Creating and Publishing Python Packages
Python is a powerful programming language with an extensive library ecosystem. One of the key features that sets Python apart from other languages is its ability to create and distribute packages. Python packages allow you to bundle and distribute reusable code, making it easier for developers to share their work and for others to leverage existing solutions.
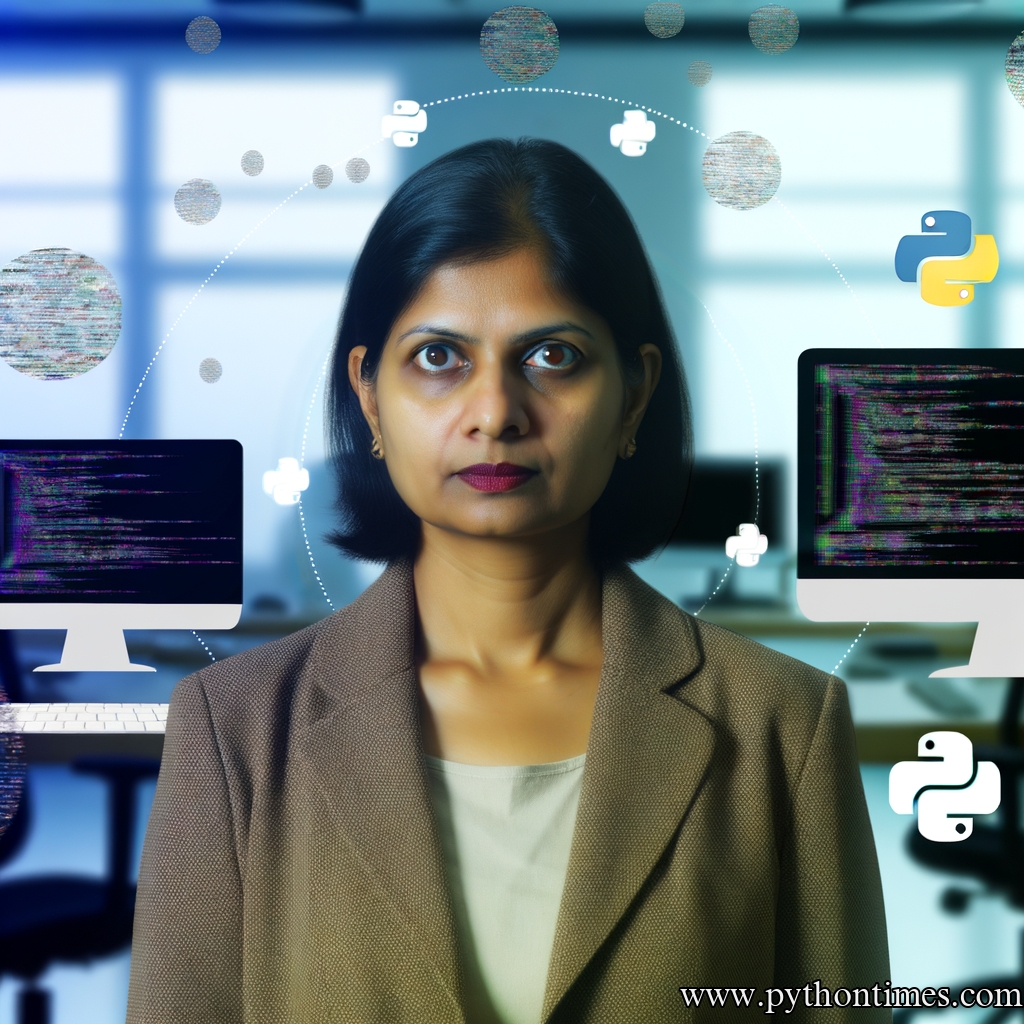
In this article, we will explore the process of creating and publishing Python packages. We will cover everything from setting up a package structure to publishing it on the Python Package Index (PyPI). Whether you are a beginner or an experienced Python programmer, this article will provide you with a comprehensive guide to creating and publishing your own Python packages.
