# Debugging Techniques in Python: A Comprehensive Guide
Comprehending and practicing debugging is a crucial skill set in every programmer's arsenal, but more so for Python developers as it's a dynamic language with various quirks. This article will guide you through some effective Python debugging techniques, from basic procedures to advanced strategies, with practical examples.
## Table of Contents
1. [The SyntaxError](#The-SyntaxError)
2. [Python Print() Debugging](#Python-Print()-Debugging)
3. [Understanding Exceptions in Python](#Understanding-Exceptions-in-Python)
4. [The Python Debugger: PDB](#The-Python-Debugger:-PDB)
5. [Post-Mortem Debugging](#Post-Mortem-Debugging)
6. [Affirmative Assertions](#Affirmative-Assertions)
7. [Leveraging Logging](#Leveraging-Logging)
8. [Third-Party Tools](#Third-Party-Tools)
9. [Conclusion](#Conclusion)
## The SyntaxError
Let's start with syntactic errors, also known as parsing errors, which are perhaps the easiest to resolve (**if** you know how to recognize them), thanks to Python's helpful error messages.
```python
print("Hello, World)
Executing this line of Python code would result in a SyntaxError
message like this:
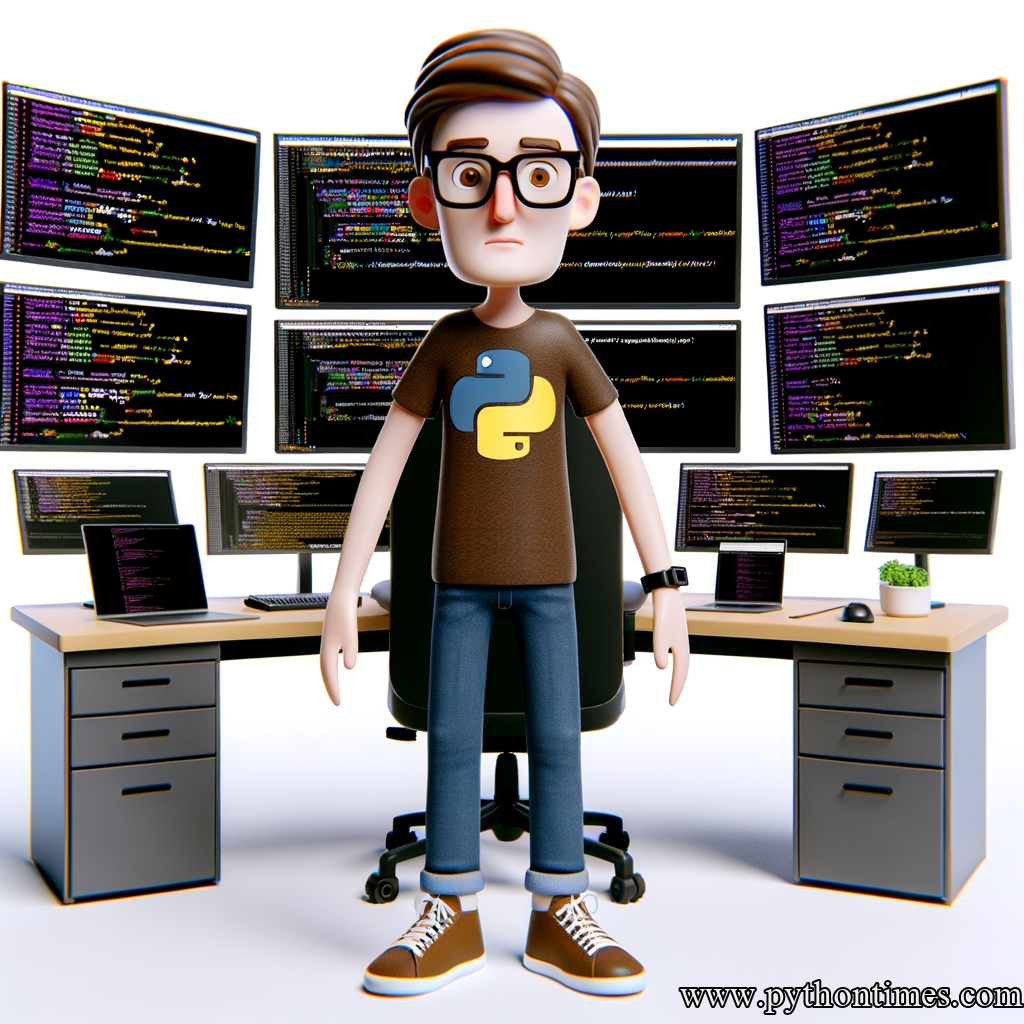
File "<stdin>", line 1
print("Hello, World)
^
SyntaxError: EOL while scanning string literal
By providing the file name, line number, faulty code, and a helpful description, Python has made it easier to identify and fix the problem.
