Building Serverless Web Applications with Python and AWS Lambda
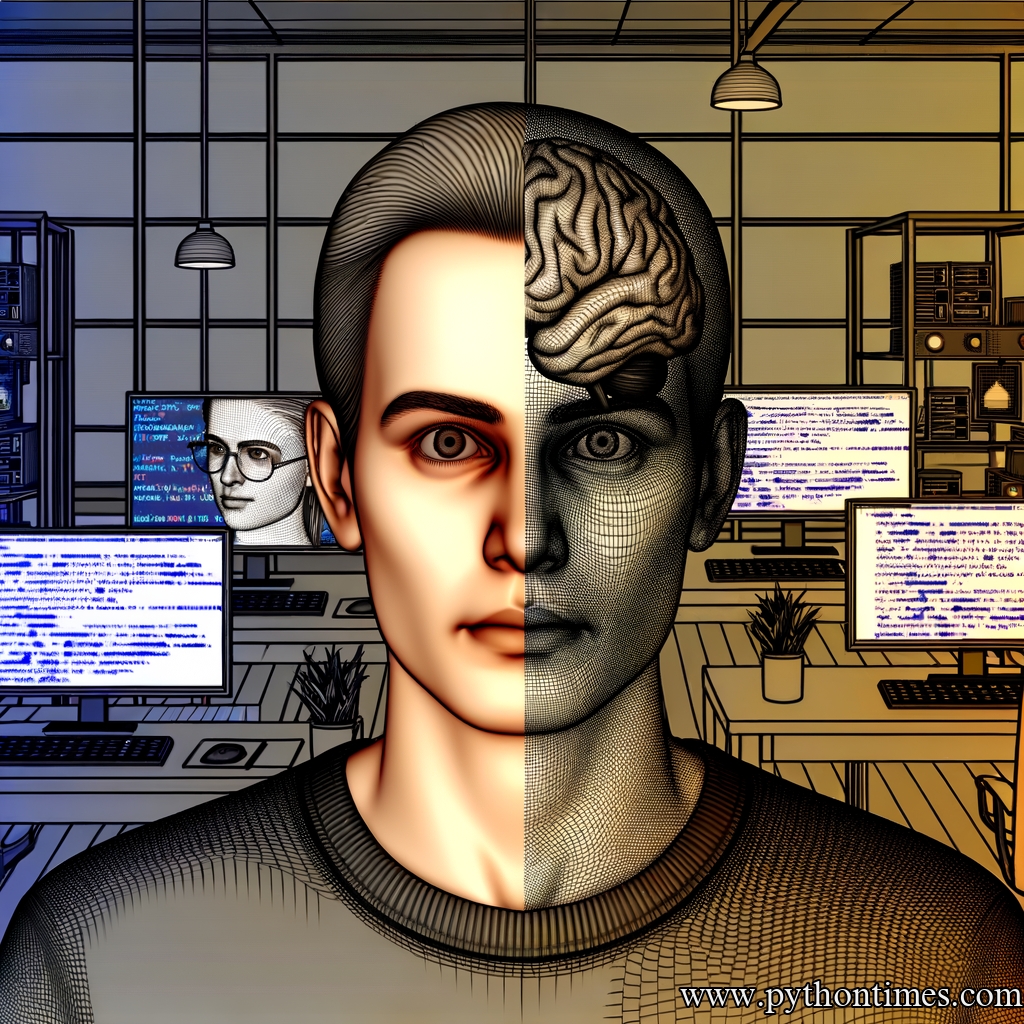
Welcome to PythonTimes.com, your leading hub for all things related to Python programming. In this article, we will dive into the exciting world of building serverless web applications using Python and AWS Lambda. Whether you are a beginner just starting your Python journey or a seasoned professional seeking in-depth insights into this topic, we will provide you with accessible yet informative content that will leave you feeling inspired and ready to embark on your own serverless ventures.
Introduction to Serverless Architecture
Before we delve into the specifics of building serverless web applications with Python and AWS Lambda, let’s take a moment to understand the concept of serverless architecture itself. Traditional web applications usually require managing servers, provisioning resources, and handling various infrastructure-related tasks. However, with serverless architecture, developers can focus solely on writing code without having to worry about server management and resource allocation.
