Building a Twitter Bot with Tweepy
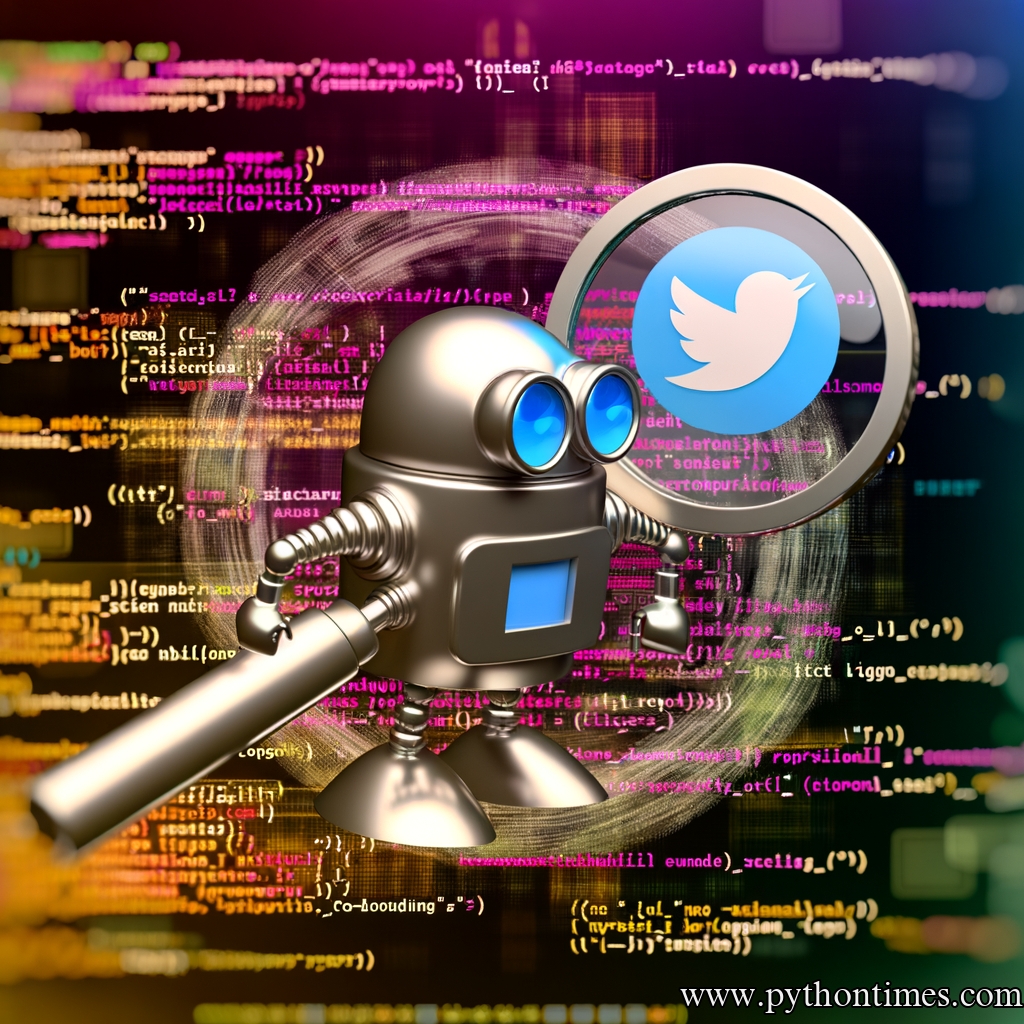
Introduction
Twitter has become a prominent social media platform with millions of users worldwide. With its vast user base, Twitter offers great potential for businesses, developers, and individuals to reach a wide audience and engage with their followers. One way to automate Twitter interactions and boost productivity is by creating a Twitter bot.
In this tutorial, we will explore how to build a Twitter bot using Tweepy – a Python library for accessing the Twitter API. We will cover the basics of Tweepy, setting up authentication, retrieving data from Twitter, posting tweets, and implementing various functionalities to make our Twitter bot interactive and useful.
Table of Contents
- What is a Twitter Bot?
- Installing Tweepy
- Prerequisites
- Installing Tweepy
- Setting up Twitter API Access
- Creating a Twitter Developer Account
- Creating a Twitter App
- Generating Access Tokens
- Authenticating with Tweepy
- Retrieving Tweets
- Posting Tweets
- Posting Text Tweets
- Posting Tweets with Media
- Interacting with Users
- Liking and Retweeting Tweets
- Following Users
- Sending Direct Messages
- Implementing Advanced Functionalities
- Searching for Tweets
- Analyzing Sentiment of Tweets
- Monitoring for Mentions and Replies
- Best Practices and Tips for Building a Twitter Bot
- Conclusion
1. What is a Twitter Bot?
Before we dive into building a Twitter bot, let’s start by understanding what exactly a Twitter bot is. A Twitter bot is a program or script that automates actions on the Twitter platform. It can perform a variety of tasks such as posting tweets, liking and retweeting tweets, following specific users, sending direct messages, and even analyzing sentiments of tweets.