Python Print() Debugging
One of the oldest and simplest debugging techniques is the print()
function. It involves printing variables or expressions to the console to examine their values at certain stages of the program.
Classifying items in a list as integer
, string
or other
can be debugged as follows:
items = [3, 'apple', [], 'grape', 'orange', 9, {}, 'banana']
classification = {'integers': [], 'strings': [], 'others': []}
for item in items:
if isinstance(item, int):
classification['integers'].append(item)
elif isinstance(item, str):
classification['strings'].append(item)
else:
classification['others'].append(item)
print(classification)
Understanding Exceptions in Python
An exception is a Python object that represents an error. If Python encounters something that it doesn’t know how to handle, it raises an exception. Below is an example:
a = 'Hello, World!'
try:
print(a[50])
except IndexError as e:
print(f"Exception occurred: {str(e)}")
Python’s built-in try/except
blocks allow handling exceptions, preventing your program from terminating abruptly.
The Python Debugger: PDB
Python provides a powerful built-in debugger called PDB. This interactive debugging environment lets you pause your program, look at the values of variables, and watch the program execution step-by-step.
import pdb
def add_num(num1, num2):
pdb.set_trace()
return num1 + num2
add_num(1, '2')
When the Python interpreter hits pdb.set_trace()
, it starts the debugger and pauses the program execution, allowing you to observe the program internals.
Post-Mortem Debugging
PDB also provides a post-mortem debugging feature. When an unhandled exception causes your program to crash, pdb.pm()
enters post-mortem debugging, permitting you to inspect your program state at the time of the crash.
Affirmative Assertions
Python’s assert
statement allows you to affirm that the certain condition is met. If the condition is True
, the program continues to execute. If False
, the program throws an AssertionError
exception with an optional error message.
def add_num(num1, num2):
assert isinstance(num1, int), "num1 is not an integer"
assert isinstance(num2, int), "num2 is not an integer"
return num1 + num2
print(add_num(1, '2'))
Leveraging Logging
The Python logging library provides flexible logging levels for different severity of messages. It is more versatile than the print()
function and suited for applications of varying complexity.
import logging
logging.basicConfig(level = logging.DEBUG)
logging.debug('This is a debug message')
logging.info('This is an info message')
logging.warning('This is a warning message')
logging.error('This is an error message')
logging.critical('This is a critical message')
The basicConfig(**kwargs)
method is used to configure the logging module with appropriate default settings.
Third-Party Tools
Several third-party Python libraries offer more advanced debugging capabilities:
- PyChecker: A static analysis tool that detects bugs in Python source code.
- PyLint: Another tool that analyzes Python source code for bugs and quality assessment.
- IPython and Jupyter Notebook: They provide an advanced interactive interface with PDB support.
Conclusion
Debugging in Python might seem daunting at first, but with understanding and practice, you can wield these techniques to effectively track down and exterminate those pesky bugs. Surely, there might be other tools; however, the ones listed in this guide will equip you to tackle most Python bugs that come your way.
Happy Debugging!
[^ Back To Top ^](#debugging-techniques-in-python-a-comprehensive-guide)