Crash Course in Django: Rapid Development for Beginners
If you’re looking to dive headfirst into web development using Python, then Django is the framework you need. Django is a powerful and popular web development framework that simplifies and streamlines the process of building dynamic web applications. In this crash course, we will guide you through the essentials of Django, empowering you to create robust and scalable web applications in no time.
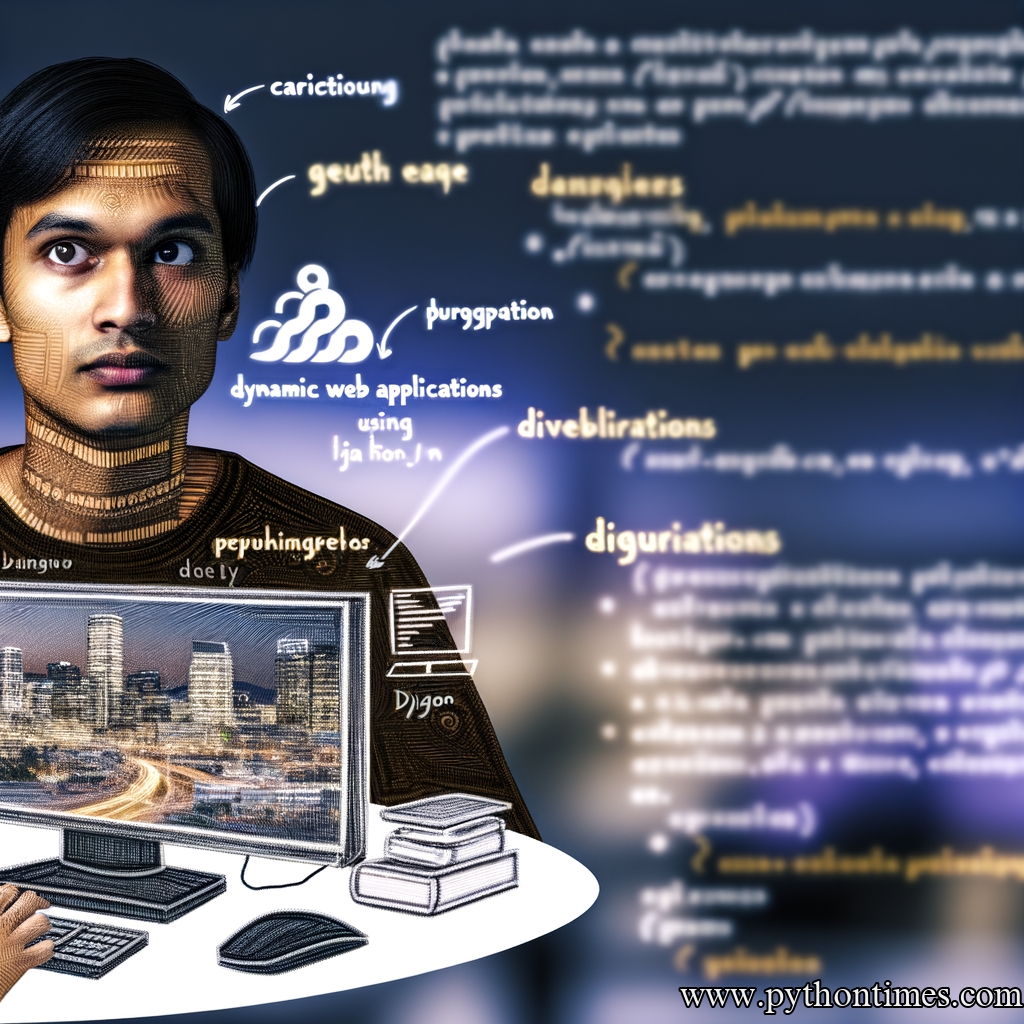
Why Django?
Before we jump into the nitty-gritty of Django, let’s take a quick moment to understand why it’s worth your time and effort. Django, as a high-level Python web framework, offers a plethora of advantages that make it a top choice for web developers:
- Rapid Development: Django follows the principle of “Don’t Repeat Yourself” (DRY), enabling you to focus on writing your application’s unique logic instead of boilerplate code.