In a serverless environment, your code is divided into small, self-contained functions known as “serverless functions.” These functions are executed only when triggered by an event, such as an HTTP request or a scheduled task. AWS Lambda, a serverless computing service provided by Amazon Web Services (AWS), allows you to run your serverless functions in a highly scalable and cost-effective manner.
Why Python and AWS Lambda?
Python, known for its simplicity and readability, is an excellent choice for serverless web application development. Its extensive standard library and broad community support make it a versatile language for building a wide range of applications.
AWS Lambda, on the other hand, provides a scalable and reliable infrastructure for running serverless functions. With its pay-per-use pricing model, you only pay for the computing resources you consume during the execution of your functions. This allows you to optimize costs and scale your applications seamlessly without worrying about infrastructure management.
Setting Up Your Development Environment
Before we start building serverless web applications, let’s ensure that our development environment is properly configured. Here are the steps to set up your environment:
-
Install Python: If you haven’t already, download and install Python from the official Python website (https://www.python.org). Make sure to choose the latest stable version compatible with your operating system.
-
Install AWS CLI: The AWS Command Line Interface (CLI) allows you to interact with various AWS services, including AWS Lambda. You can install the AWS CLI by following the instructions in the official AWS CLI documentation (https://aws.amazon.com/cli/).
-
Configure AWS CLI: After installing the AWS CLI, run the
aws configure
command in your terminal to configure your credentials. You will need an AWS access key ID and secret access key, which you can obtain from your AWS Management Console. -
Install Python Packages: Depending on the specific requirements of your serverless web application, you may need to install additional Python packages. Use the
pip
command to install necessary packages, ensuring compatibility with AWS Lambda.
With your development environment set up, we can now move on to building serverless web applications.
Creating Your First Serverless Function
To get started, let’s create a simple serverless function using Python and AWS Lambda. We will build a function that takes two numbers as input and returns their sum. Follow along with the steps below:
-
Create a New Directory: Open your terminal and navigate to the directory where you want to create your serverless function. Use the
mkdir
command to create a new directory. -
Initialize a Python Virtual Environment: It is best practice to work within a virtual environment to keep your dependencies isolated. Use the
python -m venv myenv
command to create a new virtual environment named “myenv.” -
Activate the Virtual Environment: Activate the virtual environment using the appropriate command for your operating system. On Unix or Linux-based systems, use the
source myenv/bin/activate
command. On Windows, use themyenv\Scripts\activate
command. -
Install the
boto3
Package: Boto3 is the AWS SDK for Python, which allows us to interact with AWS services, including AWS Lambda. Install theboto3
package using thepip install boto3
command. -
Create a New Python File: Use your preferred text editor to create a new Python file within your project directory. Let’s name it
sum_function.py
.
- Deploy the Function to AWS Lambda: In your terminal, use the AWS CLI to deploy your function to AWS Lambda. Run the following command:
In this command, replace YOUR_ACCOUNT_ID
with your actual AWS account ID. Additionally, make sure to update the --role
option with the ARN of the IAM role you want to use (replace lambda-role
with your actual role name). This role should have the necessary permissions to execute Lambda functions.
- Invoke the Function: Once the function is deployed, you can invoke it using the AWS CLI or the AWS Lambda console. For example:
The above command will invoke the sumFunction
with the payload {"num1": 5, "num2": 3}
and store the output in the output.json
file.
Congratulations! You have successfully created and deployed your first serverless function using Python and AWS Lambda. This simple example demonstrates the power and flexibility of serverless architecture.
Handling HTTP Requests with AWS API Gateway
While the previous example showcased invoking a serverless function directly, it is common for serverless web applications to receive HTTP requests. To handle HTTP requests, we can integrate our serverless functions with AWS API Gateway.
AWS API Gateway is a fully managed service that makes it easy to create, publish, and manage APIs at scale. It serves as the entry point for your serverless web application, routing HTTP requests to the appropriate Lambda functions.
Let’s extend our previous example to handle an HTTP request:
- Create a New Serverless Function: Within your project directory, create a new Python file named
http_function.py
and define the following function:
In this example, we retrieve the numbers to be summed from the query string parameters of the HTTP request and return the result as a JSON response.
- Create an API Gateway Endpoint: To create an API Gateway endpoint that triggers our serverless function, use the AWS CLI to define the API Gateway resource structure. Run the following command:
This command creates a new API with the name myApi
and sets the target to our sumFunction
.
