custom Context Managers
In addition to built-in context managers, Python allows you to create your own custom context managers using the contextlib
module or by defining a class with __enter__
and __exit__
methods.
Consider the following example where we want to measure the execution time of a block of code:
import time
class Timer:
def __enter__(self):
self.start_time = time.time()
return self
def __exit__(self, exc_type, exc_val, exc_tb):
total_time = time.time() - self.start_time
print(f"Execution time: {total_time} seconds")
with Timer():
# Code block to measure execution time
time.sleep(2)
By defining the __enter__
and __exit__
methods, we can use the Timer
class as a context manager. The code inside the with
block will be timed, and the result will be printed once the block is exited.
Custom context managers provide a flexible and reusable way to manage resources and add functionality to code blocks.
Pattern 3: Embrace the Power of Generators
Generators are a powerful Python feature that allows us to create iterators in a memory-efficient and lazy fashion. Instead of generating all the values at once, generators produce values on the fly, as they are needed. This enables processing of large datasets or infinite sequences without consuming excessive memory.
To illustrate the difference between traditional lists and generators, let’s consider the Fibonacci sequence. We can create a list of Fibonacci numbers up to a certain limit using a loop:
def fibonacci_list(limit):
fib_numbers = [0, 1]
while fib_numbers[-1] < limit:
next_num = fib_numbers[-1] + fib_numbers[-2]
fib_numbers.append(next_num)
return fib_numbers[:-1]
This function generates all Fibonacci numbers up to the given limit and stores them in a list. If the limit is high or unlimited, this approach can consume a significant amount of memory.
On the other hand, we can build a Fibonacci generator that generates the numbers on the fly:
def fibonacci_generator(limit):
a, b = 0, 1
while a < limit:
yield a
a, b = b, a + b
The yield
keyword transforms the function into a generator. Calling this generator produces the Fibonacci numbers one by one, conserving memory and allowing for efficient processing.
Generators can be used in a variety of scenarios, including reading large files, parsing XML or JSON data, and generating an infinite sequence of random numbers.
Pattern 4: DRY – Don’t Repeat Yourself
One of the golden principles in programming is to avoid repetition. Pythonic Patterns strongly encourage adhering to the DRY principle to promote code reusability and maintainability.
Repetition often occurs when performing similar operations on multiple objects or when implementing similar functionality in different parts of the code. By identifying and eliminating duplication, we can write more concise and maintainable code.
Let’s take a look at an example where we can apply the DRY principle. Suppose we have two functions that perform similar calculations on different input parameters:
def calculate_area(length, width):
return length * width
def calculate_volume(length, width, height):
return length * width * height
We can refactor these two functions using a generic calculate
function:
def calculate(*args):
result = 1
for value in args:
result *= value
return result
By using the variadic *args
parameter and a simple loop, we can calculate the area, volume, or any other similar calculation with a single function. This approach reduces code duplication, improves code readability, and makes it easier to maintain and modify in the future.
Remember, every time you find yourself copy-pasting code, think of ways to abstract and generalize the functionality to eliminate redundancy.
Conclusion
In this article, we explored Pythonic Patterns: Best Practices for Writing Elegant Code. By embracing these patterns, we can write code that is not only functional but also readable, maintainable, and efficient. We covered essential patterns such as list comprehension, using context managers with the with
statement, leveraging the power of generators, and following the DRY principle to avoid repetition.
Remember, writing elegant code is a continuous learning process. As you become more experienced and comfortable with Python, keep exploring new patterns, and strive to improve your coding skills. By following Pythonic Patterns, you will not only write better code but also become a more efficient and effective programmer.
Now it’s time to put these patterns into practice and let your Pythonic code shine!