Exploring WebSockets in Django
WebSockets allow for full-duplex communication channels over a single TCP connection, providing a more efficient communication method over HTTP. WebSockets serve as an excellent tool for building real-time applications like chat apps, live updates, and more.
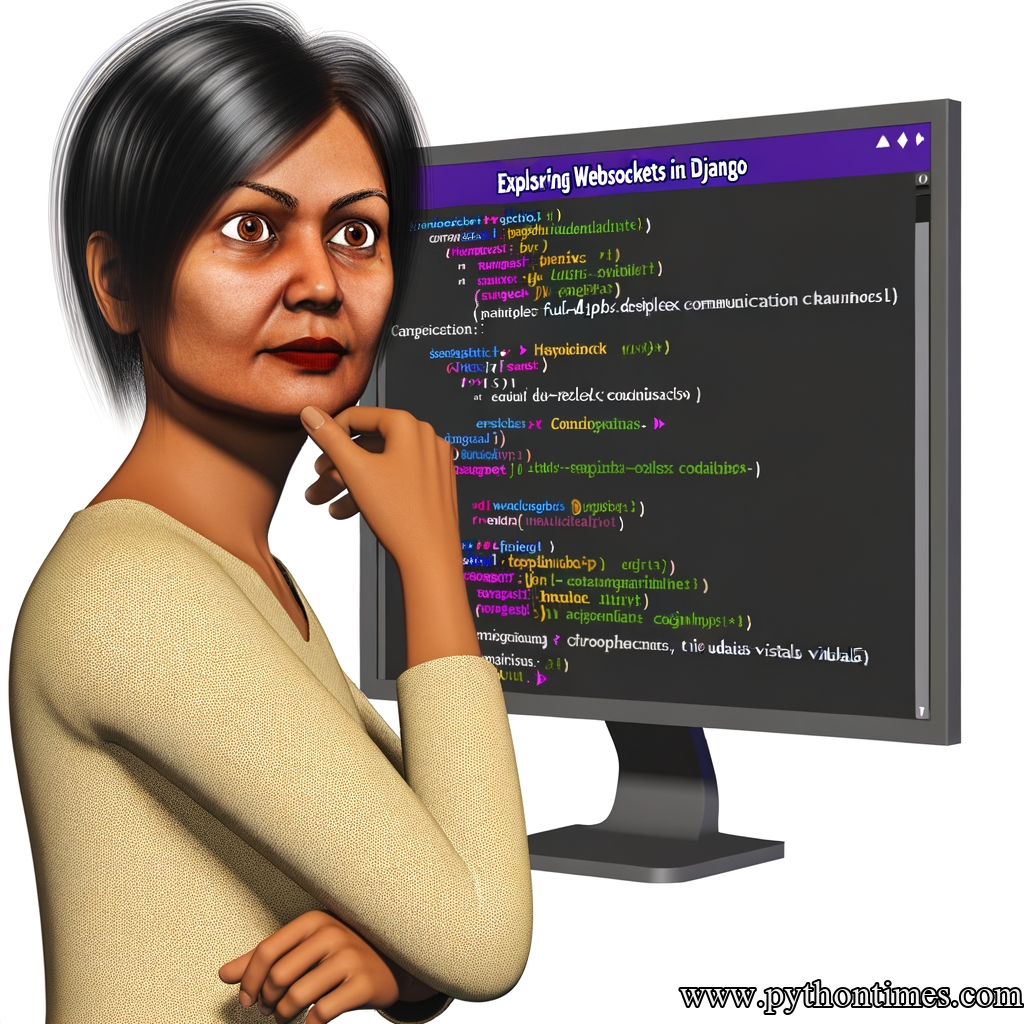
In the world of Python, Django remains a popular framework for developing powerful web applications, and with the integration of WebSockets, the functionality increases significantly. Let’s dive into the world of WebSockets with Django. Our investigation will include:
- Introduction to WebSockets
- Understanding the Django Channels
- Installing and Setting Up Django Channels
- Creating a Simple WebSocket Application using Django
- Handling Errors and Edge Cases
- Tips and Tricks for Using WebSockets with Django
Introduction to WebSockets
WebSockets is a protocol, providing bidirectional communication between the client and the server. Unlike HTTP that initiates a request from client-side only, WebSockets gives the server a way to send data to the client without being explicitly requested. This process makes real-time communication more efficient, specifically in scenarios like gaming, chats, live feeds, etc.
