Best Practices for Writing Python Code
The art of coding is similar to penning down a beautiful novel – coherent, concise, and captivating. In Python, this is facilitated by clear syntactic conventions and style guides. As one delves deeper into the programming ecosystem, understanding these best practices can boost code readability and maintenance drastically. This article provides a comprehensive set of recommendations for both novices and veterans of Python, accompanied by practical examples to reshape your coding graph.
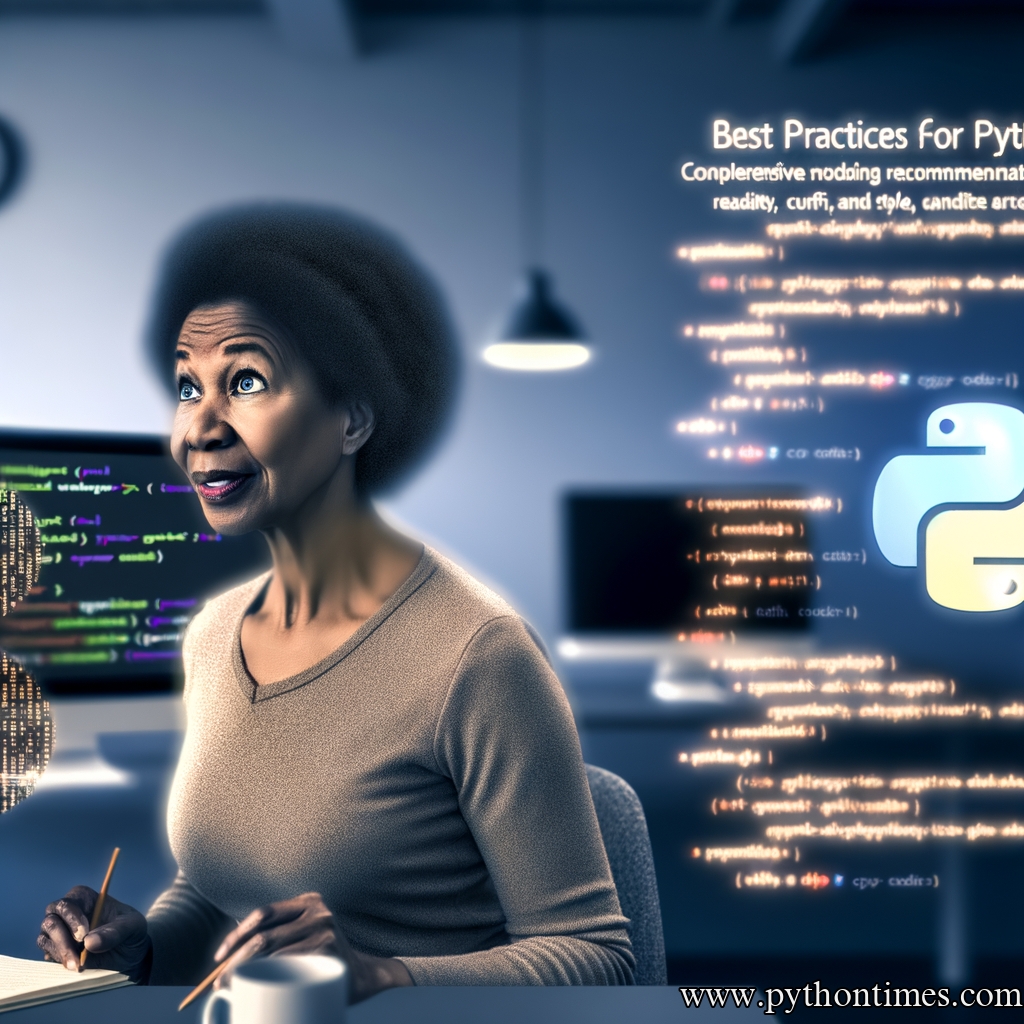
Table of Contents
- Follow PEP 8
- Writing Readable Code
- Comments
- Error Handling
- Avoid Premature Optimization
- Testing & Debugging
- Conclusion
1. Follow PEP 8
The Python Enhancement Proposal 8 (PEP 8) is the de-facto code style guide for Python. A violation of PEP 8 rules is considered a social faux pas in the Python community. Here are key highlights:
- Indentation: Use 4 spaces per indentation level.
def long_function_name(
var_one, var_two, var_three,
var_four):
pass
- Maximum Line Length: Limit all lines to a maximum of 79 characters for code and 72 for comments.
