Introduction to Reinforcement Learning with OpenAI Gym in Python
Reinforcement Learning (RL) is a fascinating field of Artificial Intelligence that can allow machines and software agents to autonomously decide the optimal behavior within a specific context, to improve their performance. It is a significant departure from other machine learning algorithms, that are mainly based on supervised or unsupervised learning.
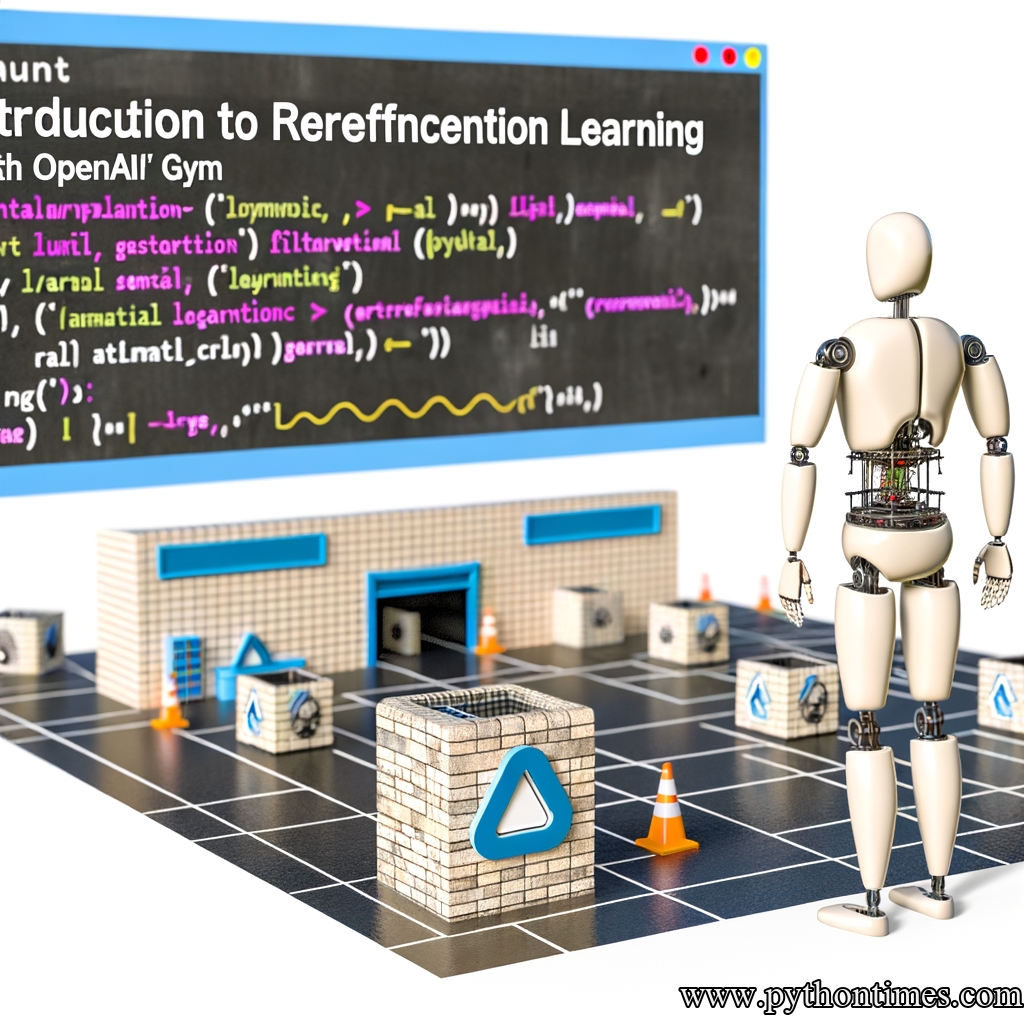
This introductory tutorial will cover reinforcement learning and its implementation using OpenAI Gym, a popular Python library for developing and comparing reinforcement learning algorithms.
