Code Optimization Techniques in Python
Python is a powerful and user-friendly programming language used by beginners and professionals alike. It’s much loved for its ease of learning and readability, which significantly reduces the cost of program maintenance. However, Python is not immune to performance issues. That’s where code optimization comes in. This article explores various Python code optimization techniques to help make your code efficient and effective.
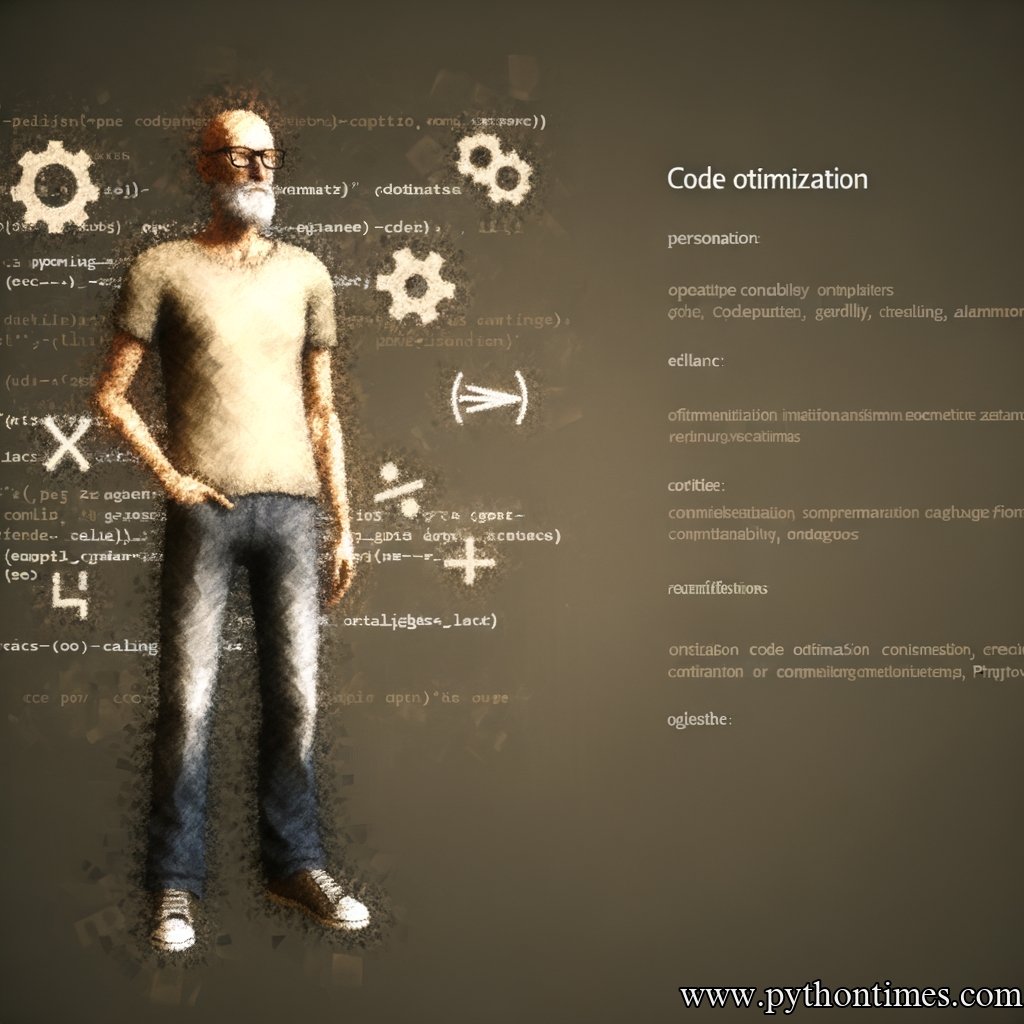
Table of Contents
- Understanding the Need for Code Optimization
- Efficient Use of Data Structures
- Code Profiling
- Cython, PyPy, and other Python implementations
- Using Built-in Functions and Libraries
- Python Code Tuning
- Practice ‘Lazy’ Computing
- Making Use of Local Variables
- Reducing Function Calls
- Loop Optimization
- Conclusion
Understanding the Need for Code Optimization
Before we delve into the techniques, it’s crucial to understand the need for Python code optimization. First, optimized code often translates to faster execution time. This could be significant in application performance and user experience. Second, it could minimize the resources required to run your code. Whether it’s CPU, memory, or storage, saving resources ultimately reduces operational costs. Finally, optimized code often means cleaner, more readable code, which always is a win in team settings.