For Python developers, Django is often a go-to choice for developing web applications. However, Django was initially designed for HTTP, not for handling WebSocket requests. Hence, Django Channels were introduced.
Understanding Django Channels
Django Channels is an official Django project that extends Django to handle WebSockets, HTTP2, and other protocol types. It serves as a complex system that allows Django applications to not just handle HTTP, but also handle the protocols that require long-term connections like WebSockets.
Channels redefine Django’s view for a synchronous callable into an asynchronous one, handled by the ASGI (Asynchronous Server Gateway Interface).
Installing and Setting up Django Channels
To utilize channels, we need to install both Django and channels using pip:
pip install django
pip install channels
Since Channels treats each Django project as an application in the Channels routing, you need to set an instance of ProtocolTypeRouter
as your root application.
In your settings.py
, add channels:
INSTALLED_APPS = (
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.sites',
'channels',
)
Now set ProtocolTypeRouter
with channels in the settings:
ROOT_URLCONF = 'mysite.urls'
WSGI_APPLICATION = 'mysite.wsgi.application'
ASGI_APPLICATION = "mysite.routing.application"
Creating a Simple WebSocket Application in Django
With Django Channels set up, we can now go ahead and create a simple chat app.
Step 1: Set up WebSocket routing
Create a new file routing.py
in your app directory and include the routing information:
from django.urls import path
from . import consumers
websocket_urlpatterns = [
path('ws/chat/<str:room_name>/', consumers.ChatConsumer),
]
Step 2: Create the consumer
In your app directory, create consumers.py
. We import AsyncWebsocketConsumer
, which we subclass to create our own.
import json
from channels.generic.websocket import AsyncWebsocketConsumer
class ChatConsumer(AsyncWebsocketConsumer):
async def connect(self):
self.room_name = self.scope['url_route']['kwargs']['room_name']
self.room_group_name = 'chat_%s' % self.room_name
await self.channel_layer.group_add(
self.room_group_name,
self.channel_name
)
await self.accept()
async def disconnect(self, close_code):
await self.channel_layer.group_discard(
self.room_group_name,
self.channel_name
)
# Receive message from WebSocket
async def receive(self, text_data):
text_data_json = json.loads(text_data)
message = text_data_json['message']
await self.channel_layer.group_send(
self.room_group_name,
{
'type': 'chat_message',
'message': message
}
)
# Receive message from room group
async def chat_message(self, event):
message = event['message']
# Send message to WebSocket
await self.send(text_data=json.dumps({
'message': message
}))
Step 3: Front End HTML Template
Create a HTML template which will use JavaScript to create a WebSocket connection.
<script>
var socket = new WebSocket('ws://localhost:8000/ws/chat/{{ room_name }}/');
socket.onmessage = function(e) {
alert(e.data);
};
socket.onclose = function(e) {
alert('The socket is closed');
};
function sendMessage(){
var messageInput = document.getElementById('chat-message');
socket.send(JSON.stringify({
'message': messageInput.value
}));
}
</script>
<input id="chat-message" type="text">
<button onclick="sendMessage()">Send</button>
That’s it! You have created a simple real-time chat app using Django Channels and WebSockets.
Handling Errors and Edge Cases
While working with WebSockets, it is essential to handle all the edge cases to ensure smooth user experiences. Common cases to consider include:
- Handling failures when creating a WebSocket connection
- Managing scenarios when the WebSocket connection is dropped
- Dealing with large data payloads over WebSockets
In each case, you should include appropriate fallback methods and procedures that assure the sustainment of application integrity and seamless user experience.
Tips & Tricks for Working with WebSockets in Django
- Optimize for performance – avoid blocking in code.
- Use Django’s Channels layers abstraction to simplify dealing with complex routing.
- WebSocket connection is not guaranteed, handle exceptions accordingly.
- Keep tracks of your worker and background tasks, use libraries like Celery.
- Always secure your WebSocket connections using Django’s built-in security features.
Conclusion
WebSockets open new avenues for creating rich, real-time experiences on the web. By using Django Channels, even complex WebSocket functionalities become accessible and manageable. With good practices, you can create robust and efficient real-time Django applications using WebSockets.
Remember, keep exploring, stay connected and make things happen with Django and WebSockets. Happy Coding!