Going Beyond Basics: Advanced Tips for Pythonistas
Python is an incredibly versatile and powerful programming language, fueled by a vibrant community of developers and enthusiasts. Whether you’re just starting out or have been coding in Python for years, there are always new tips and tricks to enhance your skills and take your Python game to the next level. In this article, we’ll dive into some advanced tips for Pythonistas, providing you with valuable insights, practical examples, and real-world applications to expand your horizons.
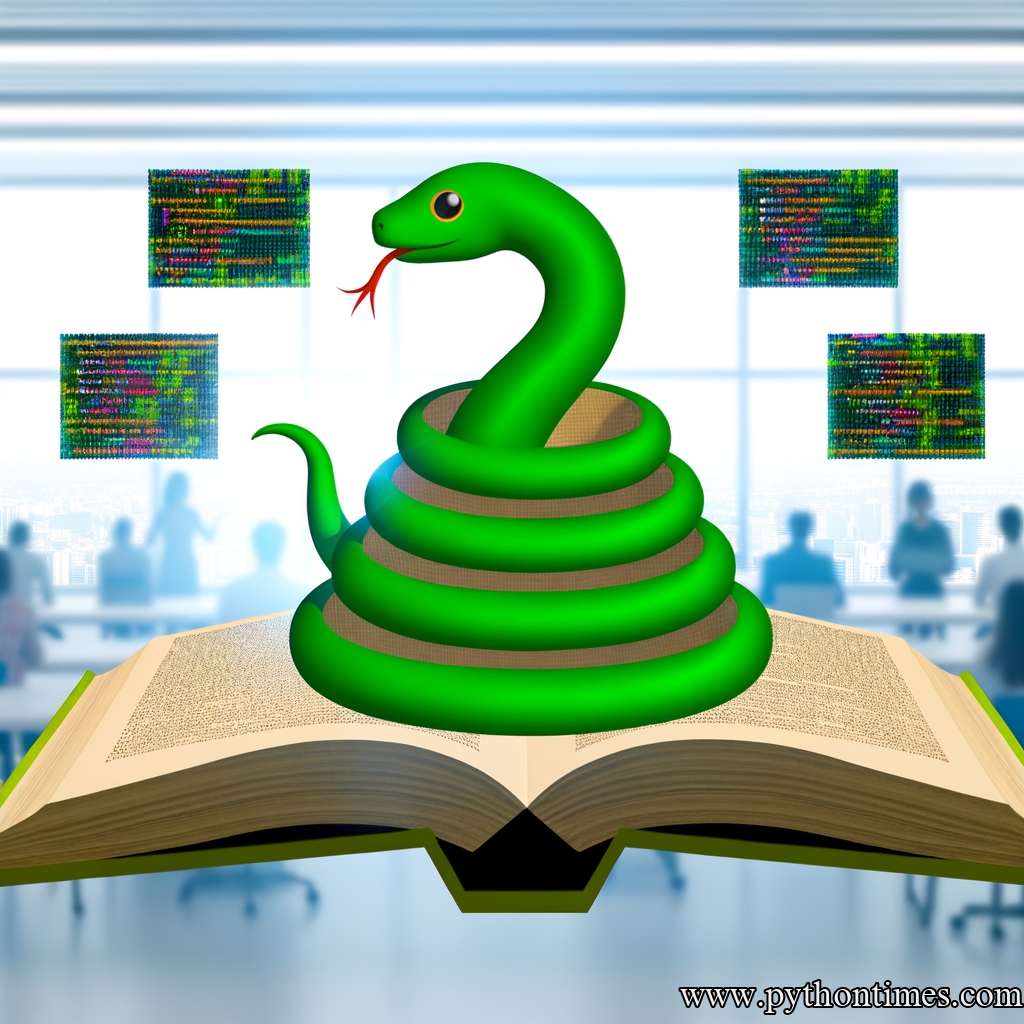
Leveling Up Your Python Skills
As you progress in your Python journey, it’s important to continuously challenge yourself and explore new concepts. Going beyond the basics not only allows you to solve more complex problems but also equips you with the knowledge to write efficient, robust, and maintainable code. Let’s explore some advanced tips that will sharpen your Python skills and make you a more proficient Pythonista.
Tip 1: Mastering Decorators
Decorators are a powerful feature in Python that allow you to modify the behavior of functions or classes without modifying their source code. They provide an elegant way to extend the functionality of existing code, making it more reusable and flexible. Understanding decorators and knowing how to create and use them is a hallmark of an advanced Pythonista.
To illustrate this concept, let’s consider a scenario where you want to log the execution time of a function. Instead of modifying the function code directly, you can create a decorator that wraps the function and adds the logging logic. Here’s an example:
import functools
import time
def log_execution_time(func):
@functools.wraps(func) # Preserve original function metadata
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
execution_time = end_time - start_time
print(f"Execution time of {func.__name__}: {execution_time} seconds")
return result
return wrapper
@log_execution_time
def my_function():
# Function code here
my_function()
In this example, the log_execution_time
decorator wraps the my_function
and adds the timing logic. By using the functools.wraps
decorator, we preserve the original function’s metadata, such as its name and docstring.
Decorators are not limited to logging execution time; they can be used for authentication, caching, input validation, and much more. The key takeaway is that decorators provide a concise and reusable way to modify the behavior of functions and classes, making your code more modular and maintainable.
