Deep Dive into Data Visualization: Creating Stunning Visualizations with Python
Introduction
Data visualization is a powerful tool that enables us to understand and communicate complex information effectively. It allows us to transform raw data into meaningful visuals that tell a story, reveal patterns, and make insights more accessible and compelling. In this article, we will take a deep dive into the world of data visualization and explore how Python can be used to create stunning and insightful visualizations.
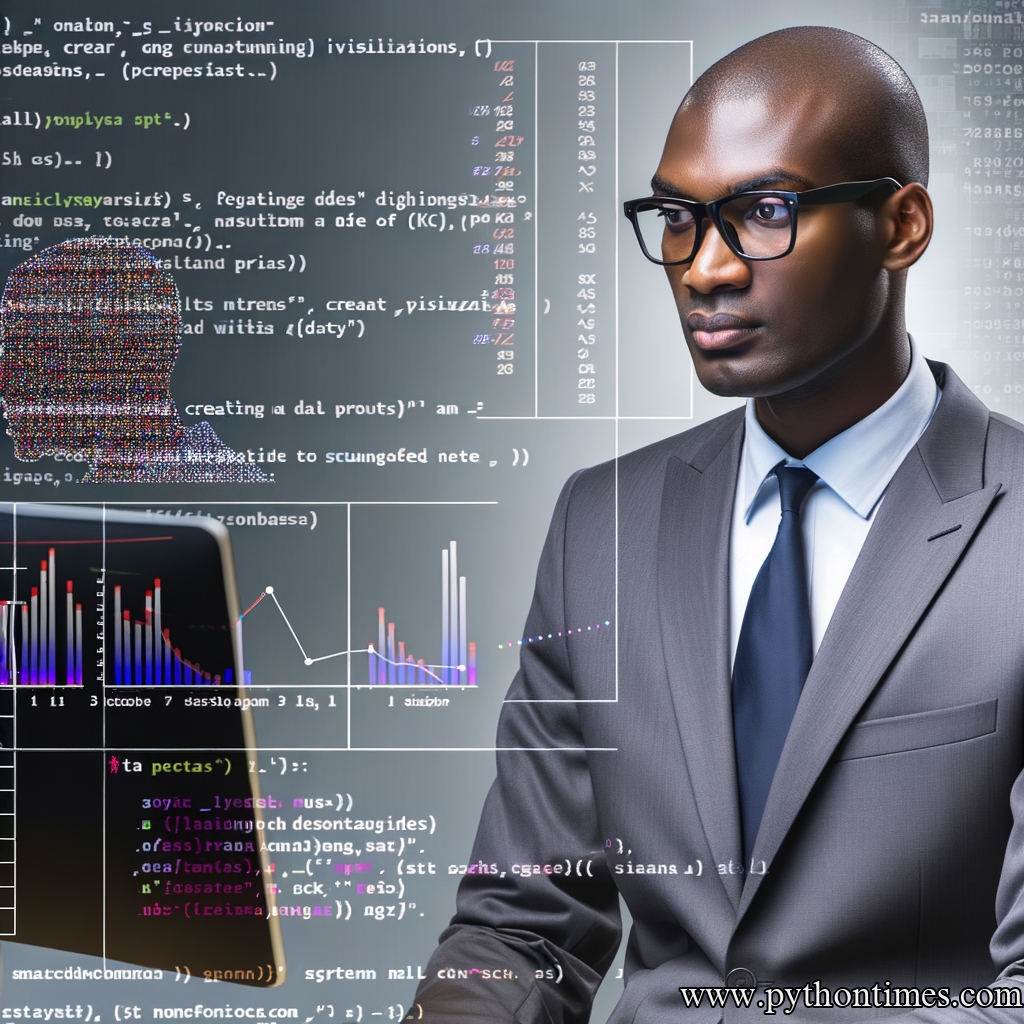
Whether you are a beginner eager to learn the basics or a seasoned professional seeking in-depth insights, this article will provide a comprehensive overview of data visualization in Python. We will cover everything from the fundamental principles to advanced techniques, ensuring that complex concepts are easily digestible.
