Tips for Writing Pythonic Code
Python, the “duck-typed” language prides itself on its readability and simplicity. Yet, for programmers new to Python, understanding what constitutes “Pythonic” code can often feel like learning a second language.
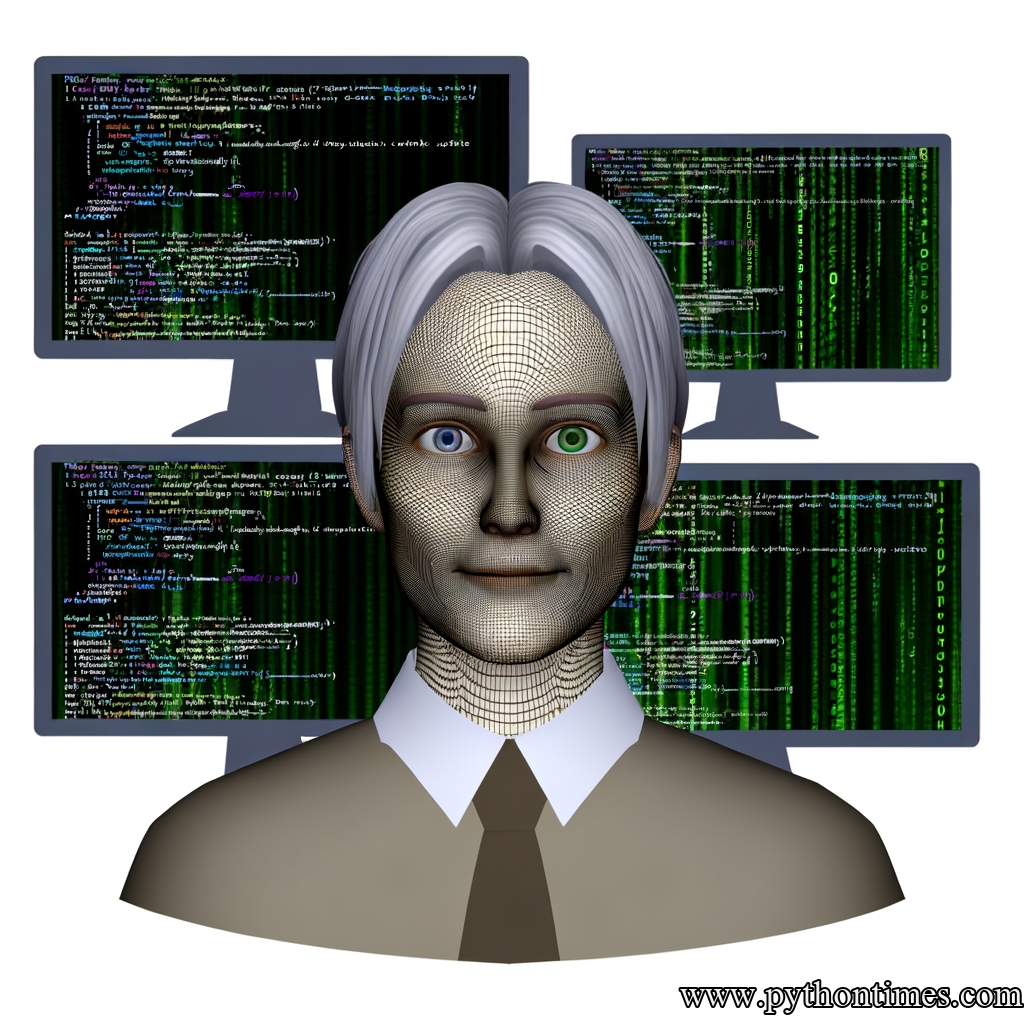
The Zen of Python, a collection of 20 software principles, guides Python’s design. Mentioned principles can provide valuable pointers to write more Pythonic code. This article aims to dissect Python’s saying – “There’s only one way to do it” – and provides you with practical tips to writing Pythonic code that both beginner and experienced Python enthusiasts will find worthy.
The Zen of Python
Before we dive into the tips, let’s take a moment to reflect upon the Zen of Python, often referred to as PEP 20 (Python Enhancement Proposal). These guiding principles, when pieced together, forms a puzzle that is the philosophy behind Python’s design.
import this
Running the above script will reveal the Zen of Python in your console.
Top principles from the Zen of Python that offer insight into what constitutes Pythonic code includes:
- Beautiful is better than ugly.
- Explicit is better than implicit.
- Simple is better than complex.
- Readability counts.
Now let’s delve into the actual tips that will help you write more Pythonic code.
1. Use Underscores for Variable Names
Python recommends Non-Camel Casing. Python developers should use underscores in their variable names. This is a small yet significant shift for developers coming from languages like Java or C++.
#Pythonic way
employee_name = "John Doe"
#Non-Pythonic way
employeeName = "John Doe"
2. Use List Comprehensions
List comprehensions provide a concise way to create lists based on existing lists. When applicable, list comprehensions can make your code more readable and Pythonic.
#Pythonic way
squares = [x**2 for x in range(10)]
#Non-Pythonic way
squares = []
for x in range(10):
squares.append(x**2)
3. Use is not
instead of !=
The is not
operator is more readable and Pythonic than !=
.
#Pythonic way
if x is not None:
#Non-Pythonic way
if x != None:
4. Use in
to Check Membership
Python provides a simple and clean way to check for membership in a sequence like a string, list, or tuple using in
.
#Pythonic way
if x in some_list:
#Non-Pythonic way
found = False
for item in some_list:
if item == x:
found = True
break
5. Keep Your Code Simple and Avoid Unnecessary Constructs
Earlier, we mentioned that “simple is better than complex”. This is one of the guiding principles of Python. Always strive to keep your code simple and clean. If there’s a simpler way to achieve the same result, never opt for a more complex solution.
#Pythonic way
custome_input = input("Enter something: ")
#Non-Pythonic way
try:
custom_input = raw_input("Enter something: ")
except NameError:
custom_input = input("Enter something: ")
6. Use Format Strings
While there are several methods to perform string formatting in Python, f-strings are the most Pythonic and should be your go-to method for string formatting.
#Pythonic way
name = "John"
print(f"Hello, {name}!")
#Non-Pythonic way
name = "John"
print("Hello, %s!" % name)
7. Use Context Managers for File Operations
Python includes context managers that manage the opening and closing of resources, reducing the risk of leaks. Always use context managers when dealing with file operations or other similar tasks.
#Pythonic way
with open('file.txt', 'w') as f:
f.write('Hello, world!')
#Non-Pythonic way
file = open('file.txt', 'w')
try:
file.write('Hello, world!')
finally:
file.close()
8. Use Generators for Large Set of Results
Generators are a smart way of managing large sequences of data without the risk of memory overflow. Whenever you’re dealing with a large set of results, using generators can make your code more efficient and Pythonic.
#Pythonic way
def large_set(n):
num = 0
while num < n:
yield num
num += 1
#Non-Pythonic way
def large_set(n):
num = 0
result = []
while num < n:
result.append(num)
num += 1
return result
These are not all the Pythonic ways to write Python code, but incorporating these tips into your Python programming can significantly improve your code’s efficiency, readability, and elegance. Importantly, Python’s core philosophy revolves around simplicity, beauty, and readability. Always strive to write code that aligns with this philosophy (or Zen), and you’re on your way to becoming a truly Pythonic programmer. Happy coding!