Table of Contents
- Setting up the Package Structure
- Defining the Package Metadata
- Writing Package Code
- Testing the Package
- Documenting the Package
- Building and Distributing the Package
- Uploading the Package to PyPI
- Conclusion
1. Setting up the Package Structure
Before diving into writing package code, it is essential to set up the package structure correctly. The package structure will determine how your package is organized and how it can be imported by other Python programs.
A typical Python package structure consists of a main package directory and one or more subdirectories containing modules. To create your package structure, you can use the following commands:
$ mkdir mypackage
$ cd mypackage
$ touch __init__.py
The __init__.py
file serves as an indicator that this directory should be treated as a Python package. It can be an empty file or can contain initialization code for the package.
Next, you can create your modules inside the package directory or in subdirectories. A module is a Python file that contains code and defines functions, classes, or variables that can be imported and used by other programs. For example, you might create a module called utils.py
inside the package:
$ touch utils.py
Now that we have set up the basic package structure, let’s move on to defining the package metadata.
2. Defining the Package Metadata
To publish your package on PyPI, you need to provide some metadata information about your package. This information includes your package name, version number, author, description, and other relevant details.
The metadata can be defined in a file called setup.py
. This file should be placed in the root of your package directory. Here is an example of a setup.py
file:
from setuptools import setup
setup(
name='mypackage',
version='1.0.0',
author='John Doe',
author_email='john.doe@example.com',
description='A helpful package for Python developers',
url='https://github.com/johndoe/mypackage',
packages=['mypackage'],
classifiers=[
'Programming Language :: Python :: 3',
'License :: OSI Approved :: MIT License',
'Operating System :: OS Independent',
],
)
In this example, we are using the setuptools
library to define our package metadata. The name
field specifies the name of your package, the version
field indicates the package version, and the author
and author_email
fields provide information about the package author. The description
field gives a brief summary of your package, and the url
field specifies the URL where users can find more information about the package.
The packages
field is a list of all the packages (directories) that should be included in your distribution. In our case, we only have one package called mypackage
.
The classifiers
field is a list of classifiers that categorize your package. These classifiers help users find packages that meet specific criteria. For example, the classifier 'Programming Language :: Python :: 3'
indicates that your package is compatible with Python 3.
Once you have defined the package metadata, you can proceed to write the actual code for your package.
3. Writing Package Code
The code you write for your package depends on its purpose and functionality. In this section, we will provide some guidelines and best practices for writing package code.
3.1. Packaging Modules
Let’s assume we have a simple package called mypackage
, and it contains a module named utils.py
. This module provides some utility functions that can be used by other programs.
Inside utils.py
, we define two functions: add_numbers
and multiply_numbers
. Here is an example implementation:
def add_numbers(a, b):
return a + b
def multiply_numbers(a, b):
return a * b
To use these utility functions in another program, we need to import the module:
from mypackage import utils
print(utils.add_numbers(2, 3)) # Output: 5
print(utils.multiply_numbers(4, 5)) # Output: 20
By importing the utils
module from the mypackage
package, we can access the functions defined inside it.
3.2. Organizing Package Code
As your package grows, it is essential to organize your code to improve readability and maintainability. One common approach is to use subdirectories within the package directory.
Let’s say we want to add another module called math.py
to our mypackage
. We can create a new file math.py
inside mypackage
:
$ touch math.py
Next, we can define some math-related functions in math.py
. For example:
def calculate_average(numbers):
return sum(numbers) / len(numbers)
def calculate_median(numbers):
sorted_numbers = sorted(numbers)
if len(sorted_numbers) % 2 == 1:
return sorted_numbers[len(sorted_numbers) // 2]
else:
middle1 = sorted_numbers[len(sorted_numbers) // 2 - 1]
middle2 = sorted_numbers[len(sorted_numbers) // 2]
return (middle1 + middle2) / 2
To use the functions from the math
module, we need to import it:
from mypackage import math
numbers = [1, 2, 3, 4, 5]
print(math.calculate_average(numbers)) # Output: 3.0
print(math.calculate_median(numbers)) # Output: 3
By using subdirectories and splitting your code into multiple modules, you can keep your package organized and make it easier for others to understand and use.
Now that we have written our package code, let’s move on to testing the package.
