Image Classification with Convolutional Neural Networks (CNNs) in Python
In the field of Artificial Intelligence, particularly in Computer Vision, one of Python’s most powerful and widely used libraries is Keras, which offers a comprehensive selection of tools for building neural networks. Particularly relevant for image processing, are Convolutional Neural Networks (CNNs) – a class of deep learning neural networks. CNNs are primarily used for image processing, classification, segmentation and also for other auto-correlative data.
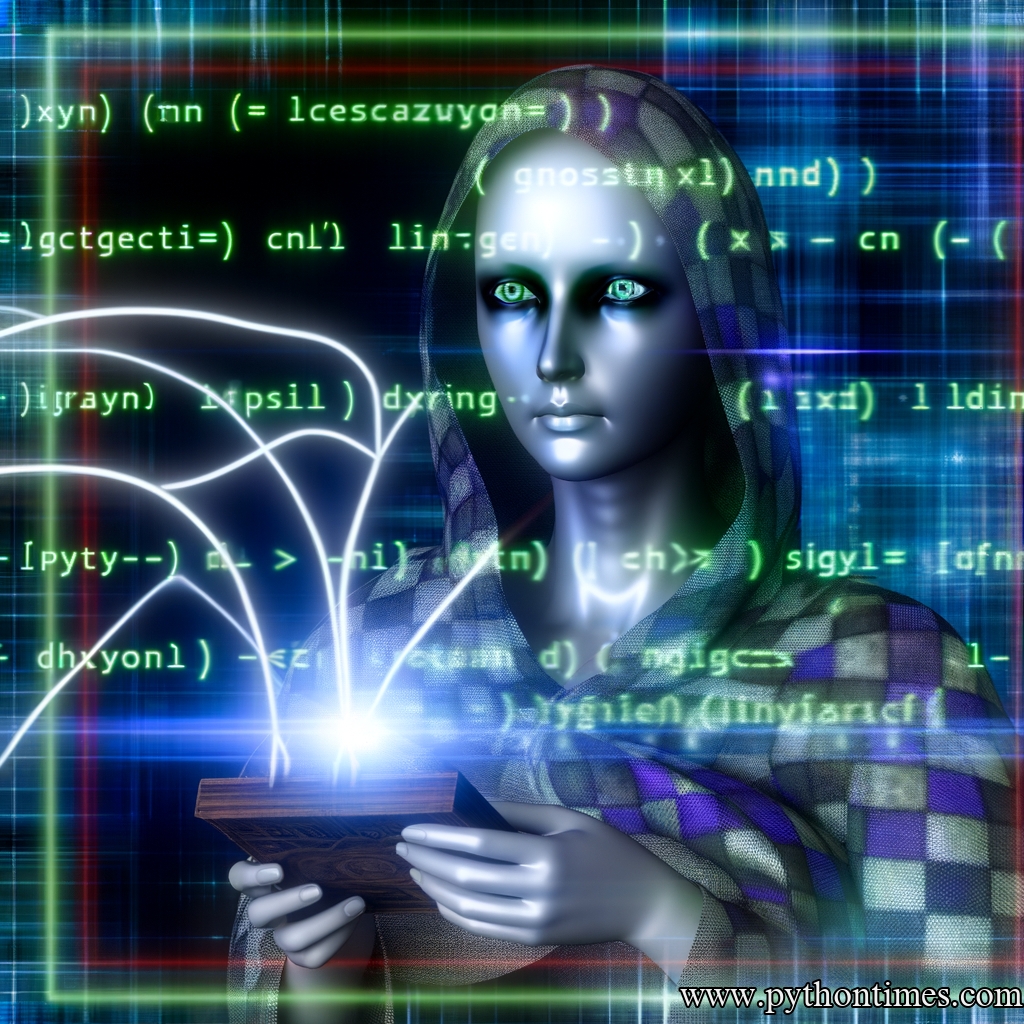
This article will explore the process of CNN-based image classification using Python’s Keras library. Although this guide will serve Python programmers of all expertise levels, a basic understanding of Python programming and familiarity with machine learning concepts will be advantageous.
Table of Contents
- What is Image Classification?
- Understanding Convolutional Neural Networks (CNNs)
- Conceptual Building Blocks of CNNs
- Building a CNN with Python and Keras
- Training and Evaluating the Model
- Summary
1. What is Image Classification?
Image Classification is a task of machine learning/deep learning in which we classify images based on the human-defined categories. It is a core task in Computer Vision that, despite its simplicity, has a large variety of practical applications.