Twitter bots can be used for various purposes, ranging from automating repetitive tasks to creating interactive chatbots and providing real-time updates. They can be customized to serve specific needs, and their functionality is limited only by the imagination of the developer.
2. Installing Tweepy
Prerequisites
To get started with building a Twitter bot using Tweepy, you’ll need the following:
- Python installed on your system (preferably Python 3.7 or later)
- A Twitter Developer Account
- Access to the Internet
Installing Tweepy
Once you have Python set up, installing Tweepy is a breeze. Open a command prompt or terminal and run the following command:
pip install tweepy
This command will download and install the latest version of Tweepy from the Python Package Index (PyPI).
3. Setting up Twitter API Access
In order to use Tweepy to interact with the Twitter API, we need to set up a Twitter Developer Account and create a Twitter App to obtain the necessary API keys and tokens.
Creating a Twitter Developer Account
To create a Twitter Developer Account, follow these steps:
- Go to the Twitter Developer Portal.
- Click on the “Apply” button to start the application process.
- Fill out the required information, including your username, phone number, and a brief explanation of how you plan to use the Twitter API.
- Read and accept the terms and conditions, then submit the application.
After submitting the application, Twitter will review your application and notify you via email regarding the status of your request. Once approved, you can proceed to the next step.
Creating a Twitter App
To create a Twitter App, follow these steps:
- Log in to your Twitter Developer Account.
- Go to the Twitter Developer Portal.
- Click on “Projects & Apps” in the navigation menu.
- Click on the “Create App” button.
- Fill out the required information, including the name, description, and website URL of your app.
- Read and accept the terms and conditions, then click on the “Create” button.
Once you’ve created your app, you will be redirected to the app’s details page. Here, you can find your API keys and tokens, which we will need for authentication.
Generating Access Tokens
To generate access tokens for your Twitter app, follow these steps:
- Go to the app’s details page in the Twitter Developer Portal.
- Click on the “Keys and tokens” tab.
- Scroll down to the “Access token & access token secret” section.
- Click on the “Generate” button to generate your access tokens.
Make sure to keep your API keys and tokens private and don’t share them with anyone. These credentials provide access to your Twitter account and must be kept confidential.
4. Authenticating with Tweepy
Before we can start interacting with the Twitter API using Tweepy, we need to authenticate ourselves using the API keys and tokens we obtained in the previous step.
import tweepy
consumer_key = "YOUR_CONSUMER_KEY"
consumer_secret = "YOUR_CONSUMER_SECRET"
access_token = "YOUR_ACCESS_TOKEN"
access_token_secret = "YOUR_ACCESS_TOKEN_SECRET"
auth = tweepy.OAuthHandler(consumer_key, consumer_secret)
auth.set_access_token(access_token, access_token_secret)
api = tweepy.API(auth)
In this code snippet, replace YOUR_CONSUMER_KEY
, YOUR_CONSUMER_SECRET
, YOUR_ACCESS_TOKEN
, and YOUR_ACCESS_TOKEN_SECRET
with your own credentials.
Once authenticated, we can access the Twitter API using the api
object, which provides various methods for interacting with Twitter.
5. Retrieving Tweets
One of the main purposes of a Twitter bot is to retrieve tweets from the platform. Tweepy provides several methods for retrieving tweets, including retrieving your own tweets, retrieving tweets from a specific user, and searching for tweets based on specific keywords.
Retrieving Your Own Tweets
To retrieve your own tweets, you can use the user_timeline()
method of the api
object.
tweets = api.user_timeline()
By default, this method will retrieve the 20 most recent tweets from your own timeline. You can also pass additional parameters to customize the retrieval, such as the number of tweets to retrieve and whether to include retweets.
tweets = api.user_timeline(count=50, include_rts=False)
This example retrieves the 50 most recent tweets from your timeline, excluding retweets.
Retrieving Tweets from a Specific User
To retrieve tweets from a specific user, you can use the user_timeline()
method and provide the screen_name
parameter with the username of the user whose tweets you want to retrieve.
tweets = api.user_timeline(screen_name="realDonaldTrump")
This example retrieves the 20 most recent tweets from the user with the username “realDonaldTrump”. You can also customize the retrieval by passing additional parameters, such as the number of tweets to retrieve and whether to include retweets.
tweets = api.user_timeline(screen_name="realDonaldTrump", count=50, include_rts=False)
Searching for Tweets
To search for tweets based on specific keywords, you can use the search()
method of the api
object.
tweets = api.search(q="Python", result_type="recent", lang="en", count=100)
This example searches for the most recent 100 tweets that contain the word “Python” and are written in English. By default, the search()
method retrieves a maximum of 15 tweets per request, but you can retrieve more by using pagination.
tweets = tweepy.Cursor(api.search, q="Python", result_type="recent", lang="en").items(200)
This example uses the Cursor
class to retrieve 200 tweets instead of the default limit of 15 tweets per request.