Tip 2: Context Managers for Resource Management
Resource management is a critical aspect of any programming language, and Python offers a convenient way to handle it through context managers. Context managers help you allocate and release resources (like files or network connections) automatically, ensuring proper cleanup and preventing resource leaks.
Python’s with
statement is the key to working with context managers. It allows you to define a block of code within a context manager using the with
keyword. Let’s examine an example using the built-in open
function for file handling:
with open('file.txt', 'r') as file:
contents = file.read()
# Do something with the file contents
# Code here is outside the context, and the file is automatically closed
In this example, the open
function returns a file object that serves as a context manager. By enclosing the file operations within the with
statement, you ensure that the file is closed properly, even if an exception occurs within the block.
You can also create your own custom context managers using the contextlib
module or by implementing the __enter__
and __exit__
methods in a class. Custom context managers can be useful when working with resources that require more complex setup and teardown operations.
Tip 3: Generators for Memory Optimization
When dealing with large datasets or computationally expensive tasks, it’s essential to optimize memory usage. Generators provide an efficient way to work with sequences of data without loading them entirely into memory.
A generator is a special type of function that returns an iterator. Instead of computing and returning the entire sequence at once, generators produce elements one at a time, on the fly. This feature enables you to process large datasets or perform expensive calculations without overwhelming your system’s memory.
Let’s consider an example where we need to compute the sum of all even numbers between 1 and 10^6:
def even_numbers(n):
for i in range(2, n+1, 2):
yield i
even_sum = sum(even_numbers(10**6))
print(even_sum)
In this example, the even_numbers
function is a generator that yields even numbers between 2 and n
. By using the sum
function on the generator, we can compute the sum of all even numbers up to 10^6 without storing them all in memory.
Generators are also handy when dealing with infinitely large sequences, such as streaming data or numerical series. Additionally, they can be combined with other Python features like list comprehensions and the itertools
module for more advanced use cases.
Tip 4: Profiling and Optimization Techniques
As a Pythonista, it’s crucial to write efficient code that performs well. While Python is known for its simplicity and readability, it can sometimes be slower compared to other programming languages. However, with the right profiling and optimization techniques, you can identify bottlenecks and improve the performance of your Python code.
Python provides several built-in modules and third-party packages for profiling and optimization. Some popular options include:
- cProfile: This built-in module allows you to profile your Python code and analyze its performance characteristics. By identifying the hotspots, you can focus your optimization efforts on the most time-consuming parts.
-
line_profiler: This third-party package extends the functionality of
cProfile
by providing line-by-line profiling. It helps you pinpoint specific lines of code that are causing performance issues. - numba: This third-party package offers just-in-time (JIT) compilation for Python code. By adding type annotations and using the provided decorators, you can significantly speed up your code.
-
pandas and
numpy
: These third-party packages are optimized for data manipulation and numerical computations, respectively. They provide highly efficient data structures and functions for working with large datasets.
By using these profiling and optimization techniques, you can identify performance bottlenecks, optimize critical sections of your code, and leverage specific libraries tailored for high-performance computing.
Tip 5: Multithreading and Multiprocessing
Python’s Global Interpreter Lock (GIL) imposes a limitation on the use of multiple threads for parallel processing. However, the threading
module still offers benefits in scenarios where code execution is limited by factors other than CPU-bound tasks, such as I/O operations.
For CPU-bound tasks, multiprocessing is the way to go. The multiprocessing
module allows you to take advantage of multiple CPU cores by creating separate processes. Each process has its own memory space and Python interpreter, effectively bypassing the GIL limitation.
Let’s see a basic example that demonstrates the difference between multithreading and multiprocessing for CPU-bound tasks:
import time
import multiprocessing
import threading
def cpu_intensive_task():
total = 0
for _ in range(10**8):
total += 1
return total
# Multithreading
start_time = time.time()
with concurrent.futures.ThreadPoolExecutor() as executor:
results = [executor.submit(cpu_intensive_task) for _ in range(4)]
total_time = time.time() - start_time
print(f"Multithreading: {total_time} seconds")
# Multiprocessing
start_time = time.time()
with concurrent.futures.ProcessPoolExecutor() as executor:
results = [executor.submit(cpu_intensive_task) for _ in range(4)]
total_time = time.time() - start_time
print(f"Multiprocessing: {total_time} seconds")
In this example, we leverage the concurrent.futures
module, which provides a high-level interface for asynchronous computation. By submitting the CPU-intensive task to multiple threads or processes, we can observe the difference in execution time between multithreading and multiprocessing.
It’s important to note that the decision to use multithreading or multiprocessing depends on the nature of your task and the resources available. For I/O-bound tasks or scenarios where the GIL doesn’t pose a significant limitation, multithreading can offer performance improvements. However, for CPU-bound tasks, multiprocessing is usually the preferred choice.
Conclusion
Congratulations! You’ve successfully expanded your Python repertoire with advanced tips and techniques. By mastering decorators, understanding context managers, leveraging generators, optimizing your code, and utilizing multithreading and multiprocessing, you are well on your way to becoming a seasoned Pythonista.
Remember, Python’s versatility and extensibility make it a powerful tool for a wide range of applications. Whether you’re exploring data science, building web applications, or working on machine learning projects, these advanced tips will give you an edge in writing efficient, maintainable, and high-performance Python code.
So, keep pushing your boundaries, stay curious, and continue exploring the vast world of Python. Happy coding, Pythonistas!