- Batteries Included: Django comes bundled with a wide array of pre-built features and tools, including an ORM (Object-Relational Mapping) layer, authentication system, template engine, Admin interface, and much more. Say goodbye to manually integrating multiple libraries – Django has it covered.
- Scalability: Django is built with scalability in mind, allowing you to handle high traffic and large user bases effortlessly. Its architecture promotes modularity, making it easier to expand and maintain your application as it grows.
- Security: Django prioritizes security and incorporates numerous built-in safeguards to protect your application from common vulnerabilities, such as Cross-Site Scripting (XSS) and Cross-Site Request Forgery (CSRF).
- Community and Ecosystem: Django boasts a vibrant and supportive community, offering extensive documentation, numerous third-party packages, and a vast collection of tutorials and resources that make learning and troubleshooting a breeze.
Setting Up Your Development Environment
To get started with Django, you’ll need to set up your development environment. The following steps will guide you through the process:
-
Install Python: Ensure Python is installed on your machine. You can download the latest version of Python from the official Python website and follow the installation instructions based on your operating system.
-
Virtual Environments: It’s a best practice to work within virtual environments to keep your project dependencies isolated. Use the
venv
module to create a virtual environment:
Activate the virtual environment:
-
On Unix or Linux:
bash source myenv/bin/activate -
On Windows:
bash myenv\Scripts\activate -
Install Django: With the virtual environment active, use pip to install Django:
This will install the latest stable version of Django.
Creating Your First Django Project
With your development environment set up, it’s time to create your first Django project. Django comes with a command-line utility, django-admin
, that helps you kickstart your project. Let’s create a project named “myproject”:
django-admin startproject myproject
This will create a directory named “myproject” with the following structure:
myproject/
manage.py
myproject/
__init__.py
settings.py
urls.py
asgi.py
wsgi.py
manage.py
: The command-line utility that allows you to interact with your Django project.myproject/
: The root directory of your Django project.settings.py
: Configuration settings for your project.urls.py
: Defines the URL patterns for your project.asgi.py
andwsgi.py
: Entry points for ASGI and WSGI servers respectively.
To ensure everything is set up correctly, navigate to the project directory and run the development server:
cd myproject
python manage.py runserver
Congratulations! You’ve just launched your first Django project. Open your web browser and visit http://localhost:8000
. You should see the default Django welcome page.
Understanding the Django Architecture
To effectively work with Django, it’s essential to grasp its underlying architecture. Django follows the Model-View-Controller (MVC) architectural pattern, with a slight variation known as Model-View-Template (MVT).
-
Models: Models represent the structure and behavior of your data. Django’s ORM allows you to define models as Python classes, which are then mapped to database tables. Models define fields, relationships, and behaviors, providing a convenient way to interact with the database.
-
Views (Controllers in MVC): Views handle the logic behind processing and responding to HTTP requests. They retrieve data from the models, perform any necessary calculations or data transformations, and render templates to produce the final HTTP response.
-
Templates (Views in MVC): Templates define the presentation layer of your application. They are responsible for generating the HTML pages that will be sent back as responses. Django’s template engine provides filters, tags, and template inheritance, making it easy to build dynamic and reusable HTML templates.
-
URLs: URLs determine the mapping between URLs and views. In the
urls.py
file of your project, you define URL patterns, associating them with specific view functions or class-based views. This mechanism allows Django to route incoming requests to the corresponding views.
Now that you have a basic understanding of Django’s architecture let’s explore each component in more detail.
Models: Defining Your Data Structure
In Django, models serve as the heart of your application, providing an intuitive and efficient way to define and manage your data structure. Models are represented as Python classes, and each attribute within the class corresponds to a database field.