- Create an Integration: Once the API is created, we need to configure an integration between our API Gateway and our Lambda function. Run the following command:
Make sure to replace YOUR_API_ID
, REGION
, and YOUR_ACCOUNT_ID
with the appropriate values.
- Create an Integration Response: Next, create an integration response to handle the Lambda function’s response. Run the following command:
Replace YOUR_API_ID
and YOUR_INTEGRATION_ID
with the appropriate values.
- Create a Route: Finally, create a route that maps incoming HTTP requests to the integration. Run the following command:
Replace YOUR_API_ID
and YOUR_INTEGRATION_ID
with the appropriate values.
Once the above steps are complete, you will have an API Gateway endpoint that triggers your serverless function in response to incoming HTTP requests. You can use tools like cURL or Postman to test your endpoint by sending HTTP requests with the appropriate query string parameters.
Real-World Applications of Serverless Web Applications
Serverless web applications have gained immense popularity due to their scalability, cost-effectiveness, and ease of development. Here are some real-world applications where serverless web applications shine:
1. Microservices Architectures
Serverless functions are a perfect fit for microservices architectures. Each microservice can be implemented as a separate serverless function, allowing teams to develop, deploy, and maintain services independently. This enables faster development cycles and greater flexibility in provisioning resources.
2. Event-Driven Processing
Serverless architecture is ideal for event-driven processing. With AWS Lambda, you can configure your serverless functions to automatically trigger in response to events such as file uploads, database changes, or scheduled tasks. This makes it easy to build data pipelines, process logs, or perform periodic maintenance tasks.
3. Chatbots and Voice Assistants
Serverless functions can power chatbots and voice assistants, allowing you to build intelligent conversational interfaces. By integrating serverless functions with natural language processing services like Amazon Lex or Dialogflow, you can create interactive bots that can understand and respond to user queries.
4. Webhooks and API Proxies
Serverless functions can act as webhook endpoints, receiving and processing data from external services. They can also serve as API proxies, allowing you to add authentication, caching, or monitoring layers to your existing APIs. This enables you to extend the functionality of your applications without modifying the underlying services.
5. Serverless Websites
With the advent of technologies like AWS Amplify and AWS S3, it is now possible to build entire websites using serverless architecture. These websites can benefit from auto-scaling, high availability, and pay-per-use pricing models. Whether you are building a personal blog or a company website, serverless architecture can provide a scalable and cost-effective solution.
Serverless web applications have a wide range of applications beyond what we’ve covered here. As you explore this technology further, you will discover even more possibilities and creative use cases that leverage the power of serverless computing.
Best Practices and Considerations
As you dive deeper into building serverless web applications with Python and AWS Lambda, keep the following best practices and considerations in mind:
-
Proper Error Handling: Ensure that your serverless functions handle errors gracefully and provide appropriate error messages. This will help you diagnose and troubleshoot issues effectively.
-
Resource Consumption: Keep an eye on your function’s resource consumption, including memory usage and execution time. Optimizing resource usage can help you manage costs more effectively.
-
Secret Management: Safely manage sensitive information such as API keys, database credentials, and other secrets. AWS provides services like AWS Secrets Manager and AWS Parameter Store to securely store and retrieve secrets in your serverless functions.
-
Testing and Debugging: Implement a robust testing and debugging strategy to catch any issues before deploying your functions to production. Tools like AWS SAM Local and the
pytest
framework can help you write unit tests and simulate AWS services locally. -
Monitoring and Logging: Configure appropriate monitoring and logging to gain insights into the performance and behavior of your serverless functions. Services like AWS CloudWatch can help you collect and analyze logs, set up alarms, and monitor important metrics.
By following these best practices and taking these considerations into account, you can ensure that your serverless web applications are scalable, resilient, and secure.
Conclusion
In this article, we have explored the exciting world of building serverless web applications with Python and AWS Lambda. We started by understanding the concept of serverless architecture and its benefits. We then set up our development environment and created our first serverless function using Python and AWS Lambda. We also learned how to handle HTTP requests using AWS API Gateway and integrated it with our serverless functions. Finally, we discussed real-world applications and best practices to consider when building serverless web applications.
Remember, serverless architecture is a powerful and flexible paradigm for building web applications, and Python, in conjunction with AWS Lambda, offers a seamless development experience. Whether you are a beginner just starting your Python journey or a seasoned professional, I encourage you to explore the possibilities of building serverless web applications with Python and AWS Lambda. Happy building!