For instance, a self-driving car needs to identify objects, such as traffic lights, pedestrians, and other vehicles, in its vicinity to navigate properly. Similarly, healthcare industries use image classification techniques for disease detection by training a model with images of various conditions and their respective classifications.
2. Understanding Convolutional Neural Networks (CNNs)
CNNs are a category of Neural Networks that have proven very effective in areas such as image recognition and classification. Convolutional Networks take advantage of the spatial nature of the data. In other words, they understand that pixels close to each other are often related and constitute parts of an object or objects in an image.
A typical CNN design begins with a convolutional layer for filtering input data, followed by a pooling layer to reduce the data dimensions, and finally, one or more fully connected layers for classification. The objective of this layered architecture is to create a detailed map of the image features, making CNNs adept at understanding the intricate patterns in data.
3. Conceptual Building Blocks of CNNs
3.1 Convolutional Layer
The core building block of a CNN is the convolutional layer. In this layer, several filters are used to perform convolution operations on the input data. These filters also known as ‘kernels’, can capture the spatial dependencies in the image.
3.2 Pooling Layer
The pooling layer comes after the Convolutional layer, which is used to reduce the spatial dimensions (Width x Height) but maintaining the depth. It works by sliding a ‘window’ across the input and feeding the content of the window to a pooling function (like MAX or AVG) that summarises the feature.
3.3 Fully Connected Layer
The final layers of a CNN are fully connected layers. Neurons in a fully connected layer have full connections to all activations in the previous layer. Their purpose is to use these features for classifying input image into various classes based on the training dataset.
4. Building a CNN with Python and Keras
Now that you have a basic understanding of CNNs, we can implement this in Python using Keras on a classic dataset: MNIST. MNIST is a widely used dataset for handwritten digit classification, and it has a training set of 60,000 examples, and a test set of 10,000 examples.
4.1 Importing Necessary Libraries
At the beginning of our Python script, we import the necessary libraries with the following lines of code:
import keras
from keras.datasets import mnist
from keras.models import Sequential
from keras.layers import Dense, Dropout, Flatten
from keras.layers import Conv2D, MaxPooling2D
from keras import backend as K
4.2 Loading Data
Then, we load our MNIST dataset with the line:
(x_train, y_train), (x_test, y_test) = mnist.load_data()
4.3 Preprocessing
After that, data preprocessing is performed:
# Reshaping the array to 4-dims so that it can work with the Keras API
x_train = x_train.reshape(x_train.shape[0], 28, 28, 1)
x_test = x_test.reshape(x_test.shape[0], 28, 28, 1)
input_shape = (28, 28, 1)
# Making sure that the values are float so that we can get decimal points after division
x_train = x_train.astype('float32')
x_test = x_test.astype('float32')
# Normalizing by dividing it by the max RGB value.
x_train /= 255
x_test /= 255
4.4 Building the CNN Model
Now, we will define our model using Keras’ Sequential API:
model = Sequential()
# Adding first convolutional layer
model.add(Conv2D(28, kernel_size=(3,3), input_shape=input_shape))
model.add(MaxPooling2D(pool_size=(2, 2)))
# Flattening the 2D arrays for fully connected layers
model.add(Flatten())
# Adding dense layer with 128 neurons
model.add(Dense(128, activation=tf.nn.relu))
# Adding dropout layer
model.add(Dropout(0.2))
# Adding output layer with 10 neurons
model.add(Dense(10,activation=tf.nn.softmax))
5. Training and Evaluating the Model
With the model defined, we will now compile and train our model using the ADAM optimizer:
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
model.fit(x=x_train,y=y_train, epochs=10)
We can now evaluate the model using the following line of code:
model.evaluate(x_test, y_test)
6. Summary
This article provided an overview of classifying images with Convolutional Neural Networks (CNNs) using Python’s Keras. We briefly introduced image classification and why it is a significant application of AI. Then we explored CNNs and how they are designed.
After understanding the fundamental building blocks of CNNs – convolutional layers, pooling layers, and fully connected layers, we implemented a CNN in Python using the Keras library and trained it on the MNIST dataset.
Remember, while this example is relatively simple, the principles and methods used can be applied to much larger and complex datasets and models. CNNs are powerful tools for image classification and are used in real-world applications across various industries. Happy Coding!