Let’s say we’re building a simple blog application, and we want to define a model for our blog posts. We start by creating a new file called models.py
in our application directory.
from django.db import models
class BlogPost(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
author = models.ForeignKey(
'Author',
on_delete=models.SET_NULL,
null=True
)
created_at = models.DateTimeField(auto_now_add=True)
In the above code snippet, we import the models
module from Django and create a class named BlogPost
that extends the models.Model
base class. We define four fields – title
, content
, author
, and created_at
– using various field types provided by Django’s ORM.
CharField
: Used for storing short strings.TextField
: Ideal for longer text content.ForeignKey
: Represents a one-to-many relationship with another model. In this case, we establish a relationship with theAuthor
model.DateTimeField
: Stores date and time values, automatically set when a new object is created.
Once you have defined your models, Django’s ORM takes care of generating the necessary SQL queries to create the corresponding database tables. To apply these changes, run the following command:
python manage.py makemigrations
This command creates a file in the migrations
directory of your app, which contains the instructions for creating the required tables. Finally, apply the changes by executing:
python manage.py migrate
That’s it! Your models are now ready to interact with the database.
Views: Handling HTTP Requests
Views are responsible for handling incoming HTTP requests, processing them, and generating appropriate responses. Django offers several ways to implement views, including function-based views and class-based views. Let’s start with function-based views.
In your views.py
file, import the required modules and define a function-based view to handle the blog post listing:
from django.shortcuts import render
from .models import BlogPost
def blog_post_list(request):
blog_posts = BlogPost.objects.all()
return render(request, 'blog/post_list.html', {'blog_posts': blog_posts})
The blog_post_list
view retrieves all blog posts from the database using the objects.all()
method and passes them to the render
function. The render
function takes the request
object, the template file name, and a context dictionary as arguments. The context dictionary contains the data that will be passed to the template for rendering.
To connect a URL to this view, open the urls.py
file in your project directory and add the following code:
from django.urls import path
from .views import blog_post_list
urlpatterns = [
path('posts/', blog_post_list, name='post_list'),
]
This urlpatterns
list maps the URL pattern 'posts/'
to the blog_post_list
view. Now, when you navigate to http://localhost:8000/posts/
, the view will be called, and the corresponding template will be rendered.
Templates: Building Dynamic Web Pages
Templates provide the HTML structure for your web application. Django’s template engine allows you to incorporate dynamic content and control structures into your templates, making them highly reusable and customizable.
Create a new directory called templates
within your application directory. Inside this directory, create a file named post_list.html
with the following content:
<h1>Blog Posts:</h1>
<ul>
{% for post in blog_posts %}
<li>{{ post.title }}</li>
{% empty %}
<li>No posts found.</li>
{% endfor %}
</ul>
In the above template, we use Django’s template language to iterate over the blog_posts
context variable passed from the view. If there are posts available, we display the title for each post. Otherwise, we display a default message.
By convention, Django will look for templates within the templates
directory, which is located in each app’s root directory. This separation ensures modularity and allows you to organize your templates efficiently.
URLs: Mapping URLs to Views
URL patterns define the mapping between the URLs of your website and the corresponding views that handle those URLs. The urls.py
file in your project directory serves as the main URL configuration module.
You’ve already seen how to add a simple URL pattern to your project. But what if you want to handle more complex URL patterns or include different applications within your project?
Django’s URL routing system allows you to achieve this easily. Let’s say you want to create a new Django app within your project to handle user authentication. You can generate this app using the following command:
python manage.py startapp accounts
Now, create a new file named urls.py
within the accounts
directory and include the following code:
from django.urls import path
from .views import login_view, logout_view, register_view
app_name = 'accounts'
urlpatterns = [
path('login/', login_view, name='login'),
path('logout/', logout_view, name='logout'),
path('register/', register_view, name='register'),
]
In this code snippet, we define three URL patterns – 'login/'
, 'logout/'
, and 'register/'
– and map them to their respective views. By setting the app_name
variable, we provide a namespace for these URLs, allowing you to refer to them within templates and other parts of your project without clashes.
