Unraveling Python’s Garbage Collection Mechanism: Memory Management Demystified
Sub: Understanding the Inner Workings of Python’s Memory Management
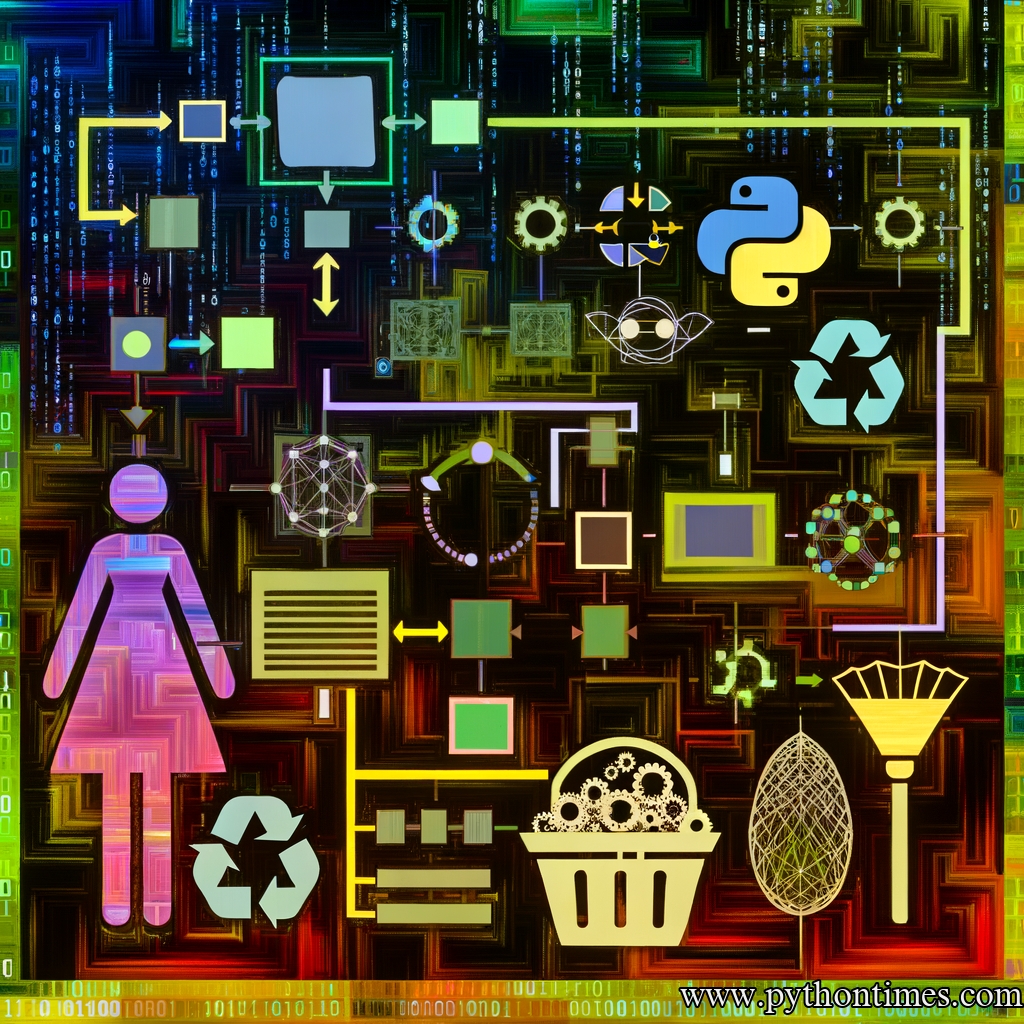
Python is a powerful and versatile programming language that is widely used across various domains, from web development to data science. One crucial aspect of Python that often goes unnoticed is its memory management. Under the hood, Python’s garbage collection mechanism efficiently manages memory, ensuring the smooth execution of programs and preventing memory leaks.
In this article, we will unravel the mystery behind Python’s garbage collection mechanism and explore how it handles memory management. Whether you are a beginner eager to grasp the fundamentals or a seasoned professional seeking a deeper understanding, this comprehensive guide will equip you with the knowledge you need to confidently navigate Python’s memory management landscape.
The Basics of Memory Management in Python
Before we dive into the intricacies of Python’s garbage collection mechanism, let’s establish a solid foundation by understanding the basics of memory management in Python.
1. Memory Allocation
When you run a Python program, memory is dynamically allocated to store objects and data. Python uses the built-in heap to manage this allocation efficiently. The heap is a region of memory where all Python objects are stored.
Unlike some low-level languages like C, Python abstracts away the complex details of memory management, allowing developers to focus on writing high-level code. However, to fully leverage the power of Python, it is essential to understand how memory is allocated and managed.
2. Reference Counting
At the core of Python’s memory management strategy lies reference counting. Each object in Python has a reference count, which is the number of references pointing to that object. For every reference created, the reference count of the object is incremented. When a reference goes out of scope or is explicitly deleted, the reference count is decremented.
When an object’s reference count reaches zero, it becomes eligible for garbage collection. Python’s garbage collector then reclaims the memory occupied by these objects and makes it available for future allocations. Reference counting is a lightweight and efficient method of memory management, but it has its limitations which we will explore later in this article.
A Deeper Dive into Python’s Garbage Collection Mechanism
Now that we have established the basics of Python’s memory management, let’s take a closer look at Python’s garbage collection mechanism.