4. Testing the Package
Testing is an essential part of the software development process. By writing tests for your package, you can ensure that it behaves as expected and that any future changes or additions do not introduce bugs or regressions.
In Python, the most commonly used testing framework is pytest
. To write tests for your package, you can create a separate directory called tests
inside your package directory. For example:
$ mkdir tests
Inside the tests
directory, you can create a test file for each module in your package. The test files should have the same name as the module they are testing, but with a test_
prefix.
Let’s create a test file for the math
module:
$ touch tests/test_math.py
In test_math.py
, we can define test functions using the pytest
framework. Here is an example of a test function that tests the calculate_average
function:
from mypackage import math
def test_calculate_average():
numbers = [1, 2, 3, 4, 5]
assert math.calculate_average(numbers) == 3.0
To run the tests, you can use the following command:
$ pytest
If all the tests pass, you should see an output similar to the following:
============================= test session starts ==============================
...
collected 1 item
tests/test_math.py . [100%]
============================== 1 passed in 0.01s ===============================
By writing comprehensive tests for your package, you can ensure its quality and reliability.
5. Documenting the Package
Documentation is crucial for helping users understand how to use your package effectively. It provides guidance on the package’s functionality, usage, and any additional considerations or requirements.
Python provides a built-in tool called pydoc
for generating documentation from docstrings. Docstrings are multi-line strings that document the purpose and usage of modules, classes, functions, and methods.
Let’s add some docstrings to the math
module. Here is an updated version of the module with docstrings:
def calculate_average(numbers):
"""
Calculates the average of a list of numbers.
Args:
numbers (list): A list of numbers.
Returns:
float: The average of the numbers.
Example:
>>> calculate_average([1, 2, 3, 4, 5])
3.0
"""
return sum(numbers) / len(numbers)
def calculate_median(numbers):
"""
Calculates the median of a list of numbers.
Args:
numbers (list): A list of numbers.
Returns:
float: The median of the numbers.
Example:
>>> calculate_median([1, 2, 3, 4, 5])
3
"""
sorted_numbers = sorted(numbers)
if len(sorted_numbers) % 2 == 1:
return sorted_numbers[len(sorted_numbers) // 2]
else:
middle1 = sorted_numbers[len(sorted_numbers) // 2 - 1]
middle2 = sorted_numbers[len(sorted_numbers) // 2]
return (middle1 + middle2) / 2
To generate documentation from the docstrings, you can use the following command:
$ pydoc -w mypackage
This command will generate HTML files for your package and its modules. Users can then open these files in a web browser to access the documentation.
Remember to keep your documentation up to date as you make changes to your package.
6. Building and Distributing the Package
Before you can publish your package on PyPI, you need to build a distribution package. A distribution package is a compressed archive (.tar.gz or .zip) that contains all the necessary files for installing and using your package.
To build a distribution package, you can use the setuptools
library and its sdist
command. From the root of your package directory, run the following command:
$ python setup.py sdist
This command will create a dist
directory inside your package directory. Inside the dist
directory, you will find the distribution package file.
It is also possible to build binary distribution packages using the bdist
command, which creates platform-specific distribution files. For more information on building different types of distribution packages, refer to the setuptools
documentation.
7. Uploading the Package to PyPI
Once you have built a distribution package, you are ready to upload it to PyPI. PyPI is the default package repository for Python packages and is widely used by the Python community.
To upload your package to PyPI, you first need to create an account. Once you have an account, you can use the twine
package to upload your package.
To install twine
, run the following command:
$ pip install twine
Once twine
is installed, you can upload your package using the following command:
$ twine upload dist/*
This command will prompt you to enter your PyPI username and password. After successful authentication, twine
will upload your package to PyPI.
Congratulations! You have now published your Python package on PyPI. Users can install your package using pip
by running the following command:
$ pip install mypackage
8. Conclusion
In this article, we explored the process of creating and publishing Python packages. We covered everything from setting up the package structure to uploading the package to PyPI. By following the steps outlined in this article, you can create and share your own Python packages with the wider Python community.
Remember to adhere to best practices, write comprehensive tests, and document your package effectively. By doing so, you can ensure the quality, usability, and maintainability of your package. Now go forth and create amazing Python packages!