Efficient Use of Data Structures
Python provides a rich set of data structures including lists, tuples, sets, and dictionaries. Using the right data structure for the right job can be a significant optimization technique. For example, a list comprehension is much faster than a for loop querying. Comparatively, the former is much faster as it specifically designed for the task.
# Using List Comprehension
squares = [x**2 for x in range(10)]
# Using for loop
squares = []
for x in range(10):
squares.append(x**2)
Code Profiling
Profiling is an essential Python optimization technique that helps identify bottlenecks in your code. Built-in Python modules like ‘cProfile’, ‘profile’ or ‘timeit’ generate complete reports of the most time-consuming parts of your code. By using these insights, you can effectively focus on the areas that need improvement.
import cProfile
def my_function():
… # your code
cProfile.run('my_function()')
Cython, PyPy and other Python Implementations
Python is an interpreted language, known for its ease of use and readability but not particularly for speed. However, alternatives such as Cython or PyPy can provide speedups. Cython is an optimizing static compiler for Python. It translates Python code into C and makes direct C-level API calls into the Python interpreter. PyPy, on the other hand, is a Python interpreter and just-in-time compiler which aims to provide better performance and memory usage over standard Python.
Using Built-in Functions and Libraries
Python has a wide range of built-in functions that are optimized and generally run faster than custom codes. It’s advisable to always check if Python has built-in functionalities for what you’re trying to achieve. Additionally, reliable libraries, such as NumPy or SciPy, offer many mathematical and scientific calculations that are optimized and faster than standard Python codes.
Python Code Tuning
Code tuning refers to modifying your code to run more efficiently. Small changes like using ‘local’ variables instead of ‘global’ variables, using the ‘in’ operator instead of a function call, or making use of ‘generator’ instead of ‘list’ can make drastic changes.
Time complexity aspects of the code also need to be reviewed. You should write your algorithms in a way that allows the code to execute faster – for instance, using ‘quick sorts’ instead of ‘bubble sorts’ can have a significant impact.
Practice ‘Lazy’ Computing
In Python, ‘lazy’ computing implies avoiding unnecessary computations, and it should be a general practice to enhance performance. You can utilize Python’s generator functions and the ‘yield’ keyword for this. They allow you to create ‘iterators’ with ease where computation occurs on-the-go, hence saving memory.
# A simple generator function
def my_generator():
for i in range(10):
yield i
Making Use of Local Variables
In Python, local variables are faster than global variables. So, if a value is used within a function, it’s more efficient to declare that value inside the function. Calling it from the global scope can slow down the program since Python takes additional time to access global variables.
Reducing Function Calls
Function calls in Python are expensive. They require a setup, argument passing, and a return evaluation. To optimize this, you can replace frequently used function calls with in-line code. For small and frequent routines, using inner functions can also speed up the program.
Loop Optimization
For loops in Python can be slow. However, if used correctly, they can be equally fast. Here are a few tactics to optimize loops:
- Avoid Looping: Whenever possible, avoid looping. Use Python built-in functions and comprehensions which are optimally written and generally faster.
- Loops Unrolling: If you constantly loop over a known number of elements, unroll the loop and place the code line for each iteration.
# Original loop
for i in range(5):
print(i)
# Loop unrolled
print(0)
print(1)
print(2)
print(3)
print(4)
- Eliminate Loop Invariants: If a loop does the same thing repeatedly, you should shift that outside the loop.
# Invariants inside loop
for i in range(n):
x = y ** 2 # This is the invariant
print(i * x)
# Invariant eliminated
x = y ** 2 # Shifted outside the loop
for i in range(n):
print(i * x)
Conclusion
There you have it. Code optimization in Python is a vast and essential aspect of Python programming, starting with efficient data structure usage, profiling, alternate Python solutions, using built-in libraries, code tuning, and loop optimization. Following these strategies, you can make your Python programs perform strongly and effectively. However, always remember Donald Knuth’s words, “Premature optimization is the root of all evil.” Write a working program first, then optimize it. Happy coding!