The Generational Hypothesis
Python’s garbage collector is based on the generational hypothesis, which assumes that most objects have a short lifespan and become garbage quickly. This hypothesis aligns with the observation that many objects created during program execution are short-lived and are no longer needed once they go out of scope.
Based on the generational hypothesis, Python divides objects into different generations based on their age. The heap is divided into three generations: 0, 1, and 2. Objects in generation 0 are the youngest, while those in generation 2 are the oldest.
The garbage collector focuses its efforts on cleaning up objects in generation 0 and only promotes objects to the next generation when they survive a garbage collection cycle. This generational approach significantly improves the garbage collection process’s efficiency, as the majority of objects are short-lived and only a small portion survive to become long-lived objects.
The Mark and Sweep Algorithm
To effectively reclaim memory occupied by unreachable objects, Python’s garbage collector employs the mark and sweep algorithm. This algorithm consists of two phases: marking and sweeping.
1. Marking Phase
During the marking phase, the garbage collector traverses the object graph starting from the root objects, which are typically global variables and objects referenced from the call stack. It marks all objects that are reachable from the root objects as live. Objects that are not marked during this phase are considered unreachable and can be safely deallocated.
2. Sweeping Phase
Once the marking phase is complete, the sweeping phase begins. In this phase, the garbage collector iterates over all objects in the heap and frees memory occupied by objects that were not marked as live during the marking phase. This memory is then added to the free list and made available for future allocations.
The mark and sweep algorithm is an effective way of reclaiming memory occupied by unreachable objects. However, it has some drawbacks. One limitation is that it requires stopping the execution of the program momentarily during the sweeping phase, which can result in occasional pauses, impacting real-time systems or applications with strict performance requirements.
Python’s Reference Cycles and the Problem of Memory Leaks
While reference counting is efficient for managing memory in most scenarios, it falls short when dealing with reference cycles. A reference cycle occurs when a group of objects reference each other, forming a closed loop where none of the objects are reachable from the root objects.
In such cases, even if the reference count of each object in the cycle is zero, they are still not eligible for garbage collection because the reference count as a collective whole is not zero. This is where Python’s garbage collector comes to the rescue.
Python’s garbage collector also includes a cycle detector that can identify and break reference cycles. It does this by using a separate algorithm called graph traversal, which explores the object graph, looking for reference cycles. Once a reference cycle is detected, the garbage collector breaks the cycle by explicitly setting one or more references to None, making the objects eligible for garbage collection.
If you ever encounter memory leaks in your Python code, chances are it is due to reference cycles. Understanding how reference cycles work and leveraging Python’s garbage collector can help you successfully track down and resolve memory leaks.
Practical Examples and Best Practices
Let’s explore some practical examples and best practices to solidify our understanding of Python’s garbage collection mechanism and memory management.
1. Explicit Deletion
While Python’s garbage collector takes care of most memory management tasks automatically, there may be cases when you want to explicitly delete objects to free up memory. By using the del
keyword, you can explicitly delete variables and objects that are no longer needed.
data = [1, 2, 3, 4, 5]
del data
By explicitly deleting objects, you can immediately free up memory, especially in situations where you know specific objects will no longer be used in your program. However, in most cases, relying on Python’s garbage collector is sufficient, and explicit deletion is not necessary.
2. Weak References
Python provides a handy module called weakref
that can help mitigate the problem of reference cycles. Weak references allow you to reference an object without increasing its reference count. If an object referenced by a weak reference is no longer referenced elsewhere, it is eligible for garbage collection.
import weakref
data = [1, 2, 3, 4, 5]
weak_ref = weakref.ref(data)
del data
# Access the object through the weak reference
if weak_ref():
print("Object still exists")
else:
print("Object has been garbage collected")
Using weak references, you can break reference cycles and ensure that objects are properly garbage collected when they are no longer needed.
3. Effective Memory Management in Large-Scale Applications
In large-scale Python applications, effective memory management is crucial to ensure optimal performance and prevent memory bottlenecks. Here are some best practices to consider:
-
Minimize the use of global variables: Global variables have a longer lifespan than local variables and can lead to memory leaks if not managed correctly. Instead, prefer using local variables within functions or encapsulating data within classes.
-
Context managers and
with
statements: Context managers and thewith
statement ensure that resources are properly managed and cleaned up when they are no longer needed. This can help prevent memory leaks. -
Profiling and memory optimization tools: Python provides powerful profiling and memory optimization tools, such as
memory_profiler
andobjgraph
, which can help identify memory-intensive parts of your code and optimize memory usage efficiently. -
Efficient data structures: Choosing the right data structures can significantly impact memory usage. For example, using generators or iterators instead of lists can save memory when dealing with large datasets.
By following these best practices and leveraging the features and tools provided by Python, you can effectively manage memory in large-scale applications and ensure optimal performance.
Conclusion
Python’s garbage collection mechanism is a vital component of the language’s memory management. Understanding how Python handles memory allocation, reference counting, and garbage collection is essential for writing efficient and robust Python code.
In this article, we explored the basics of memory management in Python, the generational hypothesis, the mark and sweep algorithm, and how Python’s garbage collector handles reference cycles. We also discussed practical examples and best practices for effective memory management.
By grasping these concepts and applying best practices, you can confidently leverage Python’s garbage collection mechanism to prevent memory leaks, optimize memory usage, and write high-performing Python code.
Remember, Python’s memory management works silently in the background, making Python an accessible and user-friendly language. Embrace its power, and let Python simplify the complexities of memory management in your programs.