Table of Contents
- Introduction to Reinforcement Learning.
- Overview of OpenAI Gym.
- Getting started with OpenAI Gym.
- Building a RL agent using OpenAI Gym.
- Understanding the results and next steps.
Introduction to Reinforcement Learning
Reinforcement Learning involves training a software agent that can learn to make decisions by trial and error. It can be broken down into three main components:
-
Agent: This is the entity (or software) that is making the decisions.
-
Environment: This is where the agent operates.
-
Action: This is the tasks that the agent performs.
In reinforcement learning, the agent observes the environment, decides which action to perform based on its current state, and performs that action. The environment then gives the agent feedback in terms of rewards or penalties. Positive rewards help the agent know it has performed a good action, and negative rewards (or penalties) indicate a bad action.
The agent’s decision-making strategy is known as a Policy. The goal of reinforcement learning is to train an agent to establish a policy that maximizes the total cumulative reward.
Overview of OpenAI Gym
OpenAI Gym, created by OpenAI, an artificial intelligence research lab, is a fantastic toolkit for developing and comparing reinforcement learning algorithms. It supports teaching agents everything from walking to playing games like Pong or Pinball.
Gym provides several predefined environments for training and testing reinforcement learning agents, including simulations of physical tasks. It also provides a straightforward and consistent interface for users to work with these environments.
Getting Started with OpenAI Gym
Before diving into reinforcement learning training, let’s set up OpenAI Gym and familiarize ourselves with its environment.
Installation:
pip install gym
When importing gym, an environment is created:
import gym
env = gym.make('CartPole-v1')
The make()
function takes an environment ID string, following a specific convention. ‘CartPole-v1’ is one of the many pre-built environments in OpenAI Gym.
To initialize this environment:
env.reset()
Using the reset()
method, we start our environment afresh.
And, to finish and close our environment:
env.close()
Building a Reinforcement Learning agent using OpenAI Gym
The problem we’re working on here is ‘CartPole-v1’, a popular beginner’s environment where we balance a pole on a cart.
Our goal throughout this tutorial will be to create an agent to maintain balance.
Here’s how the environment is set-up.
import gym
env = gym.make('CartPole-v1')
env.reset()
for _ in range(1000):
env.render()
env.step(env.action_space.sample()) # Take a random action
env.close()
In this code, step()
function returns four values:
– observation
(object): an environment-specific object representing your observation of the environment. In the “CartPole” environment, it’s a 4-dimensional array containing information about the position and velocity of the cart and pole.
– reward
(float): the amount of reward achieved by the previous action. The goal is to increase your total reward.
– done
(boolean): whether it’s time to reset the environment.
– info
(dict): diagnostic information useful for debugging.
From here, we then strive to create a better policy for the agent so that it can perform operations more wisely.
import gym
env = gym.make('CartPole-v1')
for i_episode in range(20):
observation = env.reset()
for t in range(100):
env.render()
print(observation)
action = env.action_space.sample()
observation, reward, done, info = env.step(action)
if done:
print("Episode finished after {} timesteps".format(t+1))
break
env.close()
Understanding the Results and Next Steps
By running the above codes, we can observe the agent learning from its surroundings and improving the scores over the episodes. The agent starts making the correct decisions, maintaining the balance, and a score of 200 proves that the pole was successfully balanced for 200 steps.
OpenAI Gym provides us with the necessary tools to design and evaluate algorithms in reinforcement learning. Once we learn the basics, we can head over to explore more complex environments and algorithms.
Remember, the field of reinforcement learning is vast and complex; don’t be discouraged if things don’t make sense immediately. Keep practicing, keep deepening your understanding, and soon you’ll be building some incredibly intelligent agents.
Sources: 1. OpenAI Gym Official Documentation 2. Reinforcement Learning 3. Hands-On Machine Learning with Scikit-Learn, Keras, and TensorFlow, 2nd Edition