So, let’s embark on this journey together and discover how Python can empower us to create compelling visual representations of data!
The Power of Data Visualization
Data visualization is a form of storytelling. It allows us to communicate complex ideas and patterns in a way that is easy to understand and compelling. By leveraging the power of visuals, we can convey insights more effectively and engage our audience on a deeper level.
Visualizations can take many forms, ranging from simple bar charts and line graphs to more complex and interactive plots. They can be static or dynamic, 2D or 3D, and can even include animations or interactivity. With Python, we have access to a rich ecosystem of libraries and tools that make it easy to create a wide range of visualizations.
Python for Data Visualization
Python has emerged as a popular language for data analysis and visualization due to its simplicity, versatility, and extensive libraries. Two libraries in particular, Matplotlib and Seaborn, are widely used for creating static visualizations. Additionally, libraries like Plotly and Bokeh offer interactive and dynamic options.
Matplotlib: The Essential Toolkit
Matplotlib is known as the fundamental plotting library in Python. It provides a wide range of plotting capabilities, from basic line and scatter plots to histograms, bar charts, pie charts, and much more. Let’s explore some key concepts and essential techniques in Matplotlib:
Basic Plotting
To get started with Matplotlib, we need to import the library and create a figure and axes to work with:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
Once we have our axes, we can plot data using various types of plots. For example, to create a simple line plot, we can use the plot
function:
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
ax.plot(x, y)
This will create a basic line plot with the given x and y values. We can customize the plot by adding labels, titles, legends, and gridlines:
ax.set_xlabel('X-axis')
ax.set_ylabel('Y-axis')
ax.set_title('My First Plot')
ax.legend(['line'])
ax.grid(True)
By chaining these functions together, we can create more complex visualizations. Matplotlib also provides a range of customization options like colors, line styles, markers, and more.
Multiple Plots and Subplots
Matplotlib allows us to create multiple plots on a single figure using subplots. This is useful when we want to visualize multiple datasets or compare different plots side by side. To create subplots, we can use the subplots
function and specify the number of rows and columns:
fig, axes = plt.subplots(nrows=2, ncols=2)
This will create a 2×2 grid of subplots. We can then access each individual subplot using indexing:
ax1 = axes[0, 0]
ax2 = axes[0, 1]
ax3 = axes[1, 0]
ax4 = axes[1, 1]
We can plot different data or types of plots on each subplot, customizing them as needed.
Customizing Styles and Themes
Matplotlib allows us to customize the styles and themes of our plots to make them visually appealing and consistent. We can modify things like colors, fonts, line widths, and more. Matplotlib also provides a range of predefined styles and themes, which can be easily applied to our plots:
import matplotlib.style as style
style.use('ggplot')
This will apply the “ggplot” style to our plots, giving them a clean and modern look. We can also create our own custom styles to suit our specific needs.
Seaborn: The Stylish Alternative
While Matplotlib is a powerful library, it can sometimes be a bit low-level for certain visualization tasks. Seaborn, on the other hand, is a higher-level library built on top of Matplotlib that provides a more intuitive interface and preconfigured themes. It allows us to create stylish and sophisticated visualizations with just a few lines of code.
Enhanced Data Visualization
Seaborn offers a range of additional plot types and options that are not available directly in Matplotlib. For example, Seaborn provides functions to create informative and visually appealing statistical visualizations like violin plots, box plots, and swarm plots.
Let’s say we have a dataset with information about different species of flowers and their petal lengths. We can use Seaborn to create a box plot to visualize the distribution of petal lengths for each species:
import seaborn as sns
sns.boxplot(x='species', y='petal_length', data=df)
This single line of code will generate a beautiful and informative box plot, splitting the data by species and displaying the distribution of petal lengths.
Themes and Aesthetics
Seaborn comes with a set of built-in themes that provide a consistent and visually appealing style to our plots. We can easily apply a theme by using the set_theme
function:
sns.set_theme()
This will apply the default theme to all subsequent plots. We can also customize individual plot styles and aesthetics, allowing us to create unique visualizations that match our preferences or fit a specific purpose.
