Effective Use of Comments in Python Code
Comments are one of the most powerful tools a coder can wield when it comes to making their scripts comprehensible to other developers or their future selves. Through this discussion, we will delve into the depths of how these vital fragments of text can be utilized effectively in the dynamic, pythonic world of Python code.
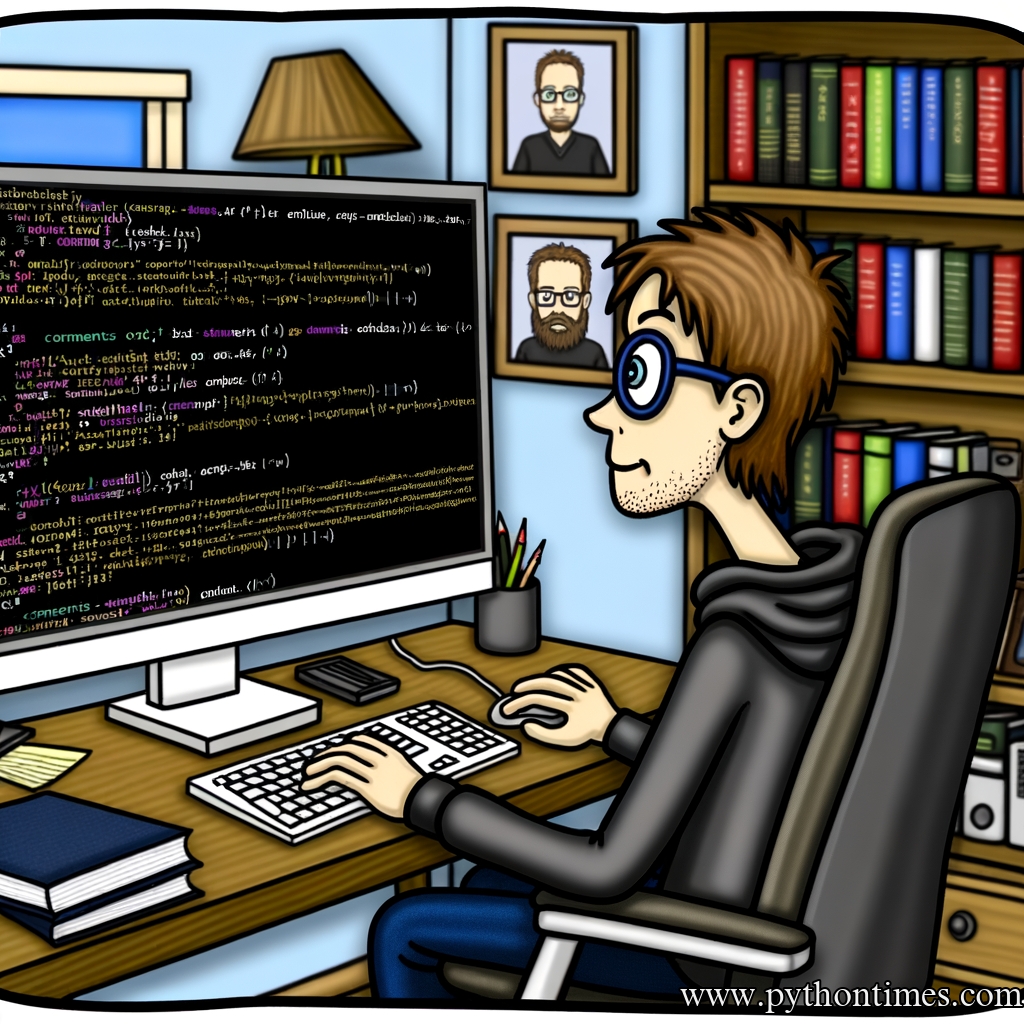
Table of Contents
- Introduction
- Importance of Comments in Python Code
- Types of Comments in Python
- The Effective Use of Comments in Python
- Best Practices to Use Comments in Python
- Common Pitfalls of Commenting in Python
- Conclusion
Introduction
In the simplest of terms, comments in Python are lines in a script that are not executed and solely serve the purpose of providing additional context and understanding for the reader. They are denoted by a hashtag (#
) and can be included either as single-line or multi-line comments.
# This is a single-line comment in Python
'''
This is a multi-line comment
or also known as a docstring in Python
'''
Importance of Comments in Python Code
There are three main reasons why commenting is vital in Python development:
- Understandability: By annotating what the specific blocks of code do, it becomes much easier for another developer (or you in the future) to understand the purpose and functionality of the code.
- Debugging: Comments are helpful in testing hypotheses during debugging. For instance, a section of code can be ‘commented out’ to check if it’s causing a bug.
- Documentation: When using docstrings, Python comments also form a significant basis for the automatically generated documentation.
Types of Comments in Python
Python recognizes two forms of comments:
- Single-line Comments: These are the most common form of comments and are denoted by
#
symbol.
# This is a single-line comment
print('Hello, Python Times readers!') # Comment after a statement
- Multi-line Comments (Docstrings): Python has a unique feature for multi-line comments—docstrings. Docstrings or document strings are strings that are used as comments. They can span multiple lines and are enclosed within triple quote marks (
'''
) or double quote marks ("""
). Docstrings are especially important as they are used in automatic documentation systems.
'''
This is a multi-line comment in Python.
It extends beyond just one line.
'''
"""
This is also a multi-line comment or docstring.
"""
The Effective Use of Comments in Python
Strategic and meaningful commenting is a skill that developers need to hone. Here are some important tips:
- Code Explanation: Comments should elucidate complex or non-obvious parts of the code. For instance, if you are using a complicated algorithm, a short overview of how it functions can be useful.
- Code Sectioning: Comments are often used to structurally divide the code into logical sections, helping in quickly locating certain parts.
- Highlighting: They can be used to highlight certain parts of the code, like TODOs or potential bugs.
- Debugging: Comments expedite the debugging operations by allowing the developers to ‘mute’ specific lines or sections of code.
Best Practices to Use Comments in Python
Developing a productive commenting strategy requires a deep understanding of best practices:
- Intent, Not How: Comments should explain ‘why’ this piece of code does, not ‘how’ it’s doing it.
- Avoid Redundancy: Don’t assert the obvious. For example,
x = 2 # Assigning 2 to x
is unnecessary. - Keep it Concise: Comments should be short and meaningful. Avoid long narratives.
- Updating Comments: Always update comments when you update your code.
- Use Docstrings for Functions and Classes: Docstrings can provide valuable information about the purpose and usage of the function or class.
def add(a, b):
'''
Function to add two numbers.
Args:
a (int): The first number.
b (int): The second number.
Returns:
The sum of two numbers.
'''
return a + b
Common Pitfalls of Commenting in Python
While comments are highly beneficial, some pitfalls could lead to confusion and code readability:
- Wrong or Inconsistent Commenting: A wrong comment can lead to misunderstanding. It’s crucial to comment accurately. Moreover, being inconsistent in providing comments can add more confusion.
- Over Commenting: Overuse of comments can make the code messy. A good piece of code is mostly self-explanatory.
- Obsolete Comments: Code evolves, and so should the comments. It’s important to update comments as the code gets updated.
Conclusion
Effective commenting is an essential skill for professional Python developers. It eases debugging, enhances readability, and is instrumental for documentation. As a developer, one should strive for comments that accurately elucidate the ‘why’ rather than the ‘how’, avoid redundancy, and keep it concise. As we grow in our Python coding journey, the art of commenting is one of the unique subtleties we need to master for ourselves and other Pythonistas who cross our codebase.