6. Posting Tweets
Now that we know how to retrieve tweets, let’s move on to posting tweets using Tweepy. To post a tweet, we can use the update_status()
method of the api
object.
Posting Text Tweets
To post a text tweet, simply pass the tweet text as a string to the update_status()
method.
api.update_status("Hello, Twitter! This is my first tweet using Tweepy. #TwitterBot")
This code will post a tweet with the specified text. You can include hashtags, mentions, and URLs in your tweets as well.
Posting Tweets with Media
Tweepy also supports posting tweets with media, such as images. To post a tweet with media, we need to upload the media file first and then pass the media file’s media_id
to the update_status()
method.
media = api.media_upload("image.jpg")
api.update_status("Check out this cool image!", media_ids=[media.media_id])
In this example, we upload an image file named “image.jpg” and then post a tweet with the text “Check out this cool image!” along with the uploaded image attached to the tweet.
7. Interacting with Users
A Twitter bot can also interact with other users by performing actions like liking and retweeting tweets, following users, and sending direct messages.
Liking and Retweeting Tweets
To like a tweet, we can use the create_favorite()
method of the api
object and pass the tweet’s ID.
api.create_favorite(tweet_id)
To retweet a tweet, we can use the retweet()
method of the api
object and pass the tweet’s ID.
api.retweet(tweet_id)
Replace tweet_id
with the actual ID of the tweet you want to like or retweet.
Following Users
To follow a user, we can use the create_friendship()
method of the api
object and pass the username or ID of the user.
api.create_friendship(screen_name="PythonTimes")
This example follows the user with the username “PythonTimes”. You can also pass the user’s ID instead of the username.
api.create_friendship(user_id="12345678")
Sending Direct Messages
To send a direct message to a user, we can use the send_direct_message()
method of the api
object and pass the recipient’s username or ID and the message text.
api.send_direct_message(screen_name="PythonTimes", text="Hello!")
This example sends a direct message with the text “Hello!” to the user with the username “PythonTimes”. You can also pass the recipient’s ID instead of the username.
api.send_direct_message(user_id="12345678", text="Hello!")
8. Implementing Advanced Functionalities
Now that we have covered the basics of building a Twitter bot with Tweepy, let’s explore some advanced functionalities to make our bot more powerful and feature-rich.
Searching for Tweets
Tweepy provides a rich set of methods for searching tweets based on various criteria. We have already seen the search()
method, but Tweepy also provides methods for searching tweets within a specific timeframe, tweets from a specific location, and even tweets from a specific user.
Analyzing Sentiment of Tweets
To analyze the sentiment of tweets, we can use natural language processing (NLP) libraries such as NLTK or TextBlob in combination with Tweepy. These libraries can help us determine whether a tweet is positive, negative, or neutral based on its content.
Monitoring for Mentions and Replies
To monitor for mentions and replies to our Twitter bot, we can use the user_timeline()
method with the mentions_timeline
parameter. This method retrieves tweets that mention or reply to our bot’s account.
mentions = api.mentions_timeline()
We can then iterate over the retrieved tweets and perform actions such as liking, retweeting, or replying to the mentions and replies.
9. Best Practices and Tips for Building a Twitter Bot
When building a Twitter bot, it’s important to follow best practices to ensure its effectiveness, reliability, and compliance with Twitter’s terms of service. Here are some tips to keep in mind:
- Respect Twitter’s API rate limits to avoid temporary or permanent suspension of your bot’s access to the API.
- Be mindful of the potential impact of your bot’s activities on other users and ensure it operates within acceptable limits.
- Use proper error handling to handle exceptions and gracefully handle any potential issues or failures.
- Regularly monitor your bot’s activities and response to ensure its proper functioning.
- Consider adding safeguards to prevent the bot from engaging in malicious or harmful activities.
- Respect user privacy and data protection regulations when implementing functionalities that involve user data.
Conclusion
In this tutorial, we have explored how to build a Twitter bot using Tweepy. We covered the basics of Tweepy, setting up authentication, retrieving data from Twitter, posting tweets, and implementing various functionalities to make our Twitter bot interactive and useful. With Tweepy, the possibilities are endless, and you can create a bot tailored to your specific needs.
As you continue to explore Tweepy and the Twitter API, remember to adhere to Twitter’s guidelines, stay updated with changes to the API, and continuously iterate on your bot’s functionality to provide the best experience for your users. Happy coding!
References: – Tweepy Documentation – Twitter Developer Portal