# Good
import os
# Bad
from os import path, walk, remove, rename, chmod, getcwd
- Blank Lines: Surround top-level functions and classes with two blank lines. Inside a class, surround methods with one blank line.
# Good
class MyClass:
def method_one(self):
pass
def method_two(self):
pass
# Bad
class MyClass:
def method_one(self):
pass
def method_two(self):
pass
2. Writing Readable Code
Writing clear and simple code acknowledges its potential readers. Here are some tips:
- Naming Conventions: Follow clear and descriptive naming. Functions should be lower case words separated by underscores. Class names should follow the CapWords convention.
# Good
class PythonGuide:
def print_hello(self):
print("Hello, Python!")
# Bad
class python_Guide:
def PrintHello(self):
print("Hello, Python!")
-
Code Simplification:
-
Use list comprehensions for creating lists succinctly.
# Good
squares = [x**2 for x in range(10)]
# Bad
squares = []
for x in range(10):
squares.append(x**2)
- Use built-in Python functions and libraries instead of re-inventing them.
# Good
import os
os.path.join('/hello/', 'world')
# Bad
'/hello/' + '/' + 'world'
3. Comments
Although Python’s syntax is quite clear, using comments aids in understanding why certain code exists. Remember, code explains the “how”, comments should explain the “why”.
- Comments: Should be complete sentences and use two spaces after a sentence-ending period.
# Good
x = x + 1 # Compensating for border
# Bad
x = x + 1 #Compensating for border
-
Inline Comments: Should be used sparingly and must be separated by at least two spaces from the statement.
-
Documentation Strings: For modules, classes, functions, and methods, documentation is written as a docstring at the beginning of the object.
def add_numbers(num1, num2):
"""
Function to add two numbers.
:param num1: The first number.
:param num2: The second number.
:return: The sum of the two numbers.
"""
return num1 + num2
4. Error Handling
Code that anticipates errors and exceptions is more robust. Python’s try and except blocks enable this.
# Good
try:
file = open('file.txt', 'rb')
except (IOError, EOFError) as e:
print("An error occurred. {}".format(e.args[-1]))
# Bad
file = open('file.txt', 'rb')
5. Avoid Premature Optimization
Donald Knuth famously said, “Premature optimization is the root of all evil”. Always prioritize readability and simplicity over minor performance gains.
# Good
items = ['<html>', '</html>']
item_string = "".join(items)
# Bad
items = ['<html>', '</html>']
item_string = ''
for item in items:
item_string += item
6. Testing & Debugging
Regularly test your code using Python’s unittest or third-party frameworks like pytest. This aids in catching bugs early and ensuring that your program works as expected.
import unittest
class Testing(unittest.TestCase):
def test_string(self):
self.assertEqual('hello'*3, 'hellohellohello')
if __name__ == '__main__':
unittest.main()
Python’s built-in pdb module is a powerful tool for debugging.
import pdb
def add_numbers(num1, num2):
# Start the debugger at the point of interest
pdb.set_trace()
return num1 + num2
7. Conclusion
Writing Pythonic code is both a science and an art. Beyond syntax, writing good Python code revolves around honoring readability and simplicity. Understanding these best practices helps code stand the test of time, comfortably accommodating new additions and changes.
Be it a hobbyist project or an enterprise solution, Python lets you focus on the fun part – solving problems, leaving the complexities of the language to these conventions and principals. As we come to end, remember the Zen of Python – Beautiful is better than ugly, Simple is better than complex, Readability counts. Happy Coding!