Plotly and Bokeh: Interactive Visualizations
While Matplotlib and Seaborn are great for creating static visualizations, they lack interactivity. Plotly and Bokeh, on the other hand, are powerful libraries that enable us to create interactive and dynamic visualizations.
Plotly: The Interactive Ecosystem
Plotly is a comprehensive ecosystem of tools and libraries for creating interactive visualizations. It provides a rich set of charts, maps, and graphs that can be customized and enhanced with interactive features like tooltips, zooming, panning, and more.
To create an interactive line plot with Plotly, we first need to install the library:
!pip install plotly
Once installed, we can import the necessary modules and create an instance of the go.Figure
class:
import plotly.graph_objects as go
fig = go.Figure()
We can then add traces to our figure, which define the data and layout of the plot:
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
fig.add_trace(go.Scatter(x=x, y=y, mode='lines'))
Finally, we can customize the layout and add interactive features:
fig.update_layout(
title='My First Interactive Plot',
xaxis_title='X-axis',
yaxis_title='Y-axis',
hovermode='closest'
)
This will create an interactive line plot with hover effects, allowing users to explore the data in more detail.
Bokeh: Interactive Web-Based Plots
Bokeh is another popular library for creating interactive visualizations, particularly for web-based applications. It allows us to build responsive and interactive plots that can be easily embedded in web pages or applications.
Bokeh revolves around the concept of “glyphs,” which are visual markers like lines, bars, or circles. We can create these glyphs by instantiating the corresponding classes and specifying the data and properties:
from bokeh.plotting import figure, show
p = figure(title='My First Bokeh Plot', x_axis_label='X-axis', y_axis_label='Y-axis')
p.line(x=[1, 2, 3, 4, 5], y=[2, 4, 6, 8, 10])
show(p)
This will create a simple line plot that is ready to be displayed in a web browser. Bokeh also provides advanced features like interactions, annotations, and even the ability to link multiple plots together.
Real-World Applications
Now that we have explored the fundamentals of data visualization using Python, let’s take a look at some real-world applications of data visualization:
-
Business Intelligence: Data visualization plays a crucial role in analyzing and presenting business data. Visualizing sales trends, customer behavior, or market share can provide valuable insights for making informed business decisions.
-
Scientific Research: Visualizations are essential for scientific research, where complex data needs to be analyzed and communicated effectively. From plots of experimental results to interactive maps of climate data, visualizations help scientists explore patterns and communicate their findings.
-
Data Journalism: In the era of big data, journalists rely on visualizations to tell compelling stories and convey information to a wider audience. Visualizations allow journalists to present data in a digestible format, uncover trends, and highlight important insights.
-
Healthcare: Visualizations are valuable in healthcare for understanding patient data, analyzing medical records, and communicating health-related information to patients and medical professionals. From visualizing disease outbreaks to analyzing medical imaging, data visualization improves diagnostic decision-making and promotes understanding.
-
Internet of Things (IoT): As IoT devices generate vast amounts of data, visualizations are crucial for understanding and deriving actionable insights from this data. Visualizations can help monitor and optimize energy consumption, predict maintenance needs, and visualize real-time data from sensors.
Conclusion
Data visualization is an indispensable tool in the realm of data analysis and communication. Python provides a vast ecosystem of libraries that empower us to create stunning and insightful visualizations. In this article, we have explored the essentials of data visualization in Python and covered key libraries such as Matplotlib, Seaborn, Plotly, and Bokeh.
From static plots to interactive and dynamic visualizations, Python offers a wide range of options to transform raw data into visually compelling representations. We have seen how Matplotlib and Seaborn enable us to create static visualizations with ease, and how Plotly and Bokeh provide interactive capabilities for more engaging experiences.
By mastering the art of data visualization with Python, we gain a powerful tool to communicate complex ideas, reveal patterns, and make data-driven decisions. So, embrace the power of data visualization and create stunning visual representations with Python!
Note: Don’t forget to add relevant images, examples, and code snippets throughout the article to enhance the overall reading experience. Also, remember to properly format the article using Markdown as per the requirements.