To include these URLs in your main project, open the urls.py
file in your project directory and add the following code:
from django.contrib import admin
from django.urls import include, path
urlpatterns = [
path('admin/', admin.site.urls),
path('accounts/', include('accounts.urls')),
]
The include
function allows you to include the URLs defined in the accounts.urls
module under the 'accounts/'
prefix. Now, when you navigate to 'http://localhost:8000/accounts/login/'
, Django will find the appropriate view and render the corresponding template.
Extending Django’s Functionality: Middleware and Apps
Apart from the core components discussed so far, Django offers additional features that enhance its functionality and allow for seamless integration with third-party libraries.
Middleware: Customizing Request/Response Processing
Middleware in Django operates on the request/response processing pipeline, allowing you to customize the behavior of your application at various stages.
For instance, let’s say you want to add authentication to your application and ensure that only authenticated users can access certain URLs. Django provides built-in middleware, such as AuthenticationMiddleware
, that handles this for you.
To enable authentication, open the settings.py
file in your project directory and locate the MIDDLEWARE
setting. Add the following entry:
MIDDLEWARE = [
# ...
'django.contrib.auth.middleware.AuthenticationMiddleware',
# ...
]
With this middleware enabled, Django will automatically associate each incoming request with the User
object if the user is authenticated.
You can also create your own custom middleware to add specific functionality to your application. For example, let’s create a middleware that adds a custom HTTP header to each response:
class CustomHeaderMiddleware:
def __init__(self, get_response):
self.get_response = get_response
def __call__(self, request):
response = self.get_response(request)
response['X-Custom-Header'] = 'Hello from Django!'
return response
To activate your custom middleware, open the settings.py
file again, locate the MIDDLEWARE
setting, and add the following entry:
MIDDLEWARE = [
# ...
'myproject.middleware.CustomHeaderMiddleware',
# ...
]
Now, each response sent by your application will include the custom header X-Custom-Header
with the value 'Hello from Django!'
.
Apps: Extending Django Functionality
Django’s functionality can be extended by adding third-party apps or creating your own reusable apps. Django provides a wide range of pre-built apps that cover common use cases, such as user authentication, content management, and social media integration.
To include a third-party app in your project, you need to follow these steps:
- Install the app via pip. For example, to install Django’s provided authentication app, run:
- Open the
settings.py
file in your project directory and locate theINSTALLED_APPS
setting. Add the name of the app to the list:
With the authentication app included, you gain access to Django’s built-in authentication views, models, and other functionalities.
You can also create your own reusable apps to encapsulate specific functionalities that can be used across multiple projects. Django provides a helpful command to create a skeleton app structure:
python manage.py startapp myapp
Deployment and Beyond
As you continue your journey with Django, it’s important to explore the deployment options available for your projects. Django is compatible with various hosting platforms and provides comprehensive documentation on deployment procedures.
Additionally, to further enhance your Django skills, you can dive into advanced topics such as Django’s built-in administration interface, managing static files and media uploads, working with forms and form validation, implementing testing strategies, and integrating Django with frontend frameworks like React or Vue.js.
Django is an ever-evolving framework, and staying up to date with the latest releases and best practices is crucial. Attend Django meetups, participate in online forums, and explore the vast amount of resources available.
Remember, Django is just the beginning of your web development journey with Python. As you gain more experience, you can explore other frameworks (such as Flask or Pyramid) or different areas of Python development (such as data analysis, machine learning, or automation).
Conclusion
In this crash course, we’ve covered the essentials of Django, providing you with the foundational knowledge needed to build sophisticated web applications. We started by discussing the benefits of Django and setting up your development environment, followed by an exploration of Django’s architecture.
You learned how to define models to structure your data, create views to handle HTTP requests, and build templates to generate dynamic web pages. We also touched on URL routing, middleware, and extending Django’s functionality through apps.
Now that you have a solid understanding of Django, it’s time to roll up your sleeves and embark on your own Django development journey. With its extensive documentation, active community, and countless tutorials available, you’ll be well-equipped to tackle any web development challenges that come your way.
So, fire up your code editor, grab a cup of coffee, and let Django empower you